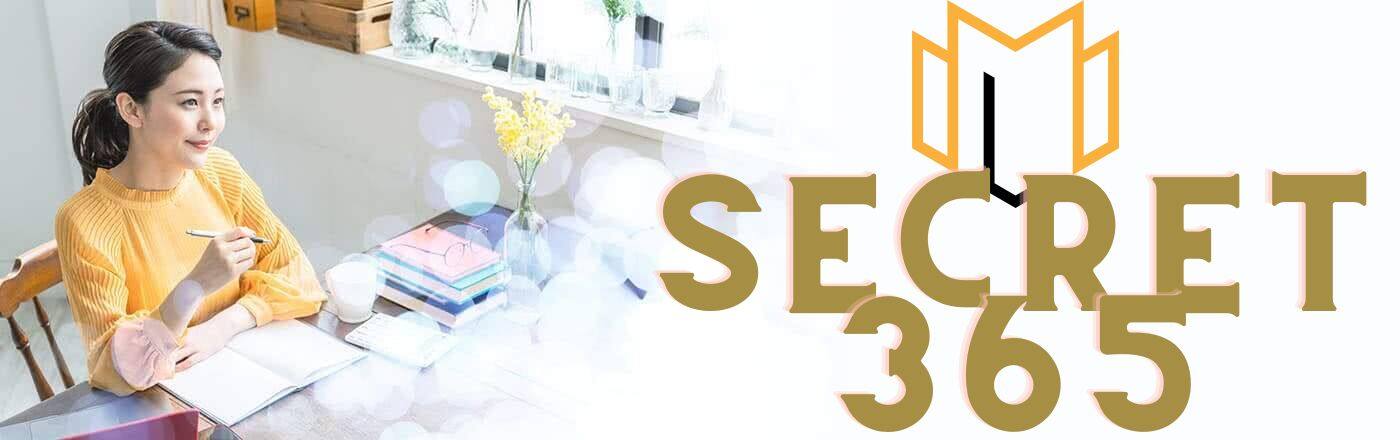
Learn TypeScript from scratch and level up your coding skills
Introduction to TypeScript for Beginners
TypeScript is a programming language that is a superset of JavaScript. It was developed by Microsoft and provides optional static typing, which allows developers to catch errors and bugs during the development process. TypeScript offers many features that enhance JavaScript, such as support for classes, interfaces, and modules. This introduction to TypeScript is designed for beginners who are familiar with JavaScript and want to learn how to leverage the benefits of static typing and other advanced features provided by TypeScript. By the end of this guide, you will have a solid understanding of TypeScript's syntax, features, and how to use it to build robust and scalable applications.
TypeScript is a programming language that has gained popularity among developers in recent years. It is a superset of JavaScript, meaning that any valid JavaScript code is also valid TypeScript code. However, TypeScript offers additional features and benefits that make it a powerful tool for building complex applications.
One of the key features of TypeScript is its static typing system. Unlike JavaScript, where variables can hold any type of value, TypeScript allows developers to explicitly declare the type of a variable. This helps catch errors at compile-time rather than at runtime, making it easier to identify and fix bugs early in the development process.
In addition to static typing, TypeScript also introduces the concept of interfaces. An interface is a way to define the shape of an object, specifying the properties and methods it should have. This allows for better code organization and helps ensure that objects are used correctly throughout the application.
Another important feature of TypeScript is its support for classes and object-oriented programming (OOP) principles. With classes, developers can create blueprints for objects, defining their properties and behavior. This makes it easier to create reusable code and build complex applications with a clear structure.
TypeScript also includes support for modules, which allow developers to organize their code into separate files and reuse it across different parts of an application. This promotes code reusability and makes it easier to manage large codebases.
One of the main advantages of using TypeScript is its compatibility with existing JavaScript code. Since TypeScript is a superset of JavaScript, developers can gradually introduce TypeScript into their projects without having to rewrite all of their existing code. This makes it a great choice for teams that want to adopt TypeScript gradually or work on projects that already have a large codebase.
To start using TypeScript, developers need to install the TypeScript compiler, which can be done using npm, the package manager for JavaScript. Once installed, developers can use the compiler to transpile TypeScript code into JavaScript code that can be run in any browser or JavaScript runtime.
When writing TypeScript code, it is important to follow best practices and conventions. This includes using meaningful variable and function names, properly documenting the code, and organizing it into modules and classes. By following these practices, developers can write clean and maintainable code that is easier to understand and debug.
In conclusion, TypeScript is a powerful programming language that offers additional features and benefits over JavaScript. Its static typing system, support for interfaces and classes, and compatibility with existing JavaScript code make it a great choice for building complex applications. By following best practices and conventions, developers can take full advantage of TypeScript's capabilities and write clean and maintainable code. Whether you are a beginner or an experienced developer, learning TypeScript can greatly enhance your programming skills and open up new opportunities in the world of web development.
TypeScript is a programming language that has gained significant popularity among developers in recent years. It is a superset of JavaScript, meaning that any valid JavaScript code is also valid TypeScript code. However, TypeScript offers additional features and benefits that make it a powerful tool for building complex applications.
One of the key features of TypeScript is its static typing system. Unlike JavaScript, where variables can be of any type, TypeScript allows developers to specify the type of each variable. This helps catch errors at compile-time rather than at runtime, making it easier to identify and fix bugs early in the development process. Additionally, static typing provides better code documentation and improves code readability, as it clearly defines the expected types of variables and function parameters.
Another important feature of TypeScript is its support for object-oriented programming (OOP) concepts. TypeScript allows developers to define classes, interfaces, and inheritance relationships, making it easier to organize and structure code. This is particularly useful for large-scale projects where code modularity and reusability are crucial. By leveraging OOP principles, developers can create more maintainable and scalable applications.
