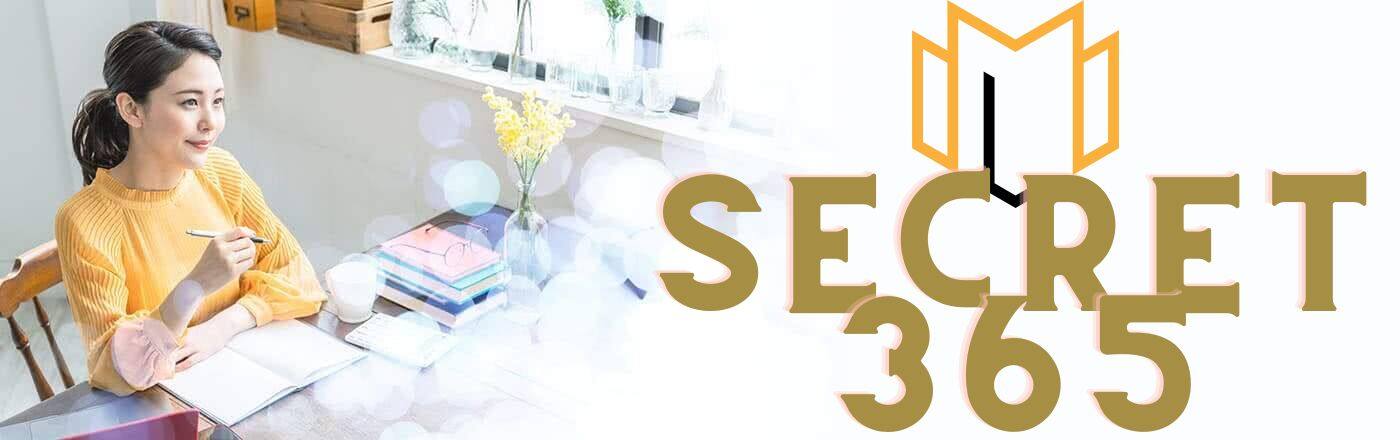
Understanding the Diamond Problem in Java: A Quick Overview
Understanding the Diamond Problem in Java: A Quick Overview
The Diamond Problem is a term used in object-oriented programming, specifically in the context of multiple inheritance. It refers to a situation where a class inherits from two or more classes that have a common superclass. This can lead to ambiguity and conflicts when accessing methods or variables from the common superclass. In Java, which does not support multiple inheritance, the Diamond Problem can still occur when using interfaces. This article provides a quick overview of the Diamond Problem in Java, explaining its causes and potential solutions.
Understanding the Diamond Problem in Java: A Quick Overview
In the world of programming, Java is a widely used language known for its simplicity and versatility. However, like any programming language, Java has its own set of challenges and complexities. One such challenge is the Diamond Problem, which can cause confusion and errors in Java programs. In this article, we will provide a quick overview of the Diamond Problem in Java and explain why it occurs.
The Diamond Problem is a term used to describe a specific issue that arises when multiple inheritance is implemented in a programming language. Multiple inheritance refers to the ability of a class to inherit properties and methods from more than one parent class. While Java does not support multiple inheritance for classes, it does allow for multiple inheritance through interfaces.
So, what exactly is the Diamond Problem in Java? Imagine a scenario where Class A and Class B both implement the same interface, let's call it Interface X. Now, let's say we have a Class C that extends both Class A and Class B. In this situation, Class C inherits the methods and properties from both Class A and Class B, including the methods defined in Interface X.
Here's where the problem arises. If both Class A and Class B have a method with the same name and signature, and Class C tries to call that method, which implementation should it use? This ambiguity is what leads to the Diamond Problem. Java does not provide a clear resolution for this conflict, and it becomes the responsibility of the programmer to handle it.
To understand why the Diamond Problem occurs, we need to delve into the concept of method overriding in Java. Method overriding allows a subclass to provide its own implementation of a method that is already defined in its superclass. When a method is called on an object of the subclass, the overridden method in the subclass is executed instead of the superclass's method.
In the case of the Diamond Problem, both Class A and Class B have a method with the same name and signature. When Class C tries to call this method, it becomes ambiguous as to which implementation should be used. This ambiguity arises because Class C has inherited the method from both Class A and Class B.
To resolve the Diamond Problem, Java provides a simple rule: the subclass must provide its own implementation of the ambiguous method. This means that Class C, in our example, must override the method defined in Interface X and provide its own implementation. By doing so, Class C clarifies which implementation should be used when the method is called.
In conclusion, the Diamond Problem in Java is a challenge that arises when multiple inheritance is implemented through interfaces. It occurs when a class inherits methods from multiple parent classes, leading to ambiguity when calling methods with the same name and signature. To resolve this ambiguity, Java requires the subclass to provide its own implementation of the ambiguous method. By understanding the Diamond Problem and following Java's rules, programmers can effectively handle this issue and ensure the smooth execution of their Java programs.
Understanding the Diamond Problem in Java: A Quick Overview
In the world of programming, Java is a widely used language known for its simplicity and versatility. However, like any programming language, Java has its own set of challenges that developers must navigate. One such challenge is the Diamond Problem, which occurs when multiple inheritance is involved.
The Diamond Problem is a term coined to describe a specific issue that arises when a class inherits from two or more classes that have a common superclass. In Java, multiple inheritance is not directly supported, but it can be achieved through the use of interfaces. This is where the Diamond Problem can come into play.
