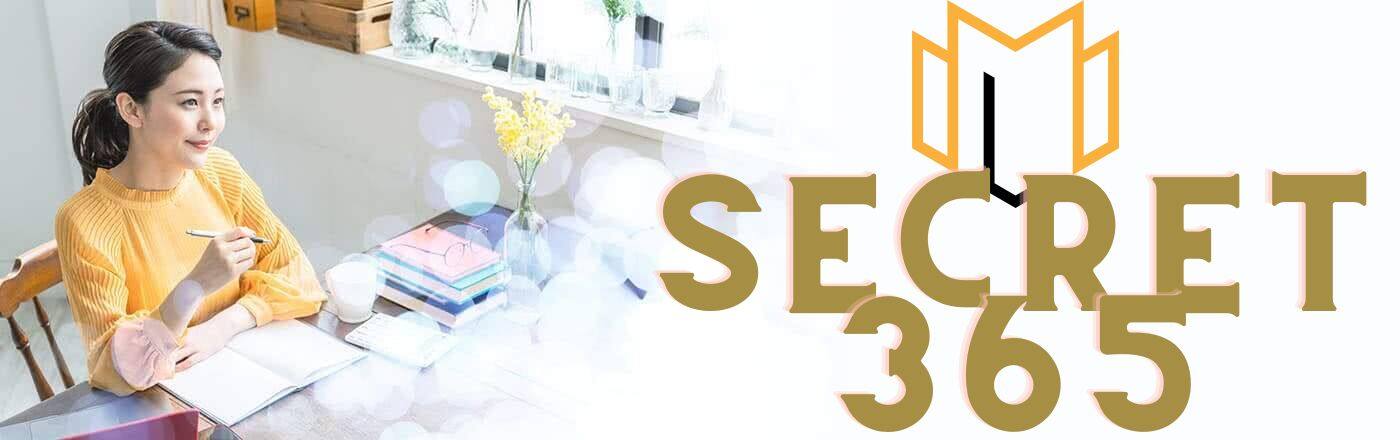
"Master Laravel development with these top 10 best practices for seamless and efficient web application creation."
Laravel is a popular PHP framework known for its elegant syntax and powerful features. To ensure efficient and maintainable code, it is important to follow best practices when developing Laravel applications. In this article, we will discuss the top 10 best practices for Laravel development. These practices include using version control, following the MVC pattern, writing clean and readable code, utilizing Laravel's built-in features, optimizing database queries, implementing caching, securing the application, writing unit tests, documenting the code, and staying up to date with Laravel updates. By adhering to these best practices, developers can create robust and scalable Laravel applications.
Laravel is a popular PHP framework known for its elegant syntax and powerful features. One of the key components of Laravel is its routing system, which allows developers to define how incoming requests should be handled. Understanding how Laravel's routing system works is essential for building robust and efficient web applications. In this article, we will explore the top 10 best practices for working with Laravel's routing system.
1. Start with the basics: Before diving into the advanced features of Laravel's routing system, it's important to understand the basics. Familiarize yourself with the concept of routes, which define the URL patterns and corresponding actions in your application.
2. Use route groups: Laravel allows you to group related routes together using the `Route::group` method. This can be useful for applying middleware, prefixing routes with a common URL segment, or sharing route attributes across multiple routes.
3. Leverage route model binding: Laravel's route model binding feature allows you to automatically inject model instances into your route callbacks. This can greatly simplify your code and make it more readable. Take advantage of this feature whenever possible.
4. Use route caching: Laravel provides a route caching mechanism that can significantly improve the performance of your application. By caching your routes, Laravel can skip the expensive route registration process on each request, resulting in faster response times.
5. Organize your routes: As your application grows, it's important to keep your routes organized. Consider splitting your routes into multiple files based on their functionality or module. This will make your codebase more maintainable and easier to navigate.
6. Name your routes: Giving meaningful names to your routes can make your code more readable and maintainable. Laravel allows you to name your routes using the `name` method. This can be particularly useful when generating URLs or redirecting to specific routes.
7. Use route model binding with slugs: If your application uses slugs in URLs, Laravel's route model binding can be a powerful tool. By binding a model to a route parameter, Laravel will automatically retrieve the corresponding model instance based on the slug value.
