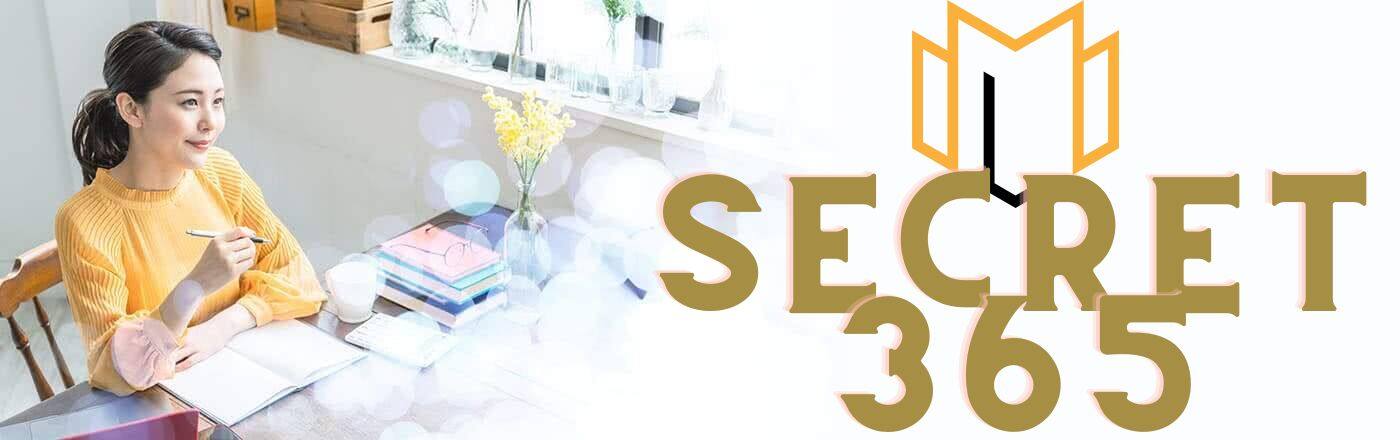
Exploring SQLite Database Navigation in Python: Enhancing Data Manipulation and Retrieval.
On Day 48 of the 100DaysOfCode challenge, we will be exploring SQLite database navigation in Python. SQLite is a lightweight and self-contained database engine that allows us to store and retrieve data efficiently. By learning how to navigate and interact with SQLite databases using Python, we can enhance our data manipulation and analysis skills. Let's dive into the world of SQLite database navigation in Python on this exciting day of coding!
Day 48 of 100DaysOfCode: Exploring SQLite Database Navigation in Python
Introduction to SQLite Database Navigation in Python
In today's article, we will delve into the world of SQLite database navigation in Python. SQLite is a lightweight, serverless, and self-contained database engine that is widely used in various applications. It offers a simple and efficient way to store and retrieve data, making it a popular choice among developers.
Before we dive into the details of SQLite database navigation, let's take a moment to understand what SQLite is and why it is so widely used. SQLite is a relational database management system that is embedded within the application itself. Unlike traditional database systems, SQLite does not require a separate server process to operate. This makes it easy to integrate into applications and eliminates the need for complex setup and administration.
To work with SQLite in Python, we need to install the sqlite3 module, which comes bundled with Python by default. This module provides a simple and intuitive interface to interact with SQLite databases. Once installed, we can start exploring the various functionalities it offers.
One of the fundamental operations in database navigation is establishing a connection to the database. In SQLite, we can create a connection object using the connect() function provided by the sqlite3 module. This function takes the name of the database file as a parameter and returns a connection object that we can use to interact with the database.
Once we have established a connection, we can execute SQL statements to perform various operations on the database. SQLite supports a wide range of SQL statements, including creating tables, inserting data, updating records, and querying data. We can execute these statements using the execute() method of the connection object.
To retrieve data from the database, we can use the fetchone() and fetchall() methods. The fetchone() method returns a single row from the result set, while the fetchall() method returns all the rows. We can iterate over the result set using a for loop to process each row individually.
In addition to executing SQL statements, SQLite also provides a way to work with transactions. A transaction is a sequence of database operations that are treated as a single unit of work. By using transactions, we can ensure that all the operations within a transaction are either completed successfully or rolled back if an error occurs.
To start a transaction, we can use the begin() method of the connection object. Once the transaction is started, we can execute multiple SQL statements and commit the changes using the commit() method. If an error occurs during the transaction, we can roll back the changes using the rollback() method.
In conclusion, SQLite database navigation in Python offers a powerful and efficient way to store and retrieve data. With its lightweight and serverless nature, SQLite is a popular choice among developers for various applications. By understanding the basics of SQLite database navigation, we can leverage its capabilities to build robust and scalable applications. In the next article, we will explore more advanced topics, such as working with multiple tables and performing complex queries. Stay tuned!
Day 48 of 100DaysOfCode: Exploring SQLite Database Navigation in Python
Understanding SQLite Database Queries in Python
In today's coding session, we delved into the world of SQLite database navigation in Python. SQLite is a lightweight, serverless database engine that allows us to store and retrieve data efficiently. With Python's built-in support for SQLite, we can easily interact with databases and perform various operations.
