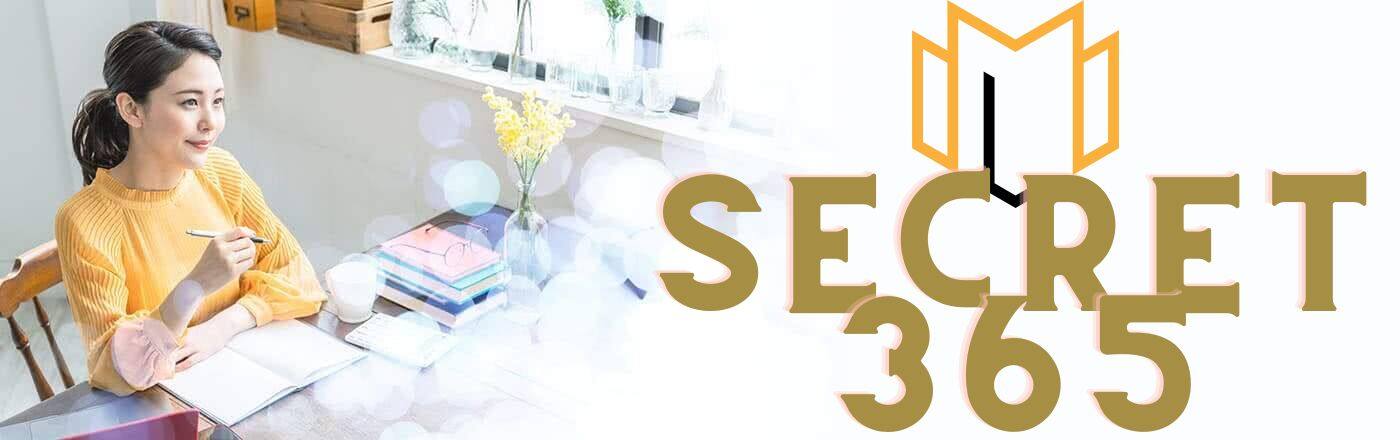
Advanced techniques for mastering the Builder Pattern in software development.
In Part 7 of our series on Mastering the Builder Pattern in Software Development, we will delve deeper into advanced techniques and best practices for implementing the Builder Pattern. We will explore topics such as handling complex object creation, managing optional parameters, and creating fluent interfaces. By the end of this article, you will have a comprehensive understanding of how to effectively utilize the Builder Pattern to enhance the flexibility and maintainability of your software projects. Let's dive in!
The Builder pattern is a widely used design pattern in software development that allows for the creation of complex objects step by step. In the previous articles of this series, we have discussed the basics of the Builder pattern and its benefits. In this article, we will focus on implementing the Builder pattern in object-oriented programming languages.
Object-oriented programming languages, such as Java, C++, and Python, provide the necessary features to implement the Builder pattern effectively. The key idea behind implementing the Builder pattern in these languages is to use a separate builder class to construct the complex object.
To implement the Builder pattern, we first need to define the class for the complex object we want to create. This class should have all the necessary attributes and methods to represent the object. However, instead of providing a public constructor, we make the constructor private or protected to prevent direct instantiation of the object.
Next, we create a separate builder class that is responsible for constructing the complex object. The builder class should have methods to set the values of the attributes of the complex object. These methods can be named in a way that is intuitive and easy to understand, such as `setFirstName()`, `setLastName()`, `setAge()`, etc.
The builder class should also have a method to build and return the final complex object. This method, commonly named `build()`, should instantiate the complex object using the values set by the builder methods and return it.
To make the implementation of the Builder pattern more convenient, we can use method chaining. Method chaining allows us to call multiple methods on an object in a single line of code. This can be achieved by returning the builder object itself from each method in the builder class.
For example, let's say we have a complex object called `Person` with attributes `firstName`, `lastName`, and `age`. We can implement the builder class as follows:
```
public class PersonBuilder {
private String firstName;
private String lastName;
private int age;
public PersonBuilder setFirstName(String firstName) {
this.firstName = firstName;
return this;
}
public PersonBuilder setLastName(String lastName) {
this.lastName = lastName;
return this;
}
public PersonBuilder setAge(int age) {
this.age = age;
return this;
}
public Person build() {
return new Person(firstName, lastName, age);
}
}
```
With this implementation, we can create a `Person` object using the builder as follows:
```
Person person = new PersonBuilder()
.setFirstName("John")
.setLastName("Doe")
.setAge(30)
.build();
```
This approach provides a clean and readable way to construct complex objects with many attributes. It also allows for flexibility in setting only the necessary attributes and provides a clear separation between the construction logic and the complex object itself.
In conclusion, implementing the Builder pattern in object-oriented programming languages involves creating a separate builder class that is responsible for constructing the complex object. This approach provides a convenient and flexible way to create complex objects step by step. By using method chaining, we can achieve a clean and readable code that separates the construction logic from the complex object.
The Builder pattern is a widely used design pattern in software development that allows for the creation of complex objects step by step. In large-scale software projects, where there are multiple components and dependencies, utilizing the Builder pattern effectively becomes crucial. In this article, we will discuss some best practices for utilizing the Builder pattern in large-scale software projects.
