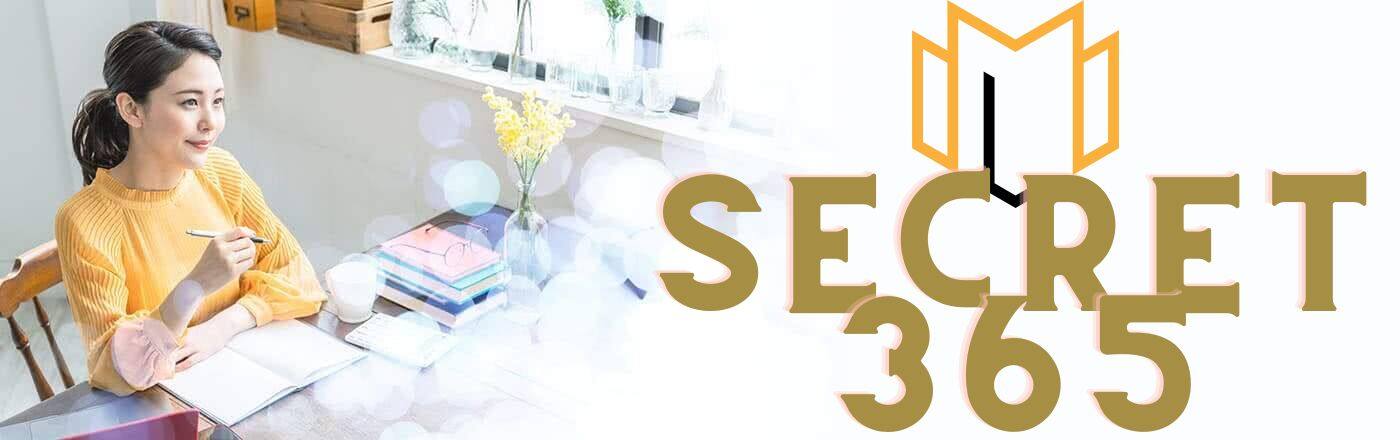
Simplify relationship mapping with Hibernate & JPA: @OneToMany
The @OneToMany annotation in Hibernate and JPA is used to establish a one-to-many relationship between two entities. It allows one entity to have multiple instances of another entity associated with it. This annotation is commonly used in object-relational mapping to map the relationship between database tables and Java objects. By mastering relationship mapping with Hibernate and JPA, developers can effectively manage and manipulate complex data relationships in their applications.
Understanding the @OneToMany Relationship Mapping in Hibernate & JPA
When working with relational databases, it is common to have relationships between tables. One such relationship is the one-to-many relationship, where one entity is associated with multiple instances of another entity. In Hibernate and JPA, this relationship can be mapped using the @OneToMany annotation.
The @OneToMany annotation is used to define a one-to-many relationship between two entities. It is typically used in the parent entity to specify a collection of child entities. For example, consider a scenario where we have a Customer entity and an Order entity. A customer can have multiple orders, so we can use the @OneToMany annotation in the Customer entity to map this relationship.
To use the @OneToMany annotation, we need to specify the target entity, which is the entity that represents the "many" side of the relationship. In our example, the target entity would be the Order entity. We also need to specify the mappedBy attribute, which is used to indicate the property in the target entity that maps back to the parent entity. In our case, the mappedBy attribute would be set to "customer" since the Order entity has a reference to the Customer entity.
In addition to the target entity and mappedBy attribute, there are other optional attributes that can be used with the @OneToMany annotation. One such attribute is cascade, which specifies the operations that should be cascaded from the parent entity to the child entities. For example, if we set the cascade attribute to CascadeType.ALL, any changes made to the Customer entity will be cascaded to the associated Order entities.
Another optional attribute is fetch, which determines how the child entities should be fetched from the database. By default, the fetch attribute is set to FetchType.LAZY, which means that the child entities will be loaded lazily when accessed. However, if we want to eagerly load the child entities along with the parent entity, we can set the fetch attribute to FetchType.EAGER.
It is worth noting that the @OneToMany annotation can be used with both collections and maps. If we want to use a collection to represent the child entities, we can use the java.util.Collection interface or one of its subinterfaces, such as java.util.List or java.util.Set. On the other hand, if we want to use a map, we can use the java.util.Map interface.
In conclusion, the @OneToMany annotation is a powerful tool in Hibernate and JPA for mapping one-to-many relationships between entities. By using this annotation, we can easily define and manage relationships between entities in our application. It is important to understand the various attributes that can be used with the @OneToMany annotation, such as target entity, mappedBy, cascade, and fetch, as they provide flexibility and control over the relationship mapping. With a solid understanding of the @OneToMany annotation, we can master relationship mapping in Hibernate and JPA and build robust and efficient applications.
Mastering Relationship Mapping with Hibernate & JPA: @OneToMany
When it comes to implementing relationship mapping in Hibernate and JPA, the @OneToMany annotation plays a crucial role. This annotation allows us to establish a one-to-many relationship between two entities, where one entity can have multiple instances of another entity associated with it. In this article, we will explore some best practices for implementing the @OneToMany relationship mapping with Hibernate and JPA.
First and foremost, it is important to understand the nature of the relationship between the entities involved. In a @OneToMany relationship, one entity acts as the parent, while the other entity acts as the child. The parent entity can have multiple child entities associated with it. For example, consider a scenario where we have a parent entity called "Department" and a child entity called "Employee." A department can have multiple employees working under it.
To establish this relationship in Hibernate and JPA, we need to annotate the parent entity's field or property with the @OneToMany annotation. This annotation takes various attributes, such as targetEntity, mappedBy, cascade, and fetch, which help define the behavior of the relationship.