To understand how the Diamond Problem occurs in Java, let's consider a simple example. Suppose we have a class called Animal, which has two subclasses: Mammal and Bird. Both Mammal and Bird inherit from Animal, as they share common characteristics such as the ability to move and reproduce.
Now, let's introduce another class called FlyingBird, which extends the Bird class. FlyingBird represents a specific type of bird that has the ability to fly. Additionally, let's create a class called Bat, which extends both Mammal and FlyingBird. The Bat class represents a mammal that can fly, like a bat in the real world.
At first glance, this class hierarchy may seem reasonable. However, a problem arises when we try to access a method or variable that is defined in both the Bird and Mammal classes. Since Bat inherits from both classes, it is unclear which version of the method or variable should be used.
This ambiguity is the essence of the Diamond Problem. When a class inherits from two or more classes that have a common superclass, and those classes define the same method or variable, Java cannot determine which version to use. This leads to a compilation error, as the code is ambiguous and cannot be resolved.
To overcome the Diamond Problem, Java provides a mechanism called default methods. Default methods were introduced in Java 8 and allow interfaces to provide a default implementation for a method. By using default methods, we can avoid conflicts when multiple inheritance is involved.
In our example, we can define a default method in the Bird and Mammal interfaces that provides a common implementation for the conflicting method. This way, when the Bat class inherits from both interfaces, it can use the default implementation without any ambiguity.
It's important to note that the Diamond Problem is not limited to Java. It is a common issue in programming languages that support multiple inheritance, such as C++. However, Java's approach of using interfaces and default methods provides a solution that allows developers to work around the problem effectively.
In conclusion, the Diamond Problem is a challenge that arises when a class inherits from two or more classes that have a common superclass. In Java, this problem can be resolved by using interfaces and default methods. By understanding the nature of the Diamond Problem and how it occurs, developers can write cleaner and more efficient code in Java.
Solutions to Resolve the Diamond Problem in Java
Now that we have a clear understanding of what the Diamond Problem is in Java, let's explore some solutions to resolve this issue. As mentioned earlier, the Diamond Problem occurs when a class inherits from two or more classes that have a common superclass. This leads to ambiguity in method resolution, as the compiler cannot determine which superclass method to call. Fortunately, Java provides several mechanisms to address this problem.
One solution is to use the "super" keyword to explicitly specify which superclass method to call. By prefixing the method call with "super", we can indicate the desired superclass. This approach ensures that the correct method is invoked, eliminating any ambiguity. However, it requires manual intervention and can be cumbersome if there are multiple conflicting methods.
Another solution is to use interfaces instead of classes for multiple inheritance. In Java, a class can implement multiple interfaces, allowing it to inherit behavior from multiple sources without the Diamond Problem. By defining interfaces that contain the desired methods, we can avoid conflicts and achieve the desired functionality. This approach promotes code reusability and flexibility, as classes can implement different combinations of interfaces based on their requirements.
Additionally, Java 8 introduced default methods in interfaces, which provide a solution to the Diamond Problem. Default methods are methods defined within an interface that have a default implementation. When a class implements multiple interfaces with conflicting default methods, it must explicitly override the method to resolve the conflict. This approach allows for more flexibility in method resolution and avoids the Diamond Problem altogether.
Furthermore, the use of abstract classes can help mitigate the Diamond Problem. Abstract classes are classes that cannot be instantiated and serve as a blueprint for subclasses. By defining abstract classes that contain the desired methods, we can provide a common implementation that subclasses can inherit. This approach allows for code reuse and avoids conflicts that arise from multiple inheritance.
In some cases, refactoring the code to eliminate the need for multiple inheritance may be the best solution. By redesigning the class hierarchy or using composition instead of inheritance, we can avoid the Diamond Problem altogether. This approach requires careful analysis of the code and may involve significant changes, but it can lead to a more robust and maintainable codebase.
Lastly, using design patterns such as the Decorator pattern or the Strategy pattern can provide alternative solutions to the Diamond Problem. These patterns allow for dynamic behavior extension without the need for multiple inheritance. By encapsulating behavior in separate classes and composing them at runtime, we can achieve the desired functionality without the conflicts associated with multiple inheritance.
In conclusion, the Diamond Problem in Java can be resolved using various solutions. Whether it's using the "super" keyword, interfaces, default methods, abstract classes, refactoring, or design patterns, there are multiple approaches to tackle this issue. Each solution has its own advantages and considerations, and the choice depends on the specific requirements of the codebase. By understanding the Diamond Problem and exploring these solutions, developers can effectively address this challenge and write robust and maintainable Java code.
1. What is the Diamond Problem in Java?
The Diamond Problem is a term used to describe a scenario in multiple inheritance where a class inherits from two or more classes that have a common superclass. This can lead to ambiguity in method resolution.
2. How does the Diamond Problem occur in Java?
In Java, the Diamond Problem occurs when a class inherits from two or more interfaces that have a common method with the same signature. If the class does not provide an implementation for that method, it becomes ambiguous which interface's method should be used.
3. How can the Diamond Problem be resolved in Java?
To resolve the Diamond Problem in Java, the class inheriting from multiple interfaces can provide its own implementation for the ambiguous method. Alternatively, the class can explicitly specify which interface's method should be used by using the interface name followed by the method name when calling it.
In conclusion, understanding the Diamond Problem in Java is crucial for developers to avoid conflicts and ambiguity when dealing with multiple inheritance and interface implementation. By recognizing the potential issues and utilizing techniques such as default methods and explicit method overriding, developers can effectively manage and resolve the Diamond Problem in their Java programs.