One of the first best practices is to define a clear and consistent naming convention for the Builder classes and methods. This helps in maintaining a standardized codebase and makes it easier for developers to understand and work with the code. For example, using names like "Director" for the class that controls the construction process and "Builder" for the class that actually builds the object can provide clarity and improve code readability.
Another important best practice is to ensure that the Builder pattern is used only when necessary. While the Builder pattern can be useful in creating complex objects, it may not be the best choice for simpler objects. It is important to evaluate the complexity and dependencies of the object being created before deciding to use the Builder pattern. If the object can be created easily without the need for a step-by-step construction process, using simpler object creation techniques like constructors or factory methods may be more appropriate.
In large-scale software projects, it is common to have multiple variations of an object that need to be created. In such cases, it is recommended to create separate Builder classes for each variation. This helps in keeping the code modular and makes it easier to add or modify variations in the future. Each Builder class can have its own set of methods for constructing the specific variation of the object, providing flexibility and maintainability.
To further enhance the flexibility of the Builder pattern in large-scale software projects, it is advisable to use interfaces or abstract classes for the Builder and Product classes. This allows for easy substitution of different implementations of the Builder and Product classes, making the code more adaptable to changes. By programming to interfaces, rather than concrete classes, the code becomes more loosely coupled and easier to test and maintain.
In large-scale software projects, it is common to have complex object hierarchies with multiple levels of inheritance. In such cases, it is important to ensure that the Builder pattern is implemented consistently across all levels of the hierarchy. This helps in maintaining a uniform construction process and avoids any inconsistencies or errors in the object creation. It is also recommended to use inheritance in the Builder classes to reuse common construction logic and avoid code duplication.
Lastly, it is crucial to thoroughly test the Builder pattern implementation in large-scale software projects. This includes testing the construction process, verifying the correctness of the created objects, and ensuring that the Builder classes handle all possible variations and edge cases correctly. Automated unit tests can be written to validate the behavior of the Builder classes and catch any potential issues early in the development cycle.
In conclusion, utilizing the Builder pattern effectively in large-scale software projects requires following some best practices. These include defining a clear naming convention, using the pattern only when necessary, creating separate Builder classes for variations, using interfaces or abstract classes, implementing consistently across object hierarchies, and thoroughly testing the implementation. By adhering to these best practices, developers can master the Builder pattern and leverage its benefits in creating complex objects in large-scale software projects.
The Builder pattern is a widely used design pattern in software development that allows for the creation of complex objects step by step. It provides a clear and concise way to construct objects, making the code more readable and maintainable. In this article, we will explore advanced techniques for customizing the Builder pattern in software development.
One of the key advantages of the Builder pattern is its flexibility. It allows developers to customize the construction process of objects by adding additional methods to the builder class. These methods can be used to set specific attributes or perform additional operations during the object creation process.
One advanced technique for customizing the Builder pattern is the use of method chaining. Method chaining allows for a more fluent and expressive way of constructing objects. By returning the builder instance after each method call, developers can chain multiple method calls together, resulting in a more concise and readable code.
Another technique for customizing the Builder pattern is the use of nested builders. Nested builders allow for the construction of complex objects with multiple levels of nesting. This technique is particularly useful when dealing with objects that have hierarchical structures or contain other objects as attributes.
In addition to method chaining and nested builders, another advanced technique for customizing the Builder pattern is the use of default values. Default values can be set for attributes that are not explicitly set by the client code. This ensures that the object being constructed always has valid values for all its attributes, even if they are not explicitly set.
Furthermore, the Builder pattern can be customized to handle optional attributes. Optional attributes are attributes that may or may not be set by the client code. By providing methods to set optional attributes, developers can make the construction process more flexible and allow for the creation of objects with varying sets of attributes.
The use of generics is another advanced technique for customizing the Builder pattern. Generics allow for the creation of builders that can be used to construct objects of different types. This provides a more generic and reusable solution, as the same builder class can be used to construct different types of objects.
Lastly, the Builder pattern can be customized to handle validation and error handling. By adding validation logic to the builder class, developers can ensure that the object being constructed is valid and meets certain criteria. Error handling can also be added to handle exceptional cases during the construction process.
In conclusion, the Builder pattern is a powerful design pattern in software development that allows for the creation of complex objects step by step. By customizing the Builder pattern using advanced techniques such as method chaining, nested builders, default values, optional attributes, generics, and validation/error handling, developers can create more flexible and customizable object construction processes. These techniques enhance the readability, maintainability, and reusability of the code, making the Builder pattern an essential tool in the software developer's toolkit.
1. What is the purpose of the Builder pattern in software development?
The Builder pattern is used to create complex objects step by step, allowing the construction process to be separated from the object's representation.
2. How does the Builder pattern differ from other creational patterns?
Unlike other creational patterns like the Factory pattern, the Builder pattern focuses on constructing complex objects by breaking down the construction process into smaller steps.
3. What are the advantages of using the Builder pattern?
Using the Builder pattern can improve code readability, allow for the creation of different object representations using the same construction