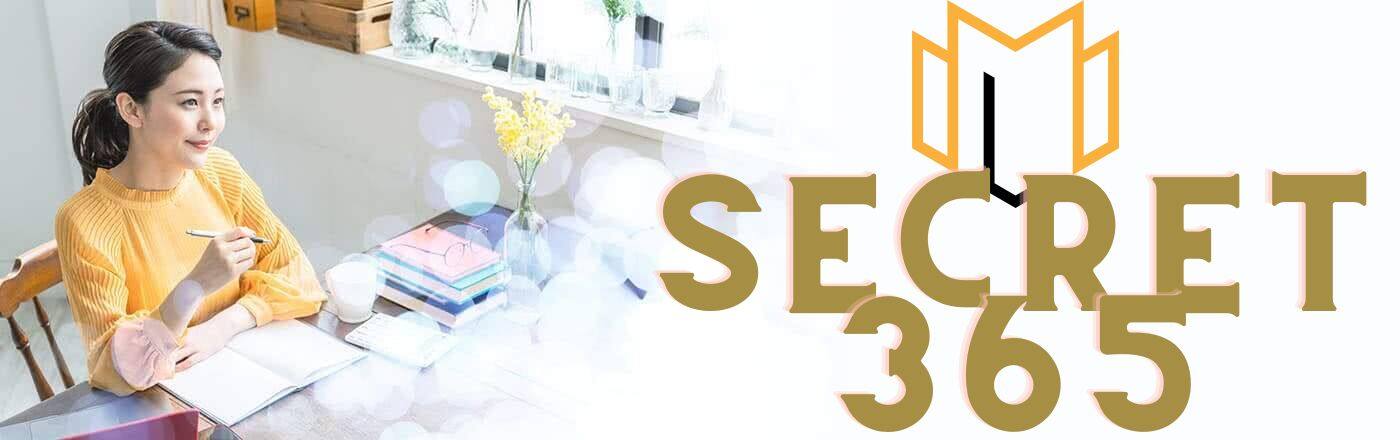
"Level up your skills with these 5 Rust projects!"
Introduction:
Rust is a powerful systems programming language known for its focus on safety, speed, and concurrency. If you're looking to enhance your Rust skills and gain hands-on experience, working on projects is an excellent way to do so. In this article, we will explore five Rust projects that can help you improve your skills and deepen your understanding of the language. These projects cover a range of topics, from building command-line tools to creating web applications, allowing you to explore different aspects of Rust development. So, let's dive in and discover these exciting projects that can take your Rust skills to the next level.
Rust is a powerful programming language that has gained popularity in recent years due to its focus on safety, performance, and concurrency. If you're looking to improve your skills in Rust, building a simple web server is a great project to undertake. In this article, we will explore the steps involved in building a basic web server using Rust.
To get started, you'll need to have Rust installed on your machine. If you haven't already done so, head over to the official Rust website and follow the installation instructions. Once you have Rust installed, you can create a new project by running the command `cargo new web_server`. This will create a new directory called `web_server` with the necessary files for a Rust project.
Next, navigate to the `web_server` directory and open the `Cargo.toml` file. This file is used to manage dependencies for your project. Add the following lines to the file:
```
[dependencies]
hyper = "0.14"
tokio = { version = "1", features = ["full"] }
```
These dependencies, `hyper` and `tokio`, are popular libraries for building web servers in Rust. `hyper` provides a high-level API for handling HTTP requests and responses, while `tokio` is a runtime for asynchronous programming.
Now that we have our dependencies set up, let's start writing some code. Create a new file called `main.rs` in the `src` directory and add the following code:
```rust
use hyper::{Body, Request, Response, Server};
use hyper::service::{make_service_fn, service_fn};
use std::convert::Infallible;
async fn handle_request(_req: Request) -> Result<Response, Infallible> {
Ok(Response::new(Body::from("Hello, World!")))
