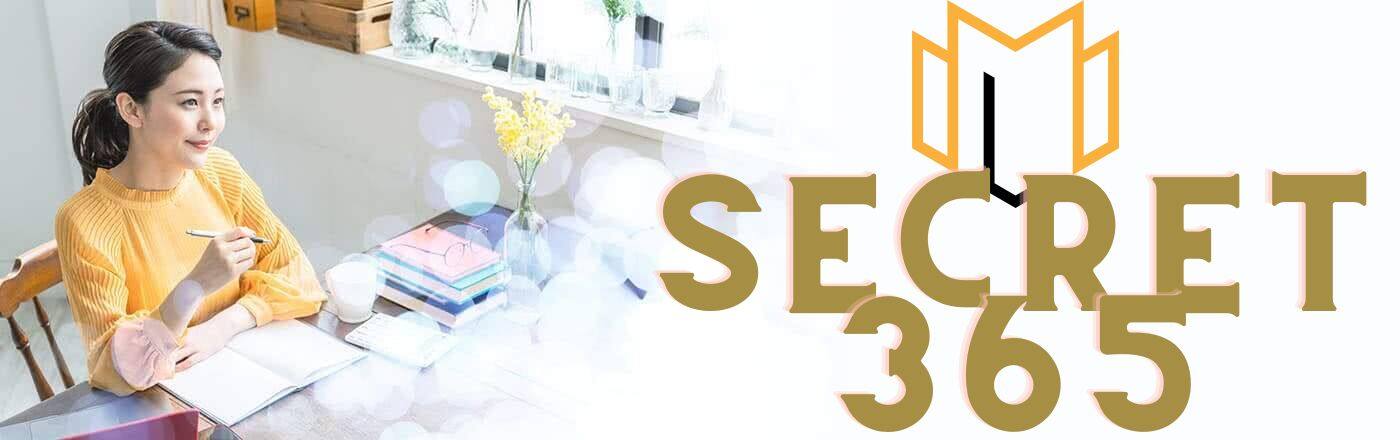
"Effortlessly integrate MediatR with ASP.NET Core for seamless notification system implementation."
Implementing MediatR with Notification System in ASP.NET Core allows developers to easily decouple the communication between different components of an application. MediatR acts as a mediator, enabling the communication between different parts of the system through the use of requests and notifications. This approach promotes a more maintainable and scalable codebase by reducing dependencies and improving code organization. By integrating MediatR with a notification system, developers can efficiently handle and broadcast events, enabling real-time updates and notifications to users.
Implementing MediatR with Notification System in ASP.NET Core
Benefits of Using MediatR for Implementing a Notification System in ASP.NET Core
When it comes to building robust and scalable applications, implementing a notification system is crucial. Notifications play a vital role in keeping users informed about important events or updates within an application. In ASP.NET Core, one popular approach to implementing a notification system is by using MediatR.
MediatR is a powerful open-source library that simplifies the implementation of the Mediator pattern in .NET applications. It provides a clean and elegant way to decouple the communication between different components of an application. By leveraging MediatR, developers can easily implement a notification system that is flexible, extensible, and maintainable.
One of the key benefits of using MediatR for implementing a notification system in ASP.NET Core is its ability to decouple the sender and receiver of notifications. Traditionally, developers would have to manually wire up the communication between different components, leading to tight coupling and increased complexity. With MediatR, this coupling is eliminated, as the sender only needs to publish a notification, and the receiver can subscribe to it without any direct dependencies.
Another advantage of using MediatR is its support for handling multiple notifications in a single request. In many scenarios, an action performed by a user may trigger multiple notifications to be sent out. With MediatR, developers can easily define and handle multiple notifications within a single request, reducing the number of round trips to the server and improving performance.
Furthermore, MediatR provides a clean and intuitive way to handle cross-cutting concerns such as logging, validation, and authorization. By leveraging the pipeline behavior feature of MediatR, developers can easily add additional logic to the notification handling process. This allows for centralized and reusable code, reducing code duplication and improving maintainability.