To begin our exploration, we first needed to establish a connection to an SQLite database. Python provides the sqlite3 module, which allows us to create a connection object using the connect() function. This connection object serves as our gateway to the database, enabling us to execute queries and retrieve results.
Once we established a connection, we could then execute SQL queries using the connection object's execute() method. This method takes a SQL statement as its parameter and executes it against the connected database. We can perform various operations such as creating tables, inserting data, updating records, and retrieving information.
To retrieve data from the database, we used the fetchall() method after executing a SELECT query. This method returns all the rows from the result set as a list of tuples. Each tuple represents a row, and we can access individual values using indexing. Alternatively, we can use the fetchone() method to retrieve a single row at a time.
To make our queries more dynamic, we can use placeholders in our SQL statements. These placeholders act as variables that we can substitute with actual values at runtime. Python's sqlite3 module provides a secure way to handle placeholders by passing a tuple of values as the second parameter to the execute() method. This prevents SQL injection attacks and ensures the integrity of our data.
In addition to placeholders, we can also use named parameters in our SQL statements. Named parameters allow us to specify values using a dictionary, where the keys correspond to the parameter names in the SQL statement. This provides more flexibility and readability, especially when dealing with complex queries.
To navigate through the result set, we can use the cursor object returned by the execute() method. The cursor object allows us to move the cursor position, fetch a specific number of rows, and iterate over the result set. This gives us fine-grained control over the data retrieval process and allows us to process large result sets efficiently.
Furthermore, we can use the executemany() method to execute the same SQL statement multiple times with different parameter values. This is particularly useful when inserting multiple rows into a table or updating multiple records simultaneously. By passing a list of tuples or dictionaries as the second parameter, we can perform batch operations efficiently.
In conclusion, exploring SQLite database navigation in Python has opened up a world of possibilities for us as developers. With Python's built-in support for SQLite, we can easily interact with databases, execute queries, and retrieve results. By understanding the various techniques and methods available to us, we can harness the power of SQLite and build robust applications that handle data efficiently. So, let's continue our coding journey and unlock the full potential of SQLite in Python.
Day 48 of 100DaysOfCode: Exploring SQLite Database Navigation in Python
In the world of programming, databases play a crucial role in storing and retrieving data efficiently. One popular database management system is SQLite, which is known for its simplicity and lightweight nature. In this article, we will delve into advanced techniques for navigating SQLite databases using Python.
To begin with, let's understand the basics of SQLite. SQLite is a serverless, self-contained, and zero-configuration database engine. It is widely used in various applications, including mobile apps and embedded systems. Python provides a built-in module called sqlite3, which allows us to interact with SQLite databases seamlessly.
The first step in working with SQLite databases is establishing a connection. We can achieve this by importing the sqlite3 module and using the connect() function. This function takes the name of the database file as an argument and returns a connection object. We can then use this object to execute SQL queries and perform various operations on the database.
Once we have established a connection, we can navigate through the database using SQL queries. The SELECT statement is commonly used to retrieve data from a database table. We can specify the columns we want to retrieve and the table we want to query. Additionally, we can use various clauses like WHERE, ORDER BY, and LIMIT to filter and sort the data.
In Python, we can execute SQL queries using the execute() method of the connection object. This method takes an SQL query as a parameter and returns a cursor object. The cursor object allows us to fetch the results of the query and iterate over them. We can use methods like fetchone(), fetchall(), and fetchmany() to retrieve the data from the cursor.
To illustrate this, let's consider an example where we have a table called "employees" with columns like "id", "name", and "salary". We can execute a SELECT query to retrieve all the employees' names and salaries. We can then use a loop to iterate over the cursor and print the results.
Moving on, we can also perform advanced navigation techniques like joining multiple tables. In SQLite, we can use the JOIN clause to combine rows from two or more tables based on a related column between them. This allows us to retrieve data from multiple tables in a single query.
In Python, we can execute JOIN queries using the execute() method just like any other SQL query. We can specify the tables we want to join and the columns we want to retrieve. We can also use various types of joins like INNER JOIN, LEFT JOIN, and RIGHT JOIN to control how the rows are combined.
Apart from querying data, we can also modify the database using SQL statements like INSERT, UPDATE, and DELETE. These statements allow us to add, update, and delete records in the database tables. We can execute these statements using the execute() method and commit the changes using the commit() method of the connection object.
In conclusion, navigating SQLite databases in Python is a powerful skill that allows us to retrieve and manipulate data efficiently. By understanding the basics of SQLite and using the sqlite3 module, we can execute SQL queries, perform advanced navigation techniques like joining tables, and modify the database using SQL statements. With these techniques in our arsenal, we can effectively work with SQLite databases and build robust applications.
1. What is the purpose of Day 48 of 100DaysOfCode?
The purpose of Day 48 is to explore SQLite database navigation in Python.
2. What specific topic is covered on Day 48?
On Day 48, the specific topic covered is navigating SQLite databases using Python.
3. What is the main goal of exploring SQLite database navigation in Python?
The main goal is to understand how to interact with SQLite databases using Python and navigate through the data stored within them.
On Day 48 of 100DaysOfCode, I explored SQLite database navigation in Python. I learned how to connect to a SQLite database, create tables, insert data, and perform various navigation operations such as selecting, updating, and deleting records. This knowledge will be valuable in developing applications that require database management and manipulation using SQLite in Python.