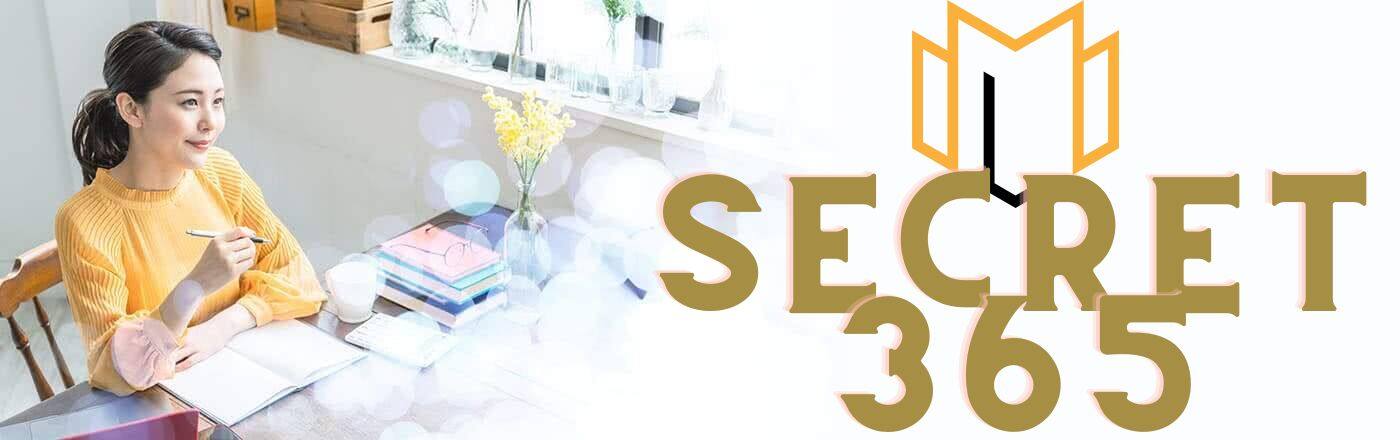
"Unlock the Power of Arrays: A Comprehensive Guide to Data Structures"
An Introduction to Arrays in Data Structures:
Arrays are one of the fundamental data structures used in computer programming. They provide a way to store and organize a collection of elements of the same type. An array is a contiguous block of memory that can hold a fixed number of elements, each identified by its index. This index allows for efficient access and manipulation of the elements within the array. Arrays are widely used in various algorithms and applications, making them an essential concept to understand in data structures.
Arrays are a fundamental data structure used in computer programming and data analysis. They provide a way to store and organize a collection of elements of the same type. Arrays offer several benefits that make them a popular choice for managing data in various applications.
One of the key advantages of using arrays is their ability to efficiently store and access elements. Arrays allocate a contiguous block of memory to store the elements, which allows for constant-time access to any element using its index. This means that accessing an element in an array takes the same amount of time, regardless of the size of the array. This property makes arrays ideal for scenarios where fast and direct access to elements is required.
Another benefit of arrays is their simplicity and ease of use. Arrays have a straightforward syntax and are supported by most programming languages. They can be easily declared and initialized, and elements can be added, modified, or removed using simple operations. This simplicity makes arrays a versatile tool that can be used in a wide range of applications, from simple data storage to complex algorithms.
Arrays also offer efficient memory utilization. Since arrays allocate a fixed amount of memory for a specific number of elements, they can be more memory-efficient compared to other data structures. This is particularly useful when dealing with large datasets or limited memory resources. Additionally, arrays can be dynamically resized to accommodate changing data requirements, allowing for efficient memory management.
Arrays are also highly compatible with other data structures and algorithms. Many algorithms and data manipulation operations are designed to work with arrays, making them a natural choice for implementing various algorithms and data processing tasks. Arrays can be easily sorted, searched, and manipulated using a wide range of algorithms, making them a versatile tool for data analysis and manipulation.
Furthermore, arrays offer efficient memory caching. When elements are stored in a contiguous block of memory, they are more likely to be stored in the CPU cache, which is faster to access compared to main memory. This can significantly improve the performance of operations that involve accessing array elements, such as iterating over the elements or performing calculations on them.
In conclusion, arrays are a powerful and versatile data structure that offers several benefits in terms of efficient storage, fast access, simplicity, memory utilization, compatibility with algorithms, and memory caching. They are widely used in various applications, ranging from simple data storage to complex data analysis and manipulation. Understanding the benefits of arrays can help programmers and data analysts make informed decisions when choosing the appropriate data structure for their specific needs.
Arrays are a fundamental data structure used in computer programming to store and manipulate collections of elements. They provide a way to organize and access data efficiently, making them an essential tool for developers. In this article, we will explore some common operations and manipulations that can be performed with arrays.
One of the most basic operations with arrays is accessing individual elements. Each element in an array is assigned a unique index, starting from zero. This index allows us to retrieve or modify the value stored at a specific position in the array. For example, if we have an array of integers called "numbers," we can access the third element by using the index 2: numbers[2]. This simple operation allows us to retrieve or update data quickly.
