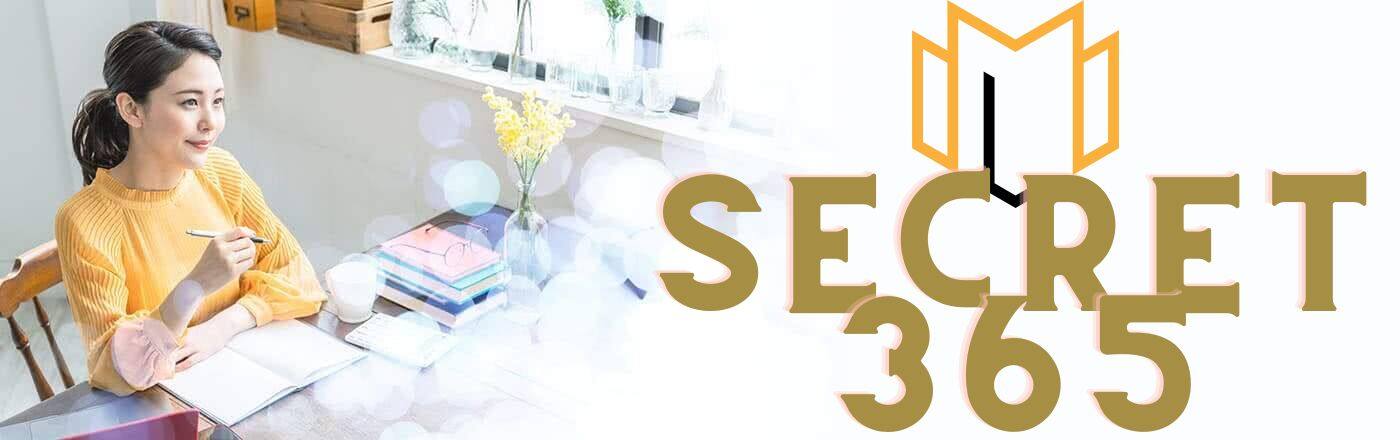
"Efficiently compress images with Python's power."
Python is a versatile programming language that can be utilized for a wide range of applications, including image compression. With its extensive libraries and powerful tools, Python provides an efficient and effective platform for developing an image compression tool. In this introduction, we will explore how Python can be utilized to create an image compression tool that optimizes file sizes without compromising image quality.
Image compression is a crucial aspect of modern technology, as it allows for the efficient storage and transmission of images. With the increasing popularity of social media platforms, websites, and mobile applications, the need for effective image compression techniques has become more important than ever. Python, a versatile and powerful programming language, offers a wide range of tools and libraries that can be utilized to develop an efficient image compression tool.
One of the most commonly used image compression techniques is the Discrete Cosine Transform (DCT). The DCT is a mathematical transformation that converts an image from the spatial domain to the frequency domain. By representing the image in terms of its frequency components, it becomes possible to discard high-frequency information that is less perceptually important. Python provides several libraries, such as NumPy and OpenCV, that offer functions for performing the DCT and inverse DCT operations.
Another popular image compression technique is the use of quantization. Quantization involves dividing the transformed image into a set of discrete levels. By reducing the number of levels, it is possible to discard some of the less important information, resulting in a compressed image. Python provides a range of functions and libraries that can be used to implement quantization algorithms, such as Lloyd-Max quantization or uniform quantization.
In addition to DCT and quantization, Python offers various other techniques that can be used to further enhance image compression. For example, wavelet-based compression techniques, such as the Discrete Wavelet Transform (DWT), can be implemented using Python libraries like PyWavelets. The DWT allows for a more localized representation of image features, which can lead to improved compression ratios.
Python also provides tools for implementing lossless compression techniques, which aim to preserve all the information in the original image. One such technique is Run-Length Encoding (RLE), which replaces consecutive repeated values with a count and a single instance of the value. Python's built-in functions, such as itertools.groupby, can be used to implement RLE efficiently.
