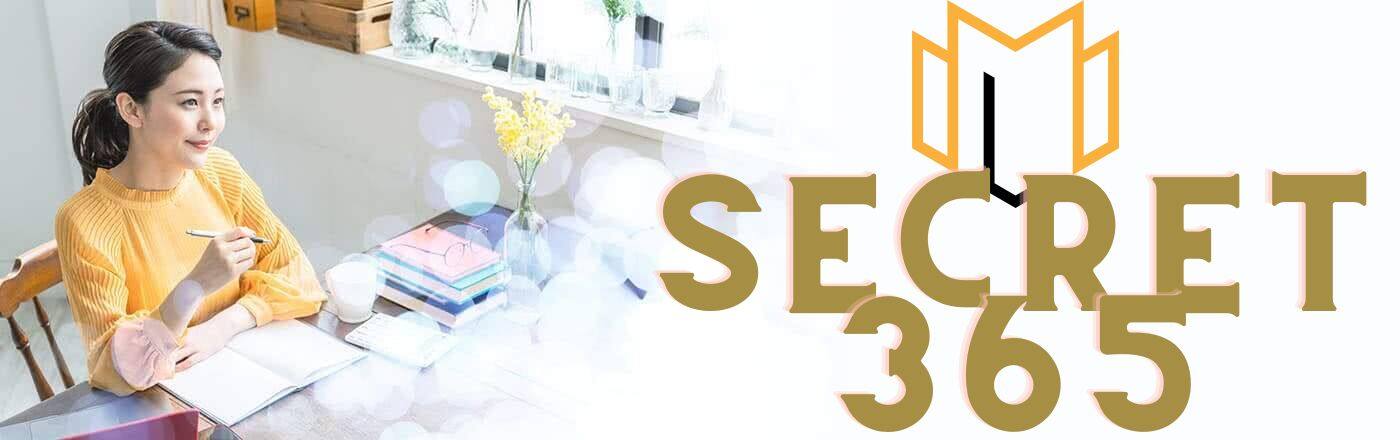
"Structuring a React App: Implementing Best Practices for Seamless Development"
Structuring a React app is crucial for maintaining code organization, scalability, and reusability. By implementing best practices, developers can ensure that their React app is well-organized, easy to understand, and maintainable. In this article, we will explore various techniques and guidelines for structuring a React app, including component organization, folder structure, state management, and code separation. By following these best practices, developers can create robust and efficient React apps that are easier to develop, test, and maintain.
Structuring a React App: Implementing Best Practices
Component Organization and Folder Structure
When it comes to building a React app, one of the key aspects to consider is how to organize your components and folder structure. A well-structured app not only improves code readability but also enhances maintainability and scalability. In this article, we will explore some best practices for component organization and folder structure in a React app.
First and foremost, it is important to have a clear understanding of the different types of components in React. There are two main types: functional components and class components. Functional components are stateless and are typically used for rendering UI elements. On the other hand, class components have state and lifecycle methods, making them suitable for more complex logic and interactivity. Keeping this distinction in mind, let's dive into the best practices for organizing your components.
One widely adopted approach is to group components based on their functionality or purpose. This can be achieved by creating separate folders for each feature or section of your app. For example, if you have a blog app, you might have folders for components related to user authentication, blog posts, comments, and so on. This way, it becomes easier to locate and manage components specific to a particular feature.
Within each feature folder, it is recommended to further organize components into subfolders based on their type. For instance, you can have separate folders for functional components and class components. This helps maintain a clear separation between the two types and makes it easier to navigate through the codebase.
Another important aspect to consider is the naming convention for your components. It is advisable to use descriptive and meaningful names that accurately reflect the purpose of the component. This not only improves code readability but also makes it easier for other developers to understand the functionality of each component. Additionally, it is a good practice to use PascalCase for component names, as it aligns with the standard naming convention in React.
In addition to organizing components, it is equally important to structure your folders in a logical manner. One common approach is to have a separate folder for each major section of your app, such as "components," "containers," "services," and "utils." The "components" folder can contain all the reusable UI components, while the "containers" folder can house components that connect to the Redux store or manage state. The "services" folder can contain any external API calls or data fetching logic, while the "utils" folder can store utility functions or helper classes.
Furthermore, it is recommended to keep your folder structure flat and avoid excessive nesting. This ensures that components and files are easily accessible and reduces the complexity of navigating through the codebase. However, if you have a large app with multiple subfeatures, it might be necessary to introduce some level of nesting to maintain a clear hierarchy.
In conclusion, organizing your components and folder structure is crucial for building a well-structured and maintainable React app. By grouping components based on functionality, using descriptive names, and following a logical folder structure, you can improve code readability, scalability, and collaboration among developers. Remember to keep your folder structure flat and avoid excessive nesting to ensure easy navigation. By implementing these best practices, you can set a solid foundation for your React app and make it easier to maintain and scale in the long run.
State Management and Data Flow
In a React app, managing state and controlling the flow of data is crucial for creating a smooth and efficient user experience. By implementing best practices for state management and data flow, developers can ensure that their app is scalable, maintainable, and easy to debug.
One of the most popular approaches to state management in React is using a library called Redux. Redux provides a centralized store for managing the state of an application, making it easier to track changes and update components accordingly. By following the principles of Redux, developers can create a predictable and reliable data flow within their app.
To implement Redux in a React app, developers need to define actions, reducers, and a store. Actions are plain JavaScript objects that describe the changes that need to be made to the state. Reducers are pure functions that take the current state and an action as input and return a new state. The store is responsible for holding the state and dispatching actions to the reducers.
