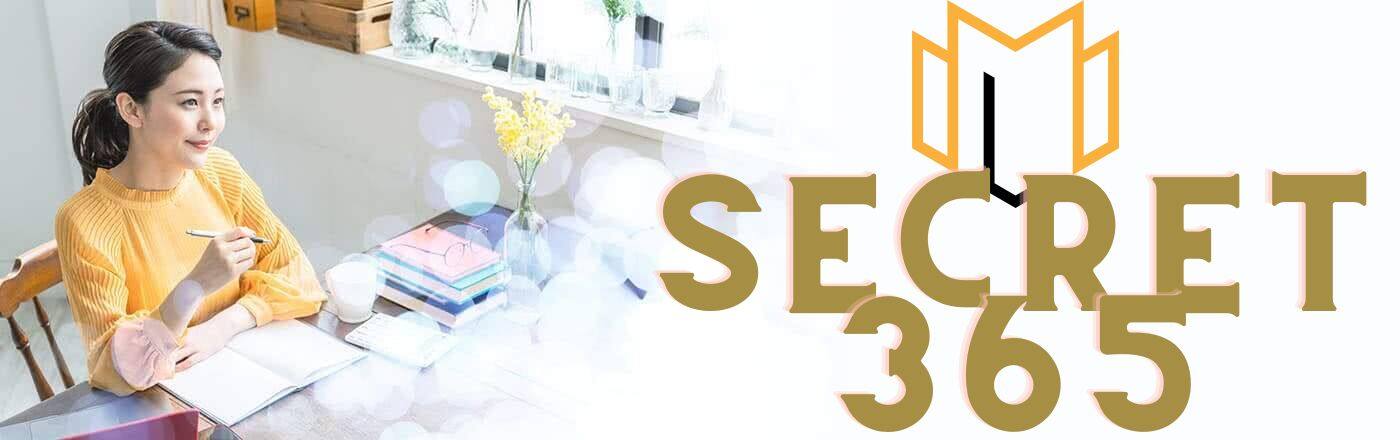
Unleash your coding potential with Essential Concepts for Every Developer.
Introduction: Essential Concepts for Every Developer
As a developer, there are certain fundamental concepts that are crucial to understand and apply in order to excel in the field. These concepts serve as the building blocks for creating efficient, scalable, and maintainable software solutions. Whether you are a beginner or an experienced developer, having a strong grasp of these essential concepts is essential for success. In this article, we will explore some of the key concepts that every developer should be familiar with, including data structures, algorithms, object-oriented programming, version control, and software testing. By mastering these concepts, developers can enhance their problem-solving skills, write clean and robust code, collaborate effectively with other developers, and deliver high-quality software products.
Object-Oriented Programming: A Foundation for Developers
In the world of software development, object-oriented programming (OOP) is a fundamental concept that every developer should understand. OOP is a programming paradigm that revolves around the idea of creating objects, which are instances of classes, and using them to build complex and modular software systems. By understanding the essential concepts of OOP, developers can write more efficient, maintainable, and scalable code.
One of the key concepts in OOP is encapsulation. Encapsulation refers to the bundling of data and methods into a single unit, known as a class. This allows developers to hide the internal details of an object and only expose the necessary information and functionality. By encapsulating data and methods, developers can ensure that the object's state remains consistent and that changes to the internal implementation do not affect other parts of the codebase.
Another important concept in OOP is inheritance. Inheritance allows developers to create new classes based on existing ones, inheriting their properties and methods. This promotes code reuse and allows for the creation of more specialized classes that inherit the common behavior from a base class. Inheritance also enables developers to create hierarchies of classes, where each class inherits from a parent class, forming a tree-like structure. This hierarchical structure makes it easier to organize and manage complex software systems.
Polymorphism is yet another crucial concept in OOP. Polymorphism allows objects of different classes to be treated as objects of a common superclass. This means that a method can be defined in a superclass and implemented differently in each subclass. This flexibility allows developers to write code that can work with objects of different types, without needing to know the specific type at compile-time. Polymorphism promotes code extensibility and flexibility, making it easier to add new functionality to existing code without modifying the existing implementation.
Abstraction is a concept closely related to encapsulation. Abstraction refers to the process of simplifying complex systems by breaking them down into smaller, more manageable parts. In OOP, abstraction is achieved through the use of abstract classes and interfaces. Abstract classes provide a blueprint for creating subclasses, while interfaces define a contract that classes must adhere to. By using abstraction, developers can focus on the essential features of an object or system, without getting bogged down in the implementation details.
Lastly, OOP emphasizes the concept of modularity. Modularity refers to the practice of breaking down a software system into smaller, independent modules that can be developed and tested separately. Each module encapsulates a specific functionality and can be easily integrated with other modules to form a complete system. This modular approach promotes code reusability, maintainability, and scalability. Developers can work on different modules concurrently, reducing development time and improving overall productivity.
In conclusion, object-oriented programming is a foundational concept that every developer should understand. By grasping the essential concepts of OOP, developers can write more efficient, maintainable, and scalable code. Encapsulation, inheritance, polymorphism, abstraction, and modularity are all key concepts that form the building blocks of OOP. By applying these concepts, developers can create software systems that are easier to understand, extend, and maintain. So, whether you are a beginner or an experienced developer, taking the time to master OOP will undoubtedly enhance your programming skills and make you a more effective developer.
Understanding Data Structures and Algorithms for Efficient Coding
In the world of software development, efficiency is key. Developers strive to create programs that are not only functional but also performant. To achieve this, it is crucial to have a solid understanding of data structures and algorithms. These concepts form the foundation of efficient coding and can greatly impact the performance of a program.
Data structures are the building blocks of any program. They provide a way to organize and store data in a way that allows for efficient retrieval and manipulation. There are various types of data structures, each with its own strengths and weaknesses. Some common examples include arrays, linked lists, stacks, queues, and trees.
Arrays are a simple and straightforward data structure that stores elements in contiguous memory locations. They offer constant-time access to elements, making them ideal for scenarios where random access is required. However, their size is fixed, and inserting or deleting elements can be costly.
Linked lists, on the other hand, provide dynamic memory allocation and flexibility. They consist of nodes that contain both data and a reference to the next node. This allows for efficient insertion and deletion operations, but accessing elements requires traversing the list, resulting in linear time complexity.
Stacks and queues are abstract data types that follow a specific order of operations. Stacks use a Last-In-First-Out (LIFO) approach, while queues use a First-In-First-Out (FIFO) approach. Stacks are commonly used for tasks such as function calls and undo operations, while queues are useful for managing tasks in a sequential manner.
Trees are hierarchical data structures that consist of nodes connected by edges. They are widely used for organizing and searching data efficiently. Binary trees, in particular, are commonly used due to their simplicity and ease of implementation. They offer efficient search, insertion, and deletion operations, with a time complexity of O(log n) in balanced trees.
While data structures provide a way to store and organize data, algorithms determine how that data is processed. An algorithm is a step-by-step procedure for solving a problem or performing a specific task. It takes input, performs a series of operations, and produces an output.
Efficient algorithms can greatly improve the performance of a program. They can reduce the time and space complexity, resulting in faster execution and reduced resource usage. Some common algorithms include sorting, searching, and graph traversal algorithms.
Sorting algorithms arrange elements in a specific order, such as ascending or descending. There are various sorting algorithms available, each with its own advantages and disadvantages. Some popular ones include bubble sort, insertion sort, selection sort, merge sort, and quicksort.
Searching algorithms, on the other hand, are used to find a specific element within a collection of data. Linear search and binary search are two commonly used searching algorithms. Linear search checks each element in sequence until a match is found, while binary search divides the search space in half at each step, resulting in a faster search for sorted data.
Graph traversal algorithms are used to explore or visit all the nodes in a graph. Depth-First Search (DFS) and Breadth-First Search (BFS) are two widely used graph traversal algorithms. DFS explores as far as possible along each branch before backtracking, while BFS explores all the vertices of a particular depth before moving on to the next level.
In conclusion, understanding data structures and algorithms is essential for every developer. These concepts form the backbone of efficient coding and can greatly impact the performance of a program. By choosing the right data structure and implementing efficient algorithms, developers can create programs that are not only functional but also performant.
Best Practices for Version Control and Collaboration in Software Development
In the world of software development, version control and collaboration are essential concepts that every developer should be familiar with. These practices ensure that multiple developers can work on a project simultaneously, while also keeping track of changes and maintaining a history of the codebase. In this article, we will explore some best practices for version control and collaboration that can help developers streamline their workflow and improve productivity.
One of the most widely used version control systems is Git. Git allows developers to create branches, which are independent lines of development that can be worked on separately. This enables multiple developers to work on different features or bug fixes simultaneously, without interfering with each other's work. It is important to create a new branch for each new feature or bug fix, as this helps keep the codebase organized and makes it easier to track changes.
When working on a branch, it is crucial to commit changes frequently. Commits serve as checkpoints in the development process, allowing developers to easily revert to a previous state if needed. It is recommended to make small, atomic commits that focus on a specific task or change. This makes it easier to understand the purpose of each commit and helps in reviewing code changes.
Another important aspect of version control is merging branches. Once a feature or bug fix is complete, it needs to be merged back into the main branch, often referred to as the "master" branch. Before merging, it is essential to ensure that the code is thoroughly tested and does not introduce any new bugs. This can be achieved through automated testing and code reviews.
Code reviews are an integral part of the collaboration process in software development. They involve having another developer review the code changes before they are merged into the main branch. Code reviews help identify potential issues, improve code quality, and ensure that the codebase adheres to best practices and coding standards. It is important to provide constructive feedback during code reviews and address any concerns or suggestions raised by the reviewer.
In addition to code reviews, effective communication among team members is crucial for successful collaboration. Developers should regularly communicate with each other to discuss progress, clarify requirements, and resolve any conflicts or issues that may arise. This can be done through team meetings, instant messaging platforms, or project management tools.
Furthermore, it is important to document the codebase and keep it up to date. This includes writing clear and concise comments within the code, as well as maintaining a comprehensive README file that provides an overview of the project, installation instructions, and any other relevant information. Documentation helps new developers understand the codebase quickly and facilitates collaboration among team members.
Lastly, it is essential to use a centralized repository to store the codebase and track changes. This allows developers to easily access the latest version of the code, collaborate effectively, and maintain a history of the project. Popular platforms for hosting repositories include GitHub, GitLab, and Bitbucket.
In conclusion, version control and collaboration are fundamental practices in software development. By following best practices such as creating branches, committing frequently, merging carefully, conducting code reviews, communicating effectively, documenting the codebase, and using a centralized repository, developers can streamline their workflow, improve productivity, and ensure the success of their projects.
1. What are essential concepts for every developer?
- Object-oriented programming
- Data structures and algorithms
- Version control systems
2. Why is object-oriented programming important for developers?
- It allows for modular and reusable code
- Encourages code organization and maintainability
- Supports code extensibility and scalability
3. What are some commonly used data structures and algorithms?
- Arrays, linked lists, stacks, and queues
- Binary search, sorting algorithms (e.g., bubble sort, merge sort), and graph algorithms (e.g., breadth-first search, depth-first search)
In conclusion, understanding essential concepts is crucial for every developer. These concepts include data structures, algorithms, programming paradigms, and software design principles. By having a strong grasp of these concepts, developers can write efficient and maintainable code, solve complex problems, and create high-quality software applications. Continuous learning and practice in these areas are essential for developers to stay updated and excel in their careers.