Furthermore, Python offers a range of image processing libraries, such as PIL (Python Imaging Library) and scikit-image, which provide a wide range of functions for manipulating and processing images. These libraries can be used to perform pre-processing tasks, such as resizing or cropping images, before applying compression techniques.
In conclusion, Python is a powerful programming language that offers a wide range of tools and libraries for implementing image compression techniques. From the widely used DCT and quantization methods to more advanced techniques like wavelet-based compression, Python provides the necessary functions and libraries to develop an efficient image compression tool. Additionally, Python's image processing libraries offer a range of pre-processing functions that can be used to enhance the compression process. By utilizing Python for image compression, developers can create effective and efficient solutions for storing and transmitting images in various applications.
Utilize Python for our Image Compression Tool
In today's digital age, where images play a crucial role in various industries, having an efficient image compression tool is essential. Image compression allows us to reduce the file size of an image without significantly compromising its quality. This not only helps in saving storage space but also enables faster image loading on websites and applications. In this step-by-step guide, we will explore how to build an image compression tool using Python, a versatile and powerful programming language.
Step 1: Installing the Required Libraries
To begin, we need to install the necessary libraries that will enable us to work with images in Python. Two popular libraries for image processing are Pillow and OpenCV. Pillow provides a user-friendly interface for image manipulation, while OpenCV offers advanced image processing capabilities. We can install these libraries using pip, the package installer for Python.
Step 2: Loading and Displaying an Image
Once we have the required libraries installed, we can proceed to load and display an image using Python. We can use the Image module from the Pillow library to open an image file and display it on the screen. This step is crucial as it allows us to visualize the image before and after compression.
Step 3: Understanding Image Compression Techniques
Before diving into the implementation, it is essential to understand the different image compression techniques available. Lossless compression algorithms preserve all the original image data, while lossy compression algorithms discard some data to achieve higher compression ratios. Depending on the specific requirements of our project, we can choose the appropriate compression technique.
Step 4: Implementing Lossless Compression
Lossless compression is ideal when we need to preserve all the image data without any loss in quality. One popular lossless compression algorithm is the Run-Length Encoding (RLE). RLE works by replacing consecutive repeated pixels with a count and the pixel value. We can implement this algorithm using Python to compress our images.
Step 5: Implementing Lossy Compression
If our primary concern is achieving higher compression ratios, we can opt for lossy compression techniques. One widely used lossy compression algorithm is the Discrete Cosine Transform (DCT) combined with Quantization. DCT converts the image from the spatial domain to the frequency domain, allowing us to discard high-frequency components. Quantization further reduces the precision of the remaining coefficients. By implementing these techniques in Python, we can achieve significant compression while maintaining acceptable image quality.
Step 6: Comparing Compression Results
After implementing both lossless and lossy compression techniques, it is crucial to compare the results. We can measure the compression ratio, which is the ratio of the original image size to the compressed image size. Additionally, we can calculate the Peak Signal-to-Noise Ratio (PSNR) to evaluate the quality of the compressed image compared to the original. These metrics will help us determine the effectiveness of our image compression tool.
Step 7: Optimizing the Compression Tool
To further enhance our image compression tool, we can explore additional techniques such as Huffman coding, which assigns shorter codes to frequently occurring pixels. This optimization can significantly improve the compression ratio. Additionally, we can experiment with different compression parameters and algorithms to find the optimal balance between compression and image quality.
In conclusion, building an image compression tool using Python can be a rewarding endeavor. By following this step-by-step guide, we can harness the power of Python libraries such as Pillow and OpenCV to implement both lossless and lossy compression techniques. With the ability to visualize and compare compression results, we can fine-tune our tool for optimal performance. Whether it is for web development, mobile applications, or any other image-intensive project, Python provides a versatile and efficient solution for image compression.
