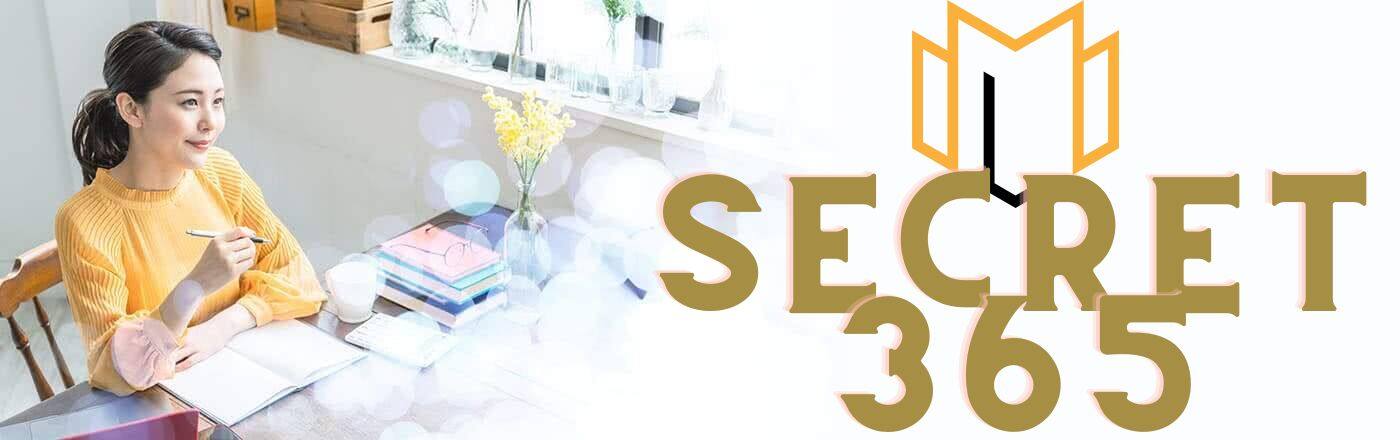
"Master ReactJS and conquer memory leaks with our comprehensive developer's guide."
The Developers' Guide to Achieving Memory Leak Immunity with ReactJS is a comprehensive resource that aims to help developers understand and address memory leaks in ReactJS applications. This guide provides insights into the causes and consequences of memory leaks, as well as practical techniques and best practices to prevent and mitigate them. By following the recommendations outlined in this guide, developers can ensure their ReactJS applications are more efficient, reliable, and immune to memory leaks.
Understanding Memory Leaks in ReactJS
Memory leaks can be a common issue for developers working with ReactJS. These leaks occur when memory that is no longer needed is not properly released, leading to a gradual depletion of available memory. This can result in decreased performance and even crashes in your application. In this article, we will explore the concept of memory leaks in ReactJS and provide some tips on how to achieve memory leak immunity.
To understand memory leaks in ReactJS, it is important to first understand how React manages memory. React uses a virtual DOM to efficiently update the actual DOM. When a component is no longer needed, React unmounts it and removes it from the virtual DOM. However, if there are any references to the component that are still held elsewhere in your code, the component will not be garbage collected and its memory will not be released.
One common cause of memory leaks in ReactJS is the improper use of event listeners. When you attach an event listener to a component, it is important to remember to remove that listener when the component is unmounted. Failure to do so will result in the listener still being referenced and the component's memory not being released. To avoid this, you can use the useEffect hook in React to add and remove event listeners when the component mounts and unmounts.
Another potential cause of memory leaks in ReactJS is the improper use of timers and intervals. If you create a timer or interval in a component and do not clear it when the component is unmounted, the timer or interval will continue to run even after the component is no longer needed. This can lead to memory leaks as the component's memory will not be released. To prevent this, you can use the useEffect hook to clear any timers or intervals when the component is unmounted.