}
#[tokio::main]
async fn main() {
let make_svc = make_service_fn(|_conn| {
async {
Ok::(service_fn(handle_request))
}
});
let addr = ([127, 0, 0, 1], 3000).into();
let server = Server::bind(&addr).serve(make_svc);
if let Err(e) = server.await {
eprintln!("server error: {}", e);
}
}
```
This code sets up a basic web server that listens on `localhost:3000` and responds with the message "Hello, World!" for every request it receives. The `handle_request` function is responsible for processing incoming requests and returning a response.
To run the web server, open a terminal, navigate to the `web_server` directory, and run the command `cargo run`. You should see output indicating that the server is running. Open your web browser and visit `http://localhost:3000` to see the "Hello, World!" message.
Congratulations! You have successfully built a simple web server in Rust. This project is a great starting point for further exploration and experimentation. You can modify the `handle_request` function to handle different types of requests, add routing logic, or integrate with a database.
Building a web server in Rust not only improves your skills in the language but also gives you a deeper understanding of how web applications work. Rust's focus on safety and performance makes it an excellent choice for building robust and efficient web servers.
In conclusion, if you're looking to improve your skills in Rust, building a simple web server is a fantastic project to undertake. By following the steps outlined in this article, you can create a basic web server that responds to HTTP requests. This project will not only enhance your understanding of Rust but also provide you with valuable experience in building web applications. So, grab your keyboard and start coding!
Rust is a powerful programming language that has gained popularity in recent years due to its focus on safety, performance, and concurrency. If you're looking to improve your skills in Rust, one great way to do so is by creating a command line tool. In this article, we will explore five Rust projects that will help you enhance your programming abilities.
Creating a command line tool in Rust is an excellent project for several reasons. First, it allows you to practice the fundamentals of the language, such as working with variables, functions, and control flow. Second, it gives you the opportunity to learn about Rust's standard library and its built-in modules for handling command line arguments, file I/O, and more. Lastly, building a command line tool will help you understand how to structure a larger Rust project and manage dependencies.
To get started, you can begin by defining the functionality of your command line tool. Think about what problem it will solve or what task it will automate. For example, you could create a tool that renames files in a directory or converts images to different formats. Once you have a clear idea of what your tool will do, you can start writing the code.
In Rust, you can use the `std::env` module to handle command line arguments. This module provides functions to access the arguments passed to your program and parse them as needed. You can also use the `std::fs` module to perform file operations, such as reading and writing files. By leveraging these modules, you can build a robust command line tool that interacts with the user and manipulates files.
As you develop your command line tool, you will likely encounter challenges and obstacles along the way. This is where Rust's error handling mechanisms come into play. Rust encourages developers to handle errors explicitly, which leads to more reliable and robust code. You can use the `Result` type and the `?` operator to propagate errors and handle them gracefully. By practicing error handling in your project, you will become more proficient in writing Rust code that is resilient and handles unexpected situations.
Another aspect to consider when creating a command line tool in Rust is testing. Rust has a built-in testing framework that allows you to write unit tests for your code. By writing tests, you can ensure that your tool behaves as expected and catches any bugs or regressions. Testing is an essential skill for any developer, and by incorporating it into your project, you will improve your ability to write reliable and maintainable code.
Once you have completed your command line tool, you can take it a step further by publishing it on a package registry like crates.io. This will give you the opportunity to share your work with the Rust community and receive feedback from other developers. Publishing your project will also teach you about versioning, documentation, and the process of releasing software.
In conclusion, creating a command line tool in Rust is an excellent project to improve your skills in the language. It allows you to practice the fundamentals, learn about Rust's standard library, and gain experience in structuring larger projects. By tackling this project, you will enhance your understanding of Rust's error handling mechanisms, testing practices, and the process of publishing software. So, pick a problem to solve, start coding, and watch your skills in Rust grow.
Rust is a powerful programming language that has gained popularity in recent years due to its focus on safety, performance, and concurrency. If you're looking to improve your skills in Rust, one great project to undertake is developing a concurrent chat application. In this article, we will explore the steps involved in creating such an application and how it can help you enhance your Rust programming abilities.
