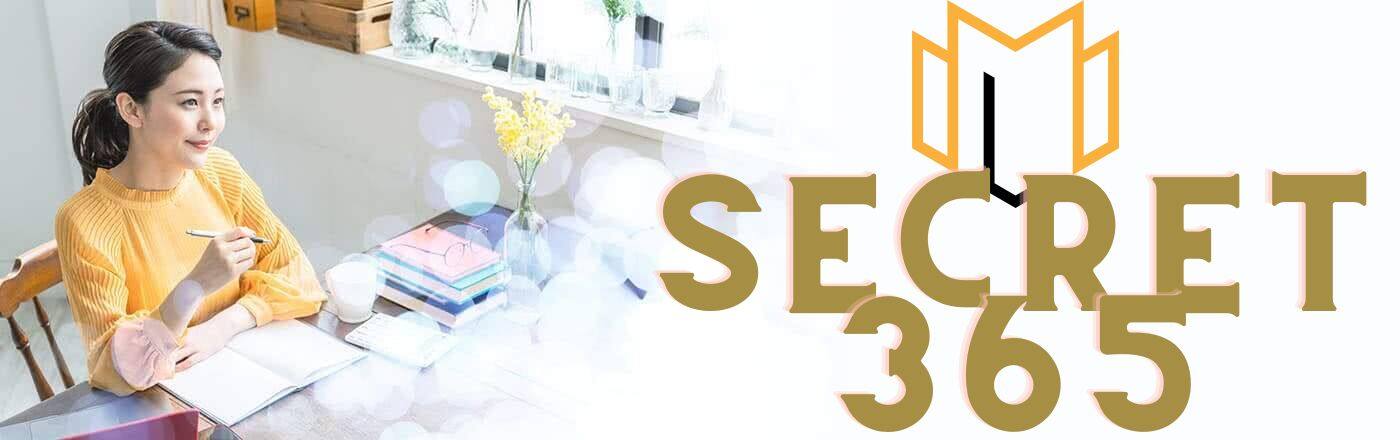
Master the art of SQL data cleaning with code examples.
Introduction:
A Comprehensive Guide to SQL Data Cleaning: Including Code Examples is a comprehensive resource that aims to provide a step-by-step approach to cleaning and preparing data using SQL. This guide is designed for both beginners and experienced SQL users who want to enhance their data cleaning skills and ensure the accuracy and reliability of their datasets.
With the increasing importance of data-driven decision making, it is crucial to have clean and well-structured data. However, data cleaning can be a complex and time-consuming task. This guide simplifies the process by breaking it down into manageable steps and providing code examples that can be easily implemented.
Throughout the guide, you will learn various techniques and best practices for identifying and handling common data quality issues such as missing values, duplicates, inconsistencies, and outliers. You will also explore advanced topics like data normalization, data validation, and data transformation.
The guide covers a wide range of SQL functions, operators, and clauses that can be used to clean and manipulate data effectively. Each concept is explained in a clear and concise manner, accompanied by code examples that demonstrate how to apply the techniques in real-world scenarios.
Whether you are working with small or large datasets, this guide will equip you with the necessary knowledge and skills to clean and prepare your data for analysis, reporting, or any other data-driven task. By following the guidelines and code examples provided, you will be able to improve the quality and reliability of your data, leading to more accurate insights and better decision making.
So, let's dive into the world of SQL data cleaning and learn how to transform messy and inconsistent data into valuable and reliable information.
A Comprehensive Guide to SQL Data Cleaning: Including Code Examples
Understanding the Importance of SQL Data Cleaning
In the world of data analysis and management, ensuring the accuracy and reliability of data is of utmost importance. This is where SQL data cleaning comes into play. SQL, or Structured Query Language, is a programming language used for managing and manipulating relational databases. Data cleaning, on the other hand, refers to the process of identifying and correcting or removing errors, inconsistencies, and inaccuracies in datasets.
Data cleaning is a critical step in the data analysis pipeline as it directly impacts the quality and validity of the insights derived from the data. Without proper data cleaning, the results of any analysis or decision-making process can be compromised, leading to incorrect conclusions and potentially harmful actions.
One of the primary reasons why data cleaning is essential is the presence of missing values in datasets. Missing values can occur due to various reasons, such as human error during data entry, system failures, or incomplete data collection processes. These missing values can significantly affect the accuracy of any analysis performed on the dataset. Therefore, it is crucial to identify and handle missing values appropriately.