Closures can also be a source of memory leaks in ReactJS. When you create a closure in a component, it captures the component's state and props. If the closure is not properly cleaned up when the component is unmounted, it will continue to reference the component's state and props, preventing their memory from being released. To avoid this, you can use the useCallback hook to create a memoized version of the closure and then use the useEffect hook to clean it up when the component is unmounted.
In addition to these specific causes, it is important to be mindful of general best practices when working with ReactJS to prevent memory leaks. Avoid creating unnecessary references to components or their state and props. Be cautious when using third-party libraries and ensure that they are properly managing memory. Regularly test your application for memory leaks using tools like Chrome DevTools or React's built-in Profiler.
In conclusion, memory leaks can be a common issue when working with ReactJS, but they can be avoided with proper understanding and implementation. By being mindful of event listeners, timers and intervals, closures, and general best practices, you can achieve memory leak immunity in your ReactJS applications. Remember to always test your application for memory leaks and use the available tools to identify and resolve any issues. With these tips in mind, you can ensure that your ReactJS applications perform optimally and provide a seamless user experience.
ReactJS is a popular JavaScript library used for building user interfaces. It offers a component-based architecture that allows developers to create reusable UI components. However, like any other software, ReactJS applications are not immune to memory leaks. Memory leaks can lead to performance issues and can be difficult to debug. In this article, we will discuss some best practices for preventing memory leaks in ReactJS applications.
One common cause of memory leaks in ReactJS is the improper use of event listeners. When adding event listeners to DOM elements, it is important to remove them when the component is unmounted. Failure to do so can result in memory leaks, as the event listeners will continue to reference the component even after it has been removed from the DOM. To prevent this, developers should always remove event listeners in the componentWillUnmount lifecycle method.
Another potential source of memory leaks in ReactJS is the improper use of timers and intervals. Timers and intervals can be useful for scheduling tasks or updating the UI at regular intervals. However, if not properly managed, they can also lead to memory leaks. It is important to clear timers and intervals when the component is unmounted to prevent them from continuing to run and referencing the component. This can be done in the componentWillUnmount lifecycle method as well.
In addition to event listeners and timers, another common cause of memory leaks in ReactJS is the improper use of closures. Closures can be a powerful tool in JavaScript, but they can also lead to memory leaks if not used correctly. When using closures in ReactJS, it is important to be mindful of the variables that are captured in the closure. If a closure captures a reference to a component, it can prevent the component from being garbage collected, resulting in a memory leak. To prevent this, developers should avoid capturing unnecessary variables in closures or use techniques like useCallback or useMemo to ensure that the captured variables are properly managed.
Furthermore, it is important to be mindful of the size of the data stored in the component's state. Storing large amounts of data in the state can consume a significant amount of memory and potentially lead to memory leaks. Developers should strive to keep the state as minimal as possible and avoid storing unnecessary data. If large amounts of data need to be managed, it may be more appropriate to store it in a separate data structure outside of the component's state.
Lastly, it is important to regularly test and profile ReactJS applications for memory leaks. Memory leaks can be difficult to detect and debug, especially in large applications. By regularly testing and profiling the application, developers can identify and address potential memory leaks before they become a performance issue. There are various tools available for profiling ReactJS applications, such as Chrome DevTools and React's built-in Profiler component.
In conclusion, memory leaks can be a common issue in ReactJS applications, but they can be prevented by following some best practices. Properly managing event listeners, timers, closures, and state can help prevent memory leaks and improve the performance of ReactJS applications. Regular testing and profiling can also help identify and address potential memory leaks. By following these best practices, developers can achieve memory leak immunity and ensure that their ReactJS applications perform optimally.
ReactJS is a popular JavaScript library used for building user interfaces. It offers a component-based architecture that allows developers to create reusable UI components. However, like any other software, ReactJS applications are not immune to memory leaks. Memory leaks can lead to performance issues and can even cause the application to crash. In this article, we will explore some tools and techniques that developers can use to detect and fix memory leaks in ReactJS applications.