To begin with, it's important to understand the concept of concurrency in Rust. Concurrency refers to the ability of a program to execute multiple tasks simultaneously. Rust provides several tools and features to handle concurrency, such as threads, channels, and locks. By developing a concurrent chat application, you will gain hands-on experience in utilizing these tools effectively.
The first step in developing a concurrent chat application is to set up the basic structure of the program. This involves creating a server that can handle multiple client connections simultaneously. Rust's standard library provides the necessary modules to create a TCP server, making it relatively straightforward to implement.
Once the server is set up, the next step is to establish communication between the server and the clients. This can be achieved using channels, which allow for message passing between different threads. In Rust, channels are implemented using the `mpsc` module. By utilizing channels, you can ensure that messages are sent and received in a synchronized manner, preventing any data races or conflicts.
After establishing communication, the next task is to implement the chat functionality. This involves allowing clients to send messages to the server, which will then broadcast them to all connected clients. To achieve this, you can maintain a list of connected clients on the server and use channels to send messages to each client. Rust's ownership system ensures that only one thread can access the list of clients at a time, preventing any data corruption.
To enhance the functionality of the chat application, you can also implement features such as private messaging and user authentication. Private messaging allows clients to send messages to specific users instead of broadcasting them to all connected clients. User authentication ensures that only authorized users can access the chat application, adding an extra layer of security.
Throughout the development process, it's important to ensure that your code is safe and free from any potential bugs or vulnerabilities. Rust's strong type system and ownership model help in preventing common programming errors such as null pointer dereferences and data races. Additionally, Rust's borrow checker enforces strict rules on memory management, eliminating the need for manual memory allocation and deallocation.
By undertaking the project of developing a concurrent chat application in Rust, you will not only improve your skills in the language but also gain a deeper understanding of concurrency and its challenges. This project will allow you to explore Rust's powerful features for handling concurrency, such as threads, channels, and locks. Moreover, it will provide you with practical experience in building a real-world application that can be expanded and customized further.
In conclusion, developing a concurrent chat application in Rust is an excellent project to enhance your skills in the language. It allows you to explore Rust's concurrency features and gain hands-on experience in building a real-world application. By undertaking this project, you will not only improve your Rust programming abilities but also deepen your understanding of concurrency and its implementation in a safe and efficient manner.
1. What are some popular Rust projects to improve your skills?
- Tokio: A runtime for asynchronous programming in Rust.
- Rocket: A web framework for building fast and secure web applications.
- Serde: A powerful serialization and deserialization library for Rust.
- Diesel: An ORM and query builder for Rust, making it easier to interact with databases.
- Actix: A high-performance, actor-based framework for building concurrent applications in Rust.
2. How can working on these projects improve your Rust skills?
- By working on these projects, you can gain hands-on experience with Rust's unique features and idioms, such as its ownership system and asynchronous programming model.
- You will learn how to build efficient and performant applications using Rust's memory safety guarantees.
- Working with popular libraries and frameworks will expose you to best practices and patterns in the Rust ecosystem.
- Collaborating with other developers on these projects can help you improve your teamwork and communication skills.
3. Are there any resources available to help beginners get started with these projects?
- Each project typically has its own documentation and community resources, such as tutorials, guides, and forums, to help beginners get started.
- Online platforms like GitHub and Stack Overflow can provide additional resources, code examples, and discussions related to these projects.
- Rust's official website (https://www.rust-lang.org/) offers a wealth of learning materials, including a book called "The Rust Programming Language" that covers various aspects of Rust development.
In conclusion, engaging in Rust projects can significantly enhance one's skills in the language. Five notable projects that can contribute to skill improvement include building a web server, creating a command-line tool, developing a game, implementing a concurrent data structure, and contributing to open-source projects. These projects offer opportunities to explore various aspects of Rust programming, such as networking, system-level programming, game development, concurrent programming, and collaboration with the wider Rust community. By undertaking these projects, individuals can gain practical experience, deepen their understanding of Rust concepts, and enhance their problem-solving abilities.