The targetEntity attribute specifies the type of the child entity. It is important to note that the child entity must have a reference to the parent entity. This can be achieved by annotating a field or property in the child entity with the @ManyToOne annotation. This annotation establishes a many-to-one relationship between the child and parent entities.
The mappedBy attribute in the @OneToMany annotation is used to specify the field or property in the child entity that maps the relationship back to the parent entity. This field or property should be annotated with the @ManyToOne annotation and should have a reference to the parent entity.
Another important attribute in the @OneToMany annotation is cascade. This attribute defines the cascading behavior of the relationship. For example, if we set cascade = CascadeType.ALL, any changes made to the parent entity will be cascaded to the child entities. This means that if we delete a department, all the associated employees will also be deleted.
The fetch attribute in the @OneToMany annotation determines how the child entities are fetched from the database. By default, it is set to FetchType.LAZY, which means that the child entities are loaded lazily when accessed. However, if we want to eagerly load the child entities along with the parent entity, we can set fetch = FetchType.EAGER.
It is worth mentioning that when using the @OneToMany annotation, we should also consider the use of collections to store the child entities. Hibernate and JPA provide various collection types, such as List, Set, and Map, which can be used to store the child entities. The choice of collection type depends on the specific requirements of the application.
In conclusion, mastering the @OneToMany relationship mapping with Hibernate and JPA is essential for building robust and efficient applications. By understanding the nature of the relationship, using the appropriate annotations and attributes, and considering the use of collections, we can implement this relationship mapping effectively. Following these best practices will ensure that our application's data model accurately represents the real-world relationships between entities and allows for seamless navigation and manipulation of the data.
Mastering Relationship Mapping with Hibernate & JPA: @OneToMany
When it comes to mapping relationships between entities in a database, Hibernate and JPA provide powerful tools that can simplify the process. One such relationship is the @OneToMany mapping, which allows us to define a one-to-many relationship between two entities. In this article, we will explore some advanced techniques for mastering @OneToMany relationship mapping with Hibernate and JPA.
Before diving into the advanced techniques, let's first understand the basics of @OneToMany mapping. In a one-to-many relationship, one entity is associated with multiple instances of another entity. For example, a Customer entity can have multiple Order entities associated with it. To establish this relationship, we use the @OneToMany annotation in Hibernate and JPA.
One of the challenges in @OneToMany mapping is deciding how to handle cascading operations. Cascading operations allow us to propagate changes from the parent entity to its associated child entities. For example, if we delete a Customer entity, we may want to delete all associated Order entities as well. Hibernate and JPA provide different options for cascading operations, such as CascadeType.ALL, CascadeType.PERSIST, and CascadeType.REMOVE. By carefully selecting the appropriate cascade type, we can ensure that changes are propagated correctly.
Another important consideration in @OneToMany mapping is the fetch strategy. The fetch strategy determines how the associated entities are loaded from the database. Hibernate and JPA offer two fetch strategies: eager and lazy. In eager fetching, all associated entities are loaded immediately when the parent entity is fetched. On the other hand, lazy fetching loads the associated entities only when they are explicitly accessed. Choosing the right fetch strategy can have a significant impact on performance, especially when dealing with large datasets.
In addition to cascading operations and fetch strategies, @OneToMany mapping also requires us to handle bidirectional relationships. In a bidirectional relationship, both entities have a reference to each other. For example, a Customer entity may have a list of Order entities, while each Order entity has a reference to the Customer entity. To establish a bidirectional relationship, we need to use the mappedBy attribute in the @OneToMany annotation. This attribute specifies the field in the child entity that maps to the parent entity. By correctly configuring the mappedBy attribute, we can ensure that both sides of the relationship are properly synchronized.
When working with @OneToMany mapping, it is also important to consider the performance implications. Loading a large number of associated entities can result in performance issues, such as increased memory consumption and slower query execution. To mitigate these issues, Hibernate and JPA provide pagination and batch fetching techniques. Pagination allows us to load a subset of associated entities at a time, while batch fetching allows us to load multiple associated entities in a single database query. By using these techniques wisely, we can optimize the performance of our @OneToMany mappings.
In conclusion, mastering @OneToMany relationship mapping with Hibernate and JPA requires a deep understanding of advanced techniques. By carefully selecting cascade types, fetch strategies, and handling bidirectional relationships, we can establish robust and efficient mappings. Additionally, considering performance implications and using pagination and batch fetching techniques can further enhance the performance of our @OneToMany mappings. With these advanced techniques in our toolkit, we can confidently tackle complex one-to-many relationships in our database applications.
1. What is the purpose of @OneToMany in Hibernate & JPA?
The purpose of @OneToMany in Hibernate & JPA is to establish a one-to-many relationship between two entities, where one entity can have multiple instances of the other entity.
2. How is @OneToMany annotation used in Hibernate & JPA?
@OneToMany annotation is used to annotate a collection or a map attribute in an entity class, indicating that it has a one-to-many relationship with another entity. It requires specifying the target entity class and the mapping column(s) that represent the relationship.
3. What are the benefits of using @OneToMany in Hibernate & JPA?
Using @OneToMany in Hibernate & JPA allows for easy management of one-to-many relationships between entities. It provides convenient methods for adding, removing, and querying related entities. It also helps in maintaining data integrity and optimizing database queries.
In conclusion, mastering relationship mapping with Hibernate and JPA's @OneToMany annotation allows developers to establish a one-to-many relationship between entities in a database. This annotation simplifies the process of mapping and managing relationships, making it easier to work with complex data models and improve the efficiency of database operations.