Additionally, MediatR promotes the use of domain events, which are a powerful concept in domain-driven design. Domain events represent significant changes or occurrences within a domain and can be used to trigger notifications. By using MediatR, developers can easily raise domain events and handle them in a decoupled manner, improving the overall design and maintainability of the application.
Another benefit of using MediatR is its extensibility. MediatR provides a simple and straightforward way to add custom behaviors and pipelines to the notification handling process. This allows developers to easily extend the functionality of MediatR to suit their specific requirements. Additionally, MediatR integrates seamlessly with other popular libraries and frameworks in the ASP.NET Core ecosystem, making it a versatile choice for implementing a notification system.
In conclusion, implementing a notification system in ASP.NET Core using MediatR offers numerous benefits. It allows for decoupled communication between components, supports handling multiple notifications in a single request, and provides a clean way to handle cross-cutting concerns. Furthermore, MediatR promotes the use of domain events and offers extensibility, making it a powerful tool for building robust and maintainable applications. By leveraging MediatR, developers can implement a flexible and scalable notification system that enhances the user experience and improves overall application performance.
Implementing MediatR with Notification System in ASP.NET Core
ASP.NET Core is a powerful framework for building web applications. It provides a flexible and scalable platform for developing modern web applications. One of the key features of ASP.NET Core is its support for MediatR, a library that enables the implementation of the Mediator pattern.
The Mediator pattern is a behavioral design pattern that allows loose coupling between components by using a mediator object to handle communication between them. This pattern promotes the principle of single responsibility and helps to keep the codebase clean and maintainable.
In this article, we will guide you through the step-by-step process of implementing MediatR with a notification system in ASP.NET Core. By the end of this guide, you will have a clear understanding of how to leverage MediatR to build a robust and efficient notification system in your ASP.NET Core application.
Step 1: Setting up the ASP.NET Core project
To get started, create a new ASP.NET Core project using your preferred development environment. Make sure to select the appropriate template and configure the necessary dependencies. Once the project is set up, we can move on to the next step.
Step 2: Installing MediatR
To use MediatR in your ASP.NET Core project, you need to install the MediatR NuGet package. Open the NuGet Package Manager Console and run the following command:
Install-Package MediatR
This will install the MediatR package and its dependencies into your project.
Step 3: Defining the notification
Next, we need to define the notification that will be sent by the system. Create a new class called "Notification" and inherit from the MediatR's "INotification" interface. This interface represents a notification that can be sent through the mediator.
Step 4: Implementing the notification handler
Now, let's implement the notification handler. Create a new class called "NotificationHandler" and inherit from the MediatR's "INotificationHandler" interface. This interface defines a handler for a specific notification.
In the "NotificationHandler" class, implement the "Handle" method to define the logic that should be executed when the notification is received. This method will be called by the mediator when a notification is sent.
Step 5: Registering MediatR in the ASP.NET Core application
To enable MediatR in your ASP.NET Core application, you need to register it in the dependency injection container. Open the "Startup.cs" file and add the following code to the "ConfigureServices" method:
services.AddMediatR(typeof(Startup));
This code registers MediatR and its dependencies in the dependency injection container.
Step 6: Sending a notification
Finally, let's send a notification from our application. In any part of your code where you want to send a notification, inject the "IMediator" interface into the constructor and use it to send the notification. For example:
public class SomeService
{
private readonly IMediator _mediator;
public SomeService(IMediator mediator)
{
_mediator = mediator;
}
public async Task DoSomething()
{
// Some logic...
await _mediator.Publish(new Notification());
// More logic...
}
}
In this example, we inject the "IMediator" interface into the "SomeService" class and use it to publish a new instance of the "Notification" class.
Conclusion
Implementing MediatR with a notification system in ASP.NET Core can greatly enhance the modularity and maintainability of your application. By following the step-by-step guide outlined in this article, you should now have a solid understanding of how to leverage MediatR to build a robust and efficient notification system in your ASP.NET Core application.