TypeScript also introduces the concept of modules, which enable developers to organize their code into separate files and reuse them across different parts of an application. This promotes code modularity and separation of concerns, making it easier to manage and maintain complex projects. Modules can be imported and exported, allowing developers to encapsulate functionality and expose only what is necessary to other parts of the application.
One of the major benefits of using TypeScript is its compatibility with existing JavaScript libraries and frameworks. Since TypeScript is a superset of JavaScript, developers can seamlessly integrate existing JavaScript code into TypeScript projects. This means that developers can leverage the vast ecosystem of JavaScript libraries and frameworks while enjoying the additional features and benefits provided by TypeScript. This compatibility also makes it easier for developers to transition from JavaScript to TypeScript, as they can gradually introduce TypeScript into their existing codebase.
TypeScript also offers improved tooling and developer experience. It provides a rich set of development tools, including a powerful type checker and an intelligent code editor with features like autocompletion and code navigation. These tools help developers write cleaner and more reliable code, reducing the likelihood of errors and improving productivity. Additionally, TypeScript has excellent support in popular integrated development environments (IDEs) like Visual Studio Code, making it easy to set up and start developing with TypeScript.
In conclusion, TypeScript offers a range of key features and benefits that make it an attractive choice for developers. Its static typing system, support for object-oriented programming, and module system provide developers with powerful tools to build complex and maintainable applications. Furthermore, its compatibility with existing JavaScript code and improved tooling make it a practical choice for both new and existing projects. Whether you are a beginner or an experienced developer, TypeScript is definitely worth considering for your next project.
TypeScript is a powerful programming language that has gained popularity among developers in recent years. It is a superset of JavaScript, meaning that any valid JavaScript code is also valid TypeScript code. However, TypeScript offers additional features and benefits that make it a preferred choice for many developers.
If you are new to TypeScript and want to get started with TypeScript development, this article will provide you with a comprehensive introduction to the language and guide you through the initial steps of setting up your development environment.
To begin with, TypeScript offers static typing, which means that variables, function parameters, and return types can be explicitly declared with their respective types. This helps catch errors during the development process and improves code quality. Additionally, TypeScript supports the latest ECMAScript features, allowing developers to write modern JavaScript code while still targeting older browsers.
To start using TypeScript, you need to have Node.js installed on your machine. Node.js is a JavaScript runtime that allows you to run JavaScript code outside of a web browser. Once you have Node.js installed, you can use the Node Package Manager (npm) to install TypeScript globally on your system by running the command "npm install -g typescript" in your terminal or command prompt.
After installing TypeScript, you can create a new TypeScript project by running the command "tsc --init" in your project's root directory. This will generate a "tsconfig.json" file that contains the configuration settings for your TypeScript project. You can modify this file to customize the behavior of the TypeScript compiler according to your project's requirements.
Once you have set up your TypeScript project, you can start writing TypeScript code in ".ts" files. TypeScript files are similar to JavaScript files, but with the ".ts" extension. You can use any text editor or integrated development environment (IDE) of your choice to write TypeScript code.
One of the key features of TypeScript is its support for type annotations. Type annotations allow you to explicitly declare the types of variables, function parameters, and return values. This helps the TypeScript compiler catch type-related errors and provides better code documentation. For example, you can declare a variable of type "number" as follows: "let age: number = 25;". Similarly, you can declare a function with typed parameters and return type: "function add(a: number, b: number): number { return a + b; }".
TypeScript also supports interfaces, which allow you to define the structure of objects. Interfaces provide a way to enforce a specific shape for objects and ensure that they have the required properties and methods. You can define an interface using the "interface" keyword and then use it to type-check objects. For example, you can define an interface for a person object as follows: "interface Person { name: string; age: number; }". You can then use this interface to enforce the structure of person objects: "let person: Person = { name: 'John', age: 30 };".
In addition to type annotations and interfaces, TypeScript offers many other advanced features such as classes, modules, generics, and decorators. These features enable you to write more maintainable and scalable code, especially for larger projects.
In conclusion, TypeScript is a powerful programming language that offers static typing, modern JavaScript features, and additional benefits for developers. By following the initial steps outlined in this article, you can set up your TypeScript development environment and start writing TypeScript code. As you gain more experience with TypeScript, you can explore its advanced features and leverage its capabilities to build robust and efficient applications.
1. What is TypeScript?
TypeScript is a programming language developed by Microsoft that is a superset of JavaScript. It adds static typing and other features to JavaScript, making it easier to write and maintain large-scale applications.
2. What are the benefits of using TypeScript?
Some benefits of using TypeScript include improved code maintainability, enhanced tooling support, early error detection through static typing, better code organization with modules and classes, and the ability to leverage the latest JavaScript features while maintaining backward compatibility.
3. How does TypeScript differ from JavaScript?
TypeScript is a superset of JavaScript, meaning that any valid JavaScript code is also valid TypeScript code. However, TypeScript adds static typing, which allows for type checking during development and provides better tooling support. TypeScript also introduces features like interfaces, classes, modules, and decorators that are not present in JavaScript.
In conclusion, the Introduction to TypeScript for Beginners provides a comprehensive overview of TypeScript, a statically typed superset of JavaScript. The tutorial covers the basics of TypeScript, including its features, benefits, and how it can enhance JavaScript development. It also explains key concepts such as types, interfaces, classes, and modules, and provides practical examples to illustrate their usage. Overall, this introduction serves as a valuable resource for beginners looking to understand and start using TypeScript in their projects.