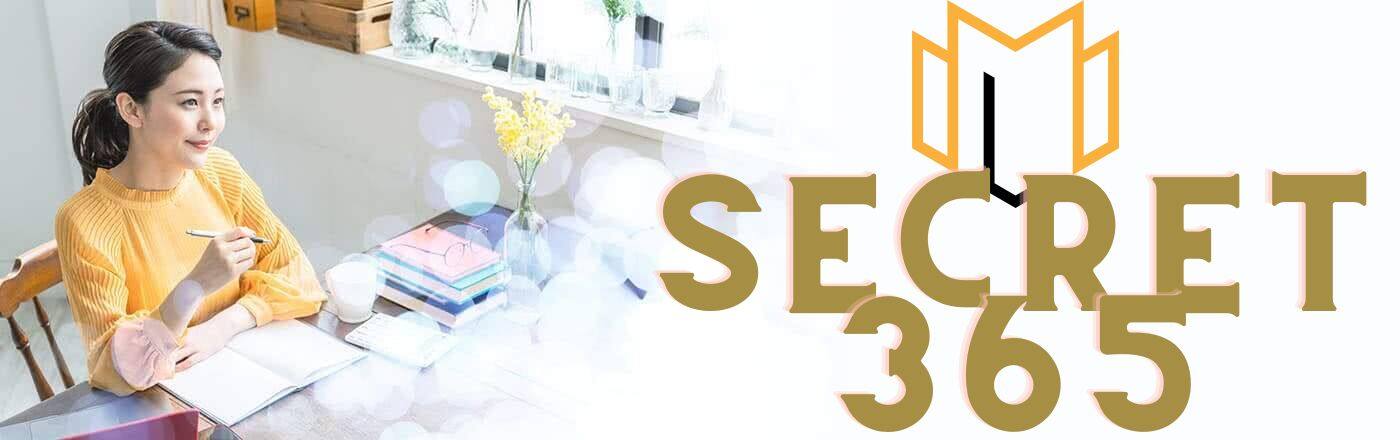
"Unlocking Efficiency: Dive into Python's Object Pooling for Optimal Performance"
Introduction:
Python's object pooling is a technique used to optimize memory usage and improve performance in applications. Object pooling involves creating a pool of pre-initialized objects that can be reused instead of creating new objects each time they are needed. This approach can be particularly beneficial in scenarios where object creation is expensive or resource-intensive.
By reusing objects from a pool, the overhead of creating and destroying objects is reduced, leading to improved efficiency. Object pooling can be especially useful in situations where objects are frequently created and destroyed, such as in network programming, database connections, or thread management.
In this article, we will explore Python's object pooling concept and discuss its benefits and considerations. We will also examine different approaches to implementing object pooling in Python, including manual implementation and using third-party libraries. Additionally, we will discuss potential trade-offs and best practices to ensure optimal efficiency when utilizing object pooling in Python applications.
Python is a versatile programming language that is widely used for various applications. One of the key features of Python is its object-oriented nature, which allows developers to create and manipulate objects. However, creating and destroying objects can be a time-consuming process, especially when dealing with large-scale applications. This is where object pooling comes into play.
Object pooling is a design pattern that aims to improve the performance and efficiency of an application by reusing objects instead of creating new ones. In Python, object pooling can be achieved using various techniques, such as using a custom object pool class or utilizing third-party libraries.
The basic idea behind object pooling is to create a pool of pre-initialized objects that can be borrowed and returned as needed. This eliminates the need for creating new objects every time they are required, thus reducing the overhead associated with object creation and destruction.
To understand how object pooling works in Python, let's consider a simple example. Suppose we have a class called "Connection" that represents a connection to a database. Creating a new connection object every time we need to interact with the database can be expensive. Instead, we can create a pool of pre-initialized connection objects and borrow one whenever we need to perform a database operation.
In Python, we can implement object pooling using a custom object pool class. This class would be responsible for managing the pool of objects and providing methods to borrow and return objects. The pool can be implemented as a list or a queue, depending on the specific requirements of the application.
When an object is borrowed from the pool, it is marked as "in use" and removed from the pool. Once the object is no longer needed, it can be returned to the pool and marked as "available" again. This way, the same object can be reused multiple times, eliminating the need for creating new objects.
Using object pooling can significantly improve the performance of an application, especially in scenarios where object creation is expensive. By reusing objects, we can reduce the overhead associated with memory allocation and deallocation, resulting in faster and more efficient code execution.
In addition to custom object pool classes, there are also third-party libraries available for object pooling in Python. These libraries provide more advanced features and functionalities, such as object expiration and automatic object creation. Some popular object pooling libraries in Python include "objpool" and "pooler".
In conclusion, object pooling is a powerful technique that can greatly enhance the efficiency and performance of Python applications. By reusing objects instead of creating new ones, we can reduce the overhead associated with object creation and destruction. Whether using a custom object pool class or a third-party library, object pooling is a valuable tool for optimizing code execution and improving overall application performance.
Python is a versatile programming language that is widely used for various applications. One of the key factors that contribute to its popularity is its simplicity and ease of use. However, when it comes to performance, Python can sometimes be slower compared to other languages. This is where object pooling comes into play.
Object pooling is a technique that allows for the reuse of objects instead of creating new ones. By reusing objects, we can reduce the overhead of object creation and destruction, resulting in improved performance. In Python, object pooling can be implemented using various techniques, each with its own advantages and trade-offs.