8. Take advantage of route model binding constraints: Laravel's route model binding constraints allow you to further customize the retrieval of model instances. You can define additional conditions or filters to ensure that the correct model is retrieved based on specific criteria.
9. Use route model binding with implicit binding: Laravel's implicit route model binding feature allows you to automatically resolve route parameters based on their type-hinted variable names. This can save you from manually retrieving model instances in your route callbacks.
10. Test your routes: Finally, it's crucial to thoroughly test your routes to ensure they are functioning as expected. Laravel provides a powerful testing framework that allows you to write unit tests for your routes. By testing your routes, you can catch any potential issues early on and ensure the stability of your application.
In conclusion, understanding Laravel's routing system is essential for building robust and efficient web applications. By following these top 10 best practices, you can make the most out of Laravel's routing capabilities. From organizing your routes to leveraging route model binding, these practices will help you write clean, maintainable, and performant code. So, go ahead and apply these best practices in your Laravel projects to take your web development skills to the next level.
Implementing Authentication and Authorization in Laravel
Laravel, the popular PHP framework, provides developers with a robust set of tools and features to build secure and scalable web applications. One of the key aspects of any web application is user authentication and authorization. In this article, we will explore the top 10 best practices for implementing authentication and authorization in Laravel.
1. Use Laravel's built-in authentication system: Laravel comes with a powerful authentication system out of the box. It provides a simple and intuitive way to handle user registration, login, and password reset functionality. Leveraging this built-in system can save you a lot of time and effort.
2. Secure passwords with hashing: Storing passwords in plain text is a major security risk. Laravel makes it easy to hash passwords using the bcrypt algorithm. By hashing passwords, you can ensure that even if your database is compromised, the passwords remain secure.
3. Implement two-factor authentication: Two-factor authentication adds an extra layer of security to your application. Laravel provides support for two-factor authentication through packages like Laravel 2FA. By enabling two-factor authentication, you can protect user accounts from unauthorized access.
4. Use middleware for authorization: Laravel's middleware feature allows you to define rules for granting or denying access to certain routes or actions. By using middleware, you can easily implement authorization checks and restrict access to specific parts of your application based on user roles or permissions.
5. Implement role-based access control: Role-based access control (RBAC) is a common approach to managing user permissions. Laravel provides a flexible RBAC system through packages like Spatie Laravel Permission. With RBAC, you can assign roles to users and define permissions for each role, giving you fine-grained control over who can access what.
6. Protect sensitive routes with authentication middleware: Some routes in your application may require authentication before they can be accessed. Laravel allows you to easily protect these routes by applying the 'auth' middleware. This ensures that only authenticated users can access sensitive parts of your application.
7. Use Laravel's authorization gates and policies: Laravel provides a powerful authorization system through gates and policies. Gates allow you to define simple authorization checks, while policies provide a more granular way to define authorization rules based on models. Leveraging these features can help you implement complex authorization logic with ease.
8. Implement password policies: Enforcing strong password policies is crucial for maintaining the security of user accounts. Laravel allows you to define password policies using validation rules. By setting requirements such as minimum length, special characters, and uppercase letters, you can ensure that users choose strong passwords.
9. Implement account lockout mechanisms: To protect against brute-force attacks, it's important to implement account lockout mechanisms. Laravel provides a built-in throttle middleware that can be used to limit the number of login attempts from a specific IP address. By configuring the throttle middleware, you can prevent attackers from guessing passwords through repeated login attempts.
10. Regularly update Laravel and its dependencies: Laravel is a constantly evolving framework, with regular updates and security patches. It's important to keep your Laravel installation up to date to benefit from the latest security enhancements. Additionally, make sure to update any third-party packages or dependencies used in your application to avoid potential vulnerabilities.
In conclusion, implementing authentication and authorization in Laravel requires careful consideration of security best practices. By following these top 10 best practices, you can ensure that your Laravel application is secure and protected against unauthorized access.
Optimizing Database Queries in Laravel
When it comes to building efficient and high-performing web applications, optimizing database queries is crucial. In Laravel, a popular PHP framework, there are several best practices that developers can follow to ensure their database queries are optimized for speed and efficiency. In this article, we will explore the top 10 best practices for optimizing database queries in Laravel.