Another common issue in datasets is the presence of duplicate records. Duplicate records can arise due to data entry errors, system glitches, or merging of multiple datasets. These duplicates can skew the results of any analysis and lead to incorrect conclusions. Therefore, it is essential to identify and remove duplicate records to ensure the accuracy of the data.
In addition to missing values and duplicate records, datasets often contain outliers. Outliers are data points that deviate significantly from the rest of the data. These outliers can arise due to measurement errors, data entry mistakes, or genuine extreme values. However, outliers can distort statistical analyses and models, leading to inaccurate results. Therefore, it is crucial to detect and handle outliers appropriately.
SQL provides various functions and techniques to address these data cleaning challenges. For example, to handle missing values, SQL offers the COALESCE function, which allows replacing missing values with a specified default value. Additionally, the IS NULL and IS NOT NULL operators can be used to identify and filter out records with missing values.
To deal with duplicate records, SQL provides the DISTINCT keyword, which eliminates duplicate rows from the result set. The GROUP BY clause can also be used to group records based on specific columns, allowing for aggregation and identification of duplicates.
When it comes to handling outliers, SQL offers various statistical functions, such as AVG, MIN, MAX, and STDDEV, which can be used to calculate summary statistics and identify extreme values. Additionally, SQL provides the HAVING clause, which allows filtering records based on specific conditions, such as excluding outliers based on a certain threshold.
In conclusion, SQL data cleaning is a crucial step in ensuring the accuracy and reliability of data for analysis and decision-making purposes. By addressing issues such as missing values, duplicate records, and outliers, data cleaning helps to improve the quality of insights derived from datasets. SQL provides a range of functions and techniques to handle these challenges effectively. By utilizing these tools, data analysts and database administrators can ensure the integrity of their data and make informed decisions based on accurate information.
Common Challenges in SQL Data Cleaning and How to Overcome Them
Data cleaning is an essential step in the data analysis process. It involves identifying and correcting or removing errors, inconsistencies, and inaccuracies in the data. SQL, or Structured Query Language, is a powerful tool for managing and manipulating data. In this article, we will explore some common challenges in SQL data cleaning and provide solutions to overcome them.
One of the most common challenges in SQL data cleaning is dealing with missing values. Missing values can occur for various reasons, such as data entry errors or incomplete data. To handle missing values, you can use the IS NULL or IS NOT NULL operators in SQL. For example, to select rows with missing values in a specific column, you can use the following query:
SELECT * FROM table_name WHERE column_name IS NULL;
Alternatively, to select rows with non-missing values in a specific column, you can use the following query:
SELECT * FROM table_name WHERE column_name IS NOT NULL;
Another challenge in SQL data cleaning is handling duplicate records. Duplicate records can occur when data is entered multiple times or when merging data from different sources. To identify and remove duplicate records, you can use the DISTINCT keyword in SQL. For example, to select distinct values from a specific column, you can use the following query:
SELECT DISTINCT column_name FROM table_name;
If you want to remove duplicate records from an entire table, you can use the GROUP BY clause in combination with the HAVING clause. For example, to remove duplicate records based on a specific column, you can use the following query:
SELECT column1, column2, column3
FROM table_name
GROUP BY column1, column2, column3
HAVING COUNT(*) > 1;
Data consistency is another challenge in SQL data cleaning. Inconsistent data can arise from different data sources or human errors. To ensure data consistency, you can use SQL functions and expressions to transform and standardize the data. For example, you can use the UPPER or LOWER functions to convert text to uppercase or lowercase, respectively. You can also use the REPLACE function to replace specific characters or substrings in a column. Here's an example:
UPDATE table_name
SET column_name = REPLACE(column_name, 'old_value', 'new_value');
Data validation is crucial in SQL data cleaning. It involves checking the integrity and accuracy of the data. SQL provides various constraints, such as NOT NULL, UNIQUE, and CHECK, to enforce data validation rules. For example, you can use the NOT NULL constraint to ensure that a column does not contain any missing values. You can use the UNIQUE constraint to ensure that a column does not contain any duplicate values. You can use the CHECK constraint to define custom validation rules for a column. Here's an example:
CREATE TABLE table_name (
column1 datatype NOT NULL,
column2 datatype UNIQUE,
column3 datatype CHECK (column3 > 0)
);
In conclusion, SQL data cleaning involves addressing common challenges such as missing values, duplicate records, data consistency, and data validation. By using SQL operators, functions, expressions, and constraints, you can overcome these challenges and ensure that your data is clean and reliable. Remember to always test your SQL queries and code examples on a sample dataset before applying them to your actual data.