One common technique for implementing object pooling in Python is using a custom class. This involves creating a class that manages a pool of objects and provides methods for acquiring and releasing objects from the pool. When an object is released, it is returned to the pool instead of being destroyed, making it available for reuse.
Another technique for object pooling in Python is using a third-party library such as `object_pool`. This library provides a generic object pool implementation that can be easily integrated into your code. It allows you to define the maximum number of objects in the pool and provides methods for acquiring and releasing objects.
When implementing object pooling in Python, it is important to consider the trade-offs. While object pooling can improve performance by reducing object creation and destruction overhead, it can also increase memory usage. Objects that are not properly released back to the pool can lead to memory leaks, causing your program to consume more memory than necessary.
To mitigate the risk of memory leaks, it is important to ensure that objects are properly released back to the pool when they are no longer needed. This can be done by using a context manager or by explicitly releasing objects using the provided release method.
In addition to managing memory usage, object pooling can also help in scenarios where object creation is expensive. For example, if your program frequently creates and destroys database connections, using object pooling can significantly improve performance by reusing existing connections instead of creating new ones.
When implementing object pooling in Python, it is important to benchmark and profile your code to measure the performance improvements. This will help you identify any bottlenecks and fine-tune your object pooling implementation for optimal efficiency.
In conclusion, object pooling is a powerful technique for improving performance in Python. By reusing objects instead of creating new ones, we can reduce the overhead of object creation and destruction, resulting in improved performance. Whether you choose to implement object pooling using a custom class or a third-party library, it is important to consider the trade-offs and ensure that objects are properly released back to the pool to avoid memory leaks. By benchmarking and profiling your code, you can fine-tune your object pooling implementation for optimal efficiency.
Exploring Python's Object Pooling for Optimal Efficiency
Python is a versatile and powerful programming language that is widely used for various applications. One of the key factors that contribute to its popularity is its simplicity and ease of use. However, when it comes to optimizing performance and efficiency, Python can sometimes fall short. This is where object pooling comes into play.
Object pooling is a technique that allows for the reuse of objects instead of creating new ones. By reusing objects, we can reduce the overhead of object creation and destruction, resulting in improved performance and efficiency. Python provides several advanced object pooling strategies that can be explored to achieve optimal efficiency.
One of the most commonly used object pooling strategies in Python is the use of a simple object pool. In this strategy, a pool of pre-initialized objects is created, and whenever an object is needed, it is borrowed from the pool. Once the object is no longer needed, it is returned to the pool for future use. This eliminates the need for creating and destroying objects repeatedly, resulting in significant performance improvements.
Another advanced object pooling strategy in Python is the use of a bounded object pool. In this strategy, the pool has a maximum capacity, and if the pool is full, new requests for objects are blocked until an object becomes available. This ensures that the pool does not grow indefinitely and helps prevent resource exhaustion. Bounded object pools are particularly useful in scenarios where the number of objects needed is known in advance and can be limited.
Python also provides support for thread-safe object pooling. In multi-threaded applications, it is crucial to ensure that objects are accessed and manipulated safely by multiple threads. Python's threading module provides synchronization primitives such as locks and semaphores that can be used to implement thread-safe object pooling. By synchronizing access to the pool, we can prevent race conditions and ensure that objects are used safely by multiple threads.
In addition to the built-in object pooling strategies, Python also allows for custom object pooling implementations. This gives developers the flexibility to tailor object pooling to their specific needs and requirements. Custom object pooling implementations can take into account factors such as object initialization, object expiration, and object eviction policies. By fine-tuning these parameters, developers can further optimize the efficiency of object pooling in their applications.
It is important to note that object pooling is not a silver bullet solution for all performance and efficiency issues in Python. While it can provide significant improvements in certain scenarios, it may not always be the best approach. Object pooling introduces additional complexity and overhead, and in some cases, the benefits may not outweigh the costs. It is essential to carefully analyze the specific requirements and constraints of the application before deciding to implement object pooling.
In conclusion, Python's object pooling strategies offer advanced techniques for optimizing performance and efficiency. By reusing objects instead of creating new ones, we can reduce overhead and improve overall application performance. Whether it is a simple object pool, a bounded object pool, or a custom implementation, object pooling can be a valuable tool in the Python developer's arsenal. However, it is crucial to carefully consider the specific requirements and constraints of the application before deciding to implement object pooling. With the right approach, object pooling can help achieve optimal efficiency in Python applications.
1. What is object pooling in Python?
Object pooling is a technique used in Python to reuse and manage a pool of pre-initialized objects. It aims to improve efficiency by reducing the overhead of object creation and destruction.
2. How does object pooling work in Python?
In Python, object pooling involves creating a pool of objects upfront and keeping them in memory. When an object is needed, it is taken from the pool, used, and then returned to the pool for future reuse. This eliminates the need for frequent object creation and destruction, resulting in improved performance.
3. What are the benefits of using object pooling in Python?
Using object pooling in Python can provide several benefits, including reduced memory usage, improved performance, and decreased overhead of object creation and destruction. It can be particularly useful in scenarios where object creation is expensive or resource-intensive.
In conclusion, exploring Python's object pooling can significantly enhance the efficiency of a program. Object pooling allows for the reuse of objects, reducing the overhead of creating and destroying objects repeatedly. This can lead to improved performance, reduced memory usage, and better utilization of system resources. By implementing object pooling techniques in Python, developers can optimize their code and achieve optimal efficiency in their applications.