1. Use Eloquent ORM: Laravel's Eloquent ORM (Object-Relational Mapping) provides a convenient and expressive way to interact with the database. It allows developers to write database queries using PHP syntax, making it easier to build and maintain complex queries.
2. Avoid N+1 Problem: The N+1 problem occurs when a query is executed multiple times to fetch related data. To avoid this issue, developers can use eager loading in Laravel. Eager loading allows developers to load all the necessary related data in a single query, reducing the number of database queries executed.
3. Use Indexes: Indexes are essential for improving the performance of database queries. They allow the database to quickly locate the required data. In Laravel, developers can create indexes on the columns that are frequently used in queries to speed up the query execution.
4. Limit the Number of Rows: When fetching data from the database, it is important to limit the number of rows returned. This can be achieved by using the `take` method in Laravel, which allows developers to specify the number of rows to retrieve.
5. Use Caching: Caching is a technique that stores the results of a query in memory, allowing subsequent requests to be served faster. Laravel provides a powerful caching system that developers can leverage to cache the results of frequently executed queries.
6. Use Raw Queries Sparingly: While Laravel's query builder and Eloquent ORM provide a convenient way to build database queries, there may be cases where using raw SQL queries is necessary. However, it is important to use raw queries sparingly as they can be harder to maintain and may not benefit from Laravel's query optimization features.
7. Avoid Selecting Unnecessary Columns: When fetching data from the database, it is important to only select the columns that are needed. Selecting unnecessary columns can increase the query execution time and consume more memory.
8. Use Database Transactions: Database transactions ensure that a group of database queries are executed as a single unit. This can help improve the performance of database operations by reducing the overhead of multiple queries.
9. Optimize Database Schema: The database schema plays a crucial role in the performance of database queries. Developers should ensure that the database schema is properly designed, with appropriate indexes and relationships, to optimize query execution.
10. Monitor and Analyze Query Performance: It is important to monitor and analyze the performance of database queries to identify bottlenecks and areas for improvement. Laravel provides tools like the Laravel Debugbar and query logging that can help developers analyze query performance and optimize their queries accordingly.
In conclusion, optimizing database queries is essential for building efficient and high-performing web applications in Laravel. By following these top 10 best practices, developers can ensure that their database queries are optimized for speed and efficiency, resulting in improved application performance.
1. What are the top 10 best practices for Laravel?
- Use proper folder structure and naming conventions.
- Follow the Single Responsibility Principle (SRP) for classes and methods.
- Utilize Laravel's built-in features and functions instead of reinventing the wheel.
- Implement proper error handling and logging.
- Write clean and readable code with proper indentation and comments.
- Use Laravel's ORM (Eloquent) for database interactions.
- Implement proper validation and sanitization of user input.
- Optimize database queries and utilize caching mechanisms.
- Implement proper security measures, such as CSRF protection and input validation.
- Write unit tests to ensure code quality and prevent regressions.
2. Why is using proper folder structure and naming conventions important in Laravel?
- It improves code organization and maintainability.
- It makes it easier for developers to understand and navigate the codebase.
- It follows Laravel's recommended conventions, making it easier to work with the framework and collaborate with other developers.
3. What are the benefits of using Laravel's ORM (Eloquent) for database interactions?
- It provides a simple and expressive syntax for querying and manipulating the database.
- It abstracts away the underlying database implementation, allowing for easier switching between different database systems.
- It handles relationships between database tables seamlessly, reducing the need for manual joins and complex queries.
- It provides features like eager loading and lazy loading, improving performance by reducing the number of database queries.
In conclusion, the top 10 best practices for Laravel include:
1. Following the MVC (Model-View-Controller) architectural pattern.
2. Utilizing Laravel's built-in authentication and authorization features.
3. Implementing proper error handling and logging.
4. Writing clean and readable code by following PSR coding standards.
5. Utilizing Laravel's routing system effectively.
6. Implementing caching mechanisms to improve performance.
7. Using Laravel's database migration and seeding features for database management.
8. Implementing unit tests to ensure code quality and prevent regressions.
9. Utilizing Laravel's queue system for handling background tasks.
10. Regularly updating Laravel and its dependencies to benefit from bug fixes and new features.