Utilizing Python for our Image Compression Tool
In today's digital age, where images play a crucial role in various industries, optimizing image compression efficiency has become a necessity. With the increasing demand for faster loading times and reduced storage space, finding ways to compress images without compromising their quality is essential. Python, a versatile programming language, offers a range of tools and libraries that can help achieve this goal.
One of the most popular libraries for image compression in Python is Pillow. Pillow provides a simple and intuitive interface for manipulating images, including compression. By using Pillow, developers can easily implement image compression algorithms and techniques to reduce file sizes while maintaining acceptable image quality.
One of the key advantages of using Python for image compression is its extensive library ecosystem. Apart from Pillow, there are several other libraries available that can be used in conjunction with Pillow to enhance the compression process. For example, NumPy, a powerful numerical computing library, can be used to perform complex mathematical operations on images, such as transforming them into arrays and applying compression algorithms.
Another useful library for image compression in Python is scikit-image. Scikit-image provides a collection of algorithms for image processing, including compression techniques. By leveraging the capabilities of scikit-image, developers can experiment with different compression algorithms and find the most suitable one for their specific needs.
Python's flexibility also allows developers to integrate image compression into existing workflows seamlessly. Whether it's a web application, a desktop software, or a command-line tool, Python can be easily integrated into the development process. This means that developers can incorporate image compression into their existing projects without having to start from scratch.
Furthermore, Python's readability and simplicity make it an ideal choice for beginners who want to learn about image compression. The language's syntax is easy to understand, and there are plenty of resources available online to help newcomers get started. This accessibility makes Python a great tool for educational purposes, allowing students and enthusiasts to explore the world of image compression without feeling overwhelmed.
When it comes to optimizing image compression efficiency, Python offers several tips and tricks that can significantly improve the process. One such tip is to leverage parallel processing using Python's multiprocessing module. By distributing the compression workload across multiple cores or processors, developers can achieve faster compression times and improve overall efficiency.
Another useful trick is to utilize Python's built-in data structures, such as dictionaries and sets, to store and manipulate image data efficiently. These data structures provide fast lookup and retrieval times, which can be beneficial when working with large image datasets.
Additionally, developers can take advantage of Python's memory management capabilities to optimize image compression. By using techniques like memory mapping and lazy loading, developers can reduce memory usage and improve the overall performance of the compression process.
In conclusion, Python is a powerful tool for optimizing image compression efficiency. With its extensive library ecosystem, flexibility, and simplicity, Python provides developers with the necessary tools and resources to implement effective image compression algorithms. By leveraging Python's capabilities and following the tips and tricks mentioned above, developers can achieve faster loading times, reduced storage space, and improved image quality in their applications.
1. How can Python be utilized for image compression?
Python can be used for image compression by implementing various algorithms such as JPEG, PNG, or other compression techniques. Python libraries like Pillow or OpenCV can be used to read, manipulate, and compress images.
2. What are the advantages of using Python for image compression?
Python offers a wide range of libraries and tools specifically designed for image processing and compression. It provides a simple and intuitive syntax, making it easier to implement complex algorithms. Additionally, Python's extensive community support ensures access to numerous resources and tutorials.
3. Are there any limitations to using Python for image compression?
While Python is a versatile language for image compression, it may not be as efficient as lower-level languages like C or C++. This can result in slower execution times for large-scale image compression tasks. However, Python's ease of use and extensive library support often outweigh these limitations for many applications.
In conclusion, utilizing Python for our image compression tool offers several advantages. Python provides a wide range of libraries and frameworks specifically designed for image processing and compression, such as Pillow and OpenCV. These libraries offer various algorithms and techniques to efficiently compress images while maintaining acceptable image quality. Additionally, Python's simplicity and readability make it easier to develop and maintain the image compression tool. Overall, utilizing Python for our image compression tool would enable us to create a robust and efficient solution for reducing image file sizes without significant loss of visual quality.