One of the most common causes of memory leaks in ReactJS is the improper use of event listeners. When an event listener is attached to a component, it is important to remove the listener when the component is unmounted. Failure to do so can result in the listener remaining in memory even after the component is no longer needed. To avoid this, developers should always remember to remove event listeners in the componentWillUnmount lifecycle method.
Another common cause of memory leaks in ReactJS is the improper use of timers. Timers, such as setInterval and setTimeout, can be useful for scheduling tasks in a ReactJS application. However, if not properly managed, timers can continue running even after the component that created them has been unmounted. To prevent this, developers should always clear timers in the componentWillUnmount lifecycle method.
In addition to event listeners and timers, another potential source of memory leaks in ReactJS is the improper use of state. ReactJS components have a state object that can be used to store and manage data. However, if the state is not properly cleaned up when a component is unmounted, it can lead to memory leaks. To avoid this, developers should always make sure to clean up the state in the componentWillUnmount lifecycle method.
To help developers detect memory leaks in ReactJS applications, there are several tools available. One such tool is the Chrome DevTools. The DevTools provide a heap snapshot feature that allows developers to take snapshots of the memory heap at different points in time. By comparing these snapshots, developers can identify objects that are not being properly garbage collected and are therefore causing memory leaks.
Another useful tool for detecting memory leaks in ReactJS is the React Developer Tools extension for Chrome and Firefox. This extension provides a component tree view that allows developers to inspect the state and props of each component in their application. By monitoring the memory usage of each component, developers can identify components that are not being properly cleaned up and are therefore causing memory leaks.
Once a memory leak has been detected, fixing it can be a challenging task. However, there are several techniques that developers can use to address memory leaks in ReactJS applications. One such technique is to use the shouldComponentUpdate lifecycle method to prevent unnecessary re-renders of components. By implementing shouldComponentUpdate, developers can ensure that a component only updates when its props or state have actually changed, reducing the risk of memory leaks.
Another technique for fixing memory leaks in ReactJS is to use the React.memo higher-order component. React.memo can be used to memoize the result of a component's render method, preventing unnecessary re-renders. By memoizing components that do not depend on props or state changes, developers can reduce the risk of memory leaks.
In conclusion, memory leaks can be a common issue in ReactJS applications. However, by following best practices and using the right tools and techniques, developers can achieve memory leak immunity. Properly managing event listeners, timers, and state, as well as using tools like Chrome DevTools and React Developer Tools, can help developers detect and fix memory leaks. Additionally, techniques such as shouldComponentUpdate and React.memo can be used to prevent unnecessary re-renders and reduce the risk of memory leaks. By being proactive in addressing memory leaks, developers can ensure that their ReactJS applications perform optimally and provide a seamless user experience.
1. What is a memory leak in ReactJS?
A memory leak in ReactJS occurs when the application retains unnecessary references to objects in memory, preventing them from being garbage collected and leading to memory consumption issues.
2. How can memory leaks be avoided in ReactJS?
To avoid memory leaks in ReactJS, developers should ensure proper cleanup of event listeners, timers, and subscriptions, use React's built-in cleanup mechanisms like useEffect's cleanup function, and avoid unnecessary object references that can lead to memory leaks.
3. Are there any tools or techniques available to detect memory leaks in ReactJS?
Yes, there are several tools and techniques available to detect memory leaks in ReactJS. Some popular options include using the Chrome DevTools Memory panel, using performance profiling tools like React Profiler, and utilizing third-party libraries like React's StrictMode and React Leakage.
In conclusion, the Developers' Guide to Achieving Memory Leak Immunity with ReactJS provides valuable insights and strategies for developers to prevent memory leaks in their ReactJS applications. By understanding the causes and consequences of memory leaks, developers can implement best practices such as proper component lifecycle management, efficient memory allocation, and effective debugging techniques. Following the guidelines outlined in the guide can help developers build more robust and efficient ReactJS applications, ensuring a better user experience and minimizing performance issues caused by memory leaks.