Implementing MediatR with Notification System in ASP.NET Core
ASP.NET Core is a powerful framework for building web applications, and MediatR is a popular library that helps developers implement the Mediator pattern in their applications. The Mediator pattern promotes loose coupling between components by allowing them to communicate through a central mediator. In this article, we will explore the best practices for efficiently implementing MediatR with a notification system in ASP.NET Core.
One of the key benefits of using MediatR is that it simplifies the communication between different components of an application. Instead of components directly calling each other's methods, they can send requests to the mediator, which then routes the request to the appropriate handler. This decoupling makes the code more maintainable and testable.
To implement MediatR with a notification system in ASP.NET Core, the first step is to install the MediatR NuGet package. This package provides the necessary classes and interfaces to implement the Mediator pattern. Once installed, you can start defining your requests, handlers, and notifications.
Requests represent the messages that components send to the mediator. Each request should have a corresponding handler that knows how to handle the request. Handlers are responsible for executing the logic associated with a request and returning a response if necessary. Notifications, on the other hand, are messages that components send to the mediator without expecting a response.
When defining requests, handlers, and notifications, it is important to follow the single responsibility principle. Each request, handler, or notification should have a clear and specific purpose. This helps keep the codebase organized and makes it easier to understand and maintain.
In addition to requests, handlers, and notifications, MediatR also provides the concept of behaviors. Behaviors allow you to add cross-cutting concerns to the request pipeline. For example, you can use behaviors to implement logging, validation, or authorization logic that applies to all requests. By using behaviors, you can keep your handlers focused on the core business logic and avoid duplicating code across multiple handlers.
To register MediatR in your ASP.NET Core application, you need to add the necessary services to the dependency injection container. This can be done in the ConfigureServices method of your Startup class. By registering MediatR, you make it available for injection into your controllers, services, or other components that need to send requests or notifications.
Once MediatR is registered, you can start using it in your application. To send a request or notification, you simply inject an instance of IMediator into your component and call its Send or Publish methods, respectively. The mediator takes care of routing the request or notification to the appropriate handler.
When implementing a notification system with MediatR, it is important to consider the performance implications. If you have a large number of subscribers for a particular notification, sending the notification synchronously can impact the responsiveness of your application. To mitigate this, you can use the Publish method with the Fire-and-Forget option, which sends the notification asynchronously without waiting for all subscribers to process it.
In conclusion, implementing MediatR with a notification system in ASP.NET Core can greatly improve the maintainability and testability of your application. By following the best practices outlined in this article, you can ensure that your code is organized, efficient, and easy to understand. MediatR provides a powerful abstraction for communication between components, and when combined with a notification system, it enables you to build robust and scalable applications.
1. What is MediatR in ASP.NET Core?
MediatR is a library in ASP.NET Core that provides a simple and elegant way to implement the Mediator pattern, which helps in decoupling the sender and receiver of a request.
2. How does MediatR work in ASP.NET Core?
MediatR acts as a mediator between the sender and receiver by handling requests and notifications. It allows you to define request and notification objects, along with their corresponding handlers, which are responsible for processing the requests and notifications.
3. How can MediatR be used with a notification system in ASP.NET Core?
To use MediatR with a notification system in ASP.NET Core, you can define notification objects that represent events or updates in your application. These notifications can be published using MediatR, and you can define notification handlers that will be responsible for handling these notifications and performing the necessary actions or sending notifications to external systems.
In conclusion, implementing MediatR with a notification system in ASP.NET Core can greatly enhance the modularity and maintainability of an application. MediatR provides a simple and elegant way to decouple the communication between different components, making it easier to add new features or modify existing ones without affecting the entire system. The notification system, on the other hand, allows for efficient broadcasting of events and notifications to interested parties, enabling real-time updates and improved user experience. Overall, combining MediatR with a notification system in ASP.NET Core can lead to a more scalable and flexible application architecture.