Another common operation is adding elements to an array. Arrays have a fixed size, meaning that they can only hold a certain number of elements. However, we can dynamically resize an array by creating a new, larger array and copying the existing elements into it. This process is known as resizing an array. It involves allocating a new block of memory, copying the existing elements, and updating the array's reference to the new memory location. By resizing an array, we can add new elements and increase its capacity as needed.
Similarly, we can remove elements from an array. When an element is removed, the remaining elements need to be shifted to fill the gap. This operation can be time-consuming, especially if the array is large or if the element being removed is at the beginning or middle of the array. To optimize this process, some programming languages provide specialized data structures, such as lists or linked lists, that handle element removal more efficiently. However, arrays remain a useful tool for many scenarios where element removal is not a frequent operation.
Sorting is another important manipulation that can be performed with arrays. Sorting an array arranges its elements in a specific order, such as ascending or descending. There are various sorting algorithms available, each with its own advantages and disadvantages in terms of time complexity and space complexity. Some common sorting algorithms include bubble sort, insertion sort, selection sort, merge sort, and quicksort. These algorithms can be implemented to sort arrays of different data types, such as integers, strings, or objects, based on specific criteria.
Searching for an element in an array is also a common operation. There are different search algorithms available, each with its own trade-offs in terms of time complexity and space complexity. Linear search is a simple algorithm that checks each element in the array sequentially until a match is found or the end of the array is reached. Binary search, on the other hand, is a more efficient algorithm that requires the array to be sorted. It repeatedly divides the array in half and compares the target element with the middle element until a match is found or the search space is exhausted.
In conclusion, arrays are a versatile data structure that allows for various operations and manipulations. They provide efficient access to individual elements, support dynamic resizing, and can be sorted or searched efficiently. While arrays have some limitations, such as fixed size and expensive element removal, they remain a fundamental tool in computer programming. Understanding how to perform common operations and manipulations with arrays is essential for any developer working with data structures.
An Introduction to Arrays in Data Structures
Understanding Array Algorithms and their Applications
Arrays are a fundamental concept in data structures, and they play a crucial role in various algorithms. In this article, we will explore the basics of arrays, their properties, and their applications in different scenarios.
To begin with, an array is a collection of elements of the same data type, stored in contiguous memory locations. Each element in the array can be accessed using its index, which represents its position within the array. The index starts from 0 for the first element and increments by 1 for each subsequent element.
One of the key advantages of arrays is their ability to store multiple elements of the same type efficiently. By allocating a fixed amount of memory, arrays provide constant-time access to any element. This makes them ideal for scenarios where quick and direct access to elements is required.
Arrays can be used to solve a wide range of problems. For example, they are commonly used to store and manipulate collections of data, such as lists of numbers, strings, or objects. Additionally, arrays can be used to represent matrices, graphs, and other complex data structures.
When working with arrays, it is important to understand various algorithms that can be applied to them. One such algorithm is searching, which involves finding a specific element within an array. The most basic search algorithm is linear search, where each element is checked sequentially until a match is found. Another commonly used search algorithm is binary search, which is applicable only to sorted arrays and has a logarithmic time complexity.
Sorting is another important algorithmic operation on arrays. Sorting involves arranging the elements of an array in a specific order, such as ascending or descending. There are numerous sorting algorithms available, each with its own advantages and disadvantages. Some popular sorting algorithms include bubble sort, insertion sort, selection sort, merge sort, and quicksort.
Arrays can also be used to implement other data structures, such as stacks and queues. A stack is a data structure that follows the Last-In-First-Out (LIFO) principle, where the last element added is the first one to be removed. This behavior can be easily achieved using an array by keeping track of the top element and updating its index accordingly.
Similarly, a queue is a data structure that follows the First-In-First-Out (FIFO) principle, where the first element added is the first one to be removed. An array can be used to implement a queue by maintaining two indices, one for the front and one for the rear. Elements are added at the rear and removed from the front, ensuring the correct order of operations.
In conclusion, arrays are a fundamental concept in data structures, offering efficient storage and access to multiple elements of the same type. They are widely used in various algorithms and have applications in different scenarios. Understanding array algorithms, such as searching, sorting, and implementing other data structures, is essential for efficient problem-solving. By mastering arrays and their algorithms, programmers can optimize their code and improve the performance of their applications.
1. What is an array in data structures?
An array is a data structure that stores a fixed-size sequence of elements of the same type.
2. What are the advantages of using arrays?
Arrays provide fast and direct access to elements using their index. They also allow efficient memory allocation and deallocation.
3. What are the limitations of arrays?
Arrays have a fixed size, which means they cannot easily be resized once created. Additionally, inserting or deleting elements in the middle of an array can be inefficient as it requires shifting all subsequent elements.
In conclusion, arrays are an essential data structure in computer science and programming. They provide a way to store and access multiple elements of the same data type efficiently. Arrays offer constant time access to elements, making them suitable for various applications. Understanding the basics of arrays, such as their declaration, initialization, and manipulation, is crucial for any programmer. Additionally, arrays can be multidimensional, allowing for the representation of more complex data structures. Overall, arrays are a fundamental concept that every programmer should be familiar with in order to effectively solve problems and develop efficient algorithms.