By separating the concerns of state management from the components themselves, Redux allows for a more modular and reusable codebase. Components can simply subscribe to the store and receive updates whenever the state changes, without having to worry about how the state is managed or where it comes from.
Another important aspect of state management is handling asynchronous actions. In a real-world app, data fetching and API calls are common tasks that need to be handled asynchronously. Redux provides middleware, such as Redux Thunk or Redux Saga, to handle these asynchronous actions.
With Redux Thunk, developers can dispatch functions instead of plain objects as actions. These functions can perform asynchronous operations, such as fetching data from an API, and dispatch additional actions once the operation is complete. This allows for more complex data flows and enables developers to handle asynchronous actions in a more controlled and organized manner.
Redux Saga, on the other hand, uses generator functions to handle asynchronous actions. These generator functions can pause and resume execution, making it easier to handle complex asynchronous flows, such as handling multiple API calls in parallel or sequentially. Redux Saga provides a powerful and flexible solution for managing asynchronous actions in a React app.
In addition to Redux, React also provides its own built-in state management solution called React Context. React Context allows developers to share state between components without the need for prop drilling. It provides a way to pass data through the component tree without explicitly passing props at every level.
While React Context can be a convenient solution for small to medium-sized apps, it may not be suitable for larger and more complex applications. Redux, with its centralized store and predictable data flow, is often a better choice for managing state in larger apps with multiple components and complex data dependencies.
In conclusion, state management and data flow are essential aspects of building a React app. By implementing best practices, such as using Redux for state management and handling asynchronous actions with middleware like Redux Thunk or Redux Saga, developers can create scalable, maintainable, and efficient apps. React Context can also be a useful tool for smaller apps, but for larger and more complex applications, Redux provides a more robust solution. By following these best practices, developers can ensure that their React apps are well-structured and adhere to industry standards.
Performance Optimization and Code Splitting
When it comes to building a React app, performance optimization is a crucial aspect that developers need to consider. A well-optimized app not only provides a better user experience but also ensures that the app runs smoothly and efficiently. In this section, we will explore some best practices for performance optimization and code splitting in a React app.
One of the first steps in optimizing a React app is to identify and eliminate any unnecessary re-renders. React's virtual DOM efficiently updates only the components that have changed, but sometimes, unnecessary re-renders can occur due to improper usage of lifecycle methods or inefficient state management. By using shouldComponentUpdate or React.memo, developers can prevent unnecessary re-renders and improve the overall performance of the app.
Another important aspect of performance optimization is lazy loading or code splitting. Code splitting allows developers to split their app's code into smaller chunks and load them on-demand, rather than loading the entire app at once. This can significantly improve the initial load time of the app, especially for larger applications. React provides a built-in mechanism called React.lazy, which allows developers to easily implement code splitting in their app.
To implement code splitting using React.lazy, developers need to wrap the components that they want to lazy load with the lazy function. This function takes a dynamic import statement as its argument, which specifies the module to be loaded when the component is rendered. The lazy-loaded component is then rendered using the Suspense component, which displays a fallback UI while the component is being loaded.
In addition to lazy loading components, developers can also split their app's code based on routes. This means that each route in the app can have its own separate bundle, which is loaded only when that specific route is accessed. This can be achieved using libraries like React Router, which provides a Route-based code splitting feature. By splitting the code based on routes, developers can further optimize the app's performance by reducing the initial load time and improving the overall user experience.
Apart from lazy loading and route-based code splitting, there are other techniques that developers can use to optimize the performance of their React app. One such technique is tree shaking, which is a process of eliminating unused code from the app's bundle. By removing unused code, the app's bundle size can be significantly reduced, resulting in faster load times and improved performance.
Another technique is to use performance monitoring tools like React Profiler or Chrome DevTools to identify any performance bottlenecks in the app. These tools provide valuable insights into the app's rendering performance, component lifecycles, and overall performance metrics. By analyzing these metrics, developers can pinpoint areas that need optimization and make the necessary improvements.
In conclusion, performance optimization and code splitting are essential aspects of building a well-optimized React app. By implementing best practices like lazy loading, route-based code splitting, tree shaking, and using performance monitoring tools, developers can significantly improve the app's performance and provide a better user experience. It is important for developers to continuously monitor and optimize their app's performance to ensure that it runs smoothly and efficiently.
1. What are some best practices for structuring a React app?
- Use a component-based architecture
- Separate concerns by organizing components into folders
- Follow a consistent naming convention for files and components
- Utilize container and presentational components for better separation of concerns
- Keep components small and focused on a single responsibility
- Use a state management library like Redux for managing complex application state
2. How can I organize my React components into folders?
- Group related components together in folders based on their functionality or feature
- Use a common folder structure like the "src" folder for all components
- Create subfolders within the "src" folder for different sections or modules of your app
- Use index.js files within folders to export components for easier importing
3. What is the benefit of using container and presentational components?
- Container components handle the logic and state management of a component, while presentational components focus on rendering the UI
- This separation of concerns makes components easier to understand, test, and maintain
- Container components can be reused across different presentational components, promoting code reusability
- Presentational components can be easily styled and customized without affecting the logic in container components
In conclusion, structuring a React app by implementing best practices is crucial for maintaining a clean and scalable codebase. By following guidelines such as separating concerns, organizing components, using container and presentational components, and utilizing state management libraries, developers can enhance the maintainability and reusability of their React applications. Additionally, adopting a modular approach, optimizing performance, and adhering to coding conventions contribute to a more efficient development process and improved user experience. Overall, implementing best practices in structuring a React app is essential for creating high-quality and maintainable applications.