A Comprehensive Guide to SQL Data Cleaning: Including Code Examples
Data cleaning is a crucial step in the data analysis process. It involves identifying and correcting errors, inconsistencies, and inaccuracies in the data to ensure its quality and reliability. SQL, or Structured Query Language, is a powerful tool that can be used for data cleaning tasks. In this article, we will provide a step-by-step guide to SQL data cleaning, complete with code examples.
Step 1: Identify and Understand the Data Issues
The first step in data cleaning is to identify and understand the data issues. This can be done by examining the data and looking for patterns or inconsistencies. Common data issues include missing values, duplicate records, incorrect data types, and outliers. By understanding the nature of these issues, you can develop an effective strategy for cleaning the data.
Step 2: Remove Duplicate Records
Duplicate records can distort the analysis results and lead to incorrect conclusions. To remove duplicate records in SQL, you can use the DISTINCT keyword. For example, to retrieve a list of unique customer names from a table called "customers", you can use the following SQL query:
SELECT DISTINCT customer_name
FROM customers;
This query will return a list of unique customer names, eliminating any duplicate records.
Step 3: Handle Missing Values
Missing values are a common issue in datasets and can affect the accuracy of the analysis. There are several ways to handle missing values in SQL. One approach is to replace missing values with a default value or an average value. For example, to replace missing values in a column called "age" with the average age, you can use the following SQL query:
UPDATE table_name
SET age = (SELECT AVG(age) FROM table_name)
WHERE age IS NULL;
This query will update the missing values in the "age" column with the average age from the same table.
Step 4: Correct Data Types
Data types define the kind of data that can be stored in a column. Incorrect data types can lead to data integrity issues and affect the analysis results. To correct data types in SQL, you can use the ALTER TABLE statement. For example, to change the data type of a column called "price" from VARCHAR to DECIMAL, you can use the following SQL query:
ALTER TABLE table_name
ALTER COLUMN price DECIMAL(10,2);
This query will modify the data type of the "price" column to DECIMAL with a precision of 10 and a scale of 2.
Step 5: Remove Outliers
Outliers are extreme values that deviate significantly from the rest of the data. They can skew the analysis results and should be removed or corrected. To remove outliers in SQL, you can use the HAVING clause in combination with aggregate functions. For example, to retrieve records from a table called "sales" where the sales amount is within two standard deviations from the mean, you can use the following SQL query:
SELECT *
FROM sales
HAVING sales_amount BETWEEN (SELECT AVG(sales_amount) - 2 * STDDEV(sales_amount) FROM sales)
AND (SELECT AVG(sales_amount) + 2 * STDDEV(sales_amount) FROM sales);
This query will return records where the sales amount is within two standard deviations from the mean.
In conclusion, SQL is a powerful tool for data cleaning tasks. By following this step-by-step guide and using the provided code examples, you can effectively clean your data and ensure its quality and reliability for analysis. Remember to identify and understand the data issues, remove duplicate records, handle missing values, correct data types, and remove outliers. With these techniques, you can confidently proceed with your data analysis knowing that your data is clean and accurate.
1. What is "A Comprehensive Guide to SQL Data Cleaning: Including Code Examples"?
It is a guidebook that provides detailed information and code examples on how to clean and manipulate data using SQL.
2. What does the guide cover?
The guide covers various techniques and best practices for cleaning and transforming data using SQL, along with code examples to illustrate the concepts.
3. Who is the target audience for this guide?
The guide is aimed at SQL users and data professionals who want to learn effective data cleaning techniques using SQL.
In conclusion, "A Comprehensive Guide to SQL Data Cleaning: Including Code Examples" provides a detailed and practical resource for individuals working with SQL databases. The guide covers various techniques and strategies for cleaning and transforming data, ensuring its accuracy and reliability. With the inclusion of code examples, readers can easily understand and implement the concepts discussed. This guide serves as a valuable reference for SQL professionals seeking to improve the quality of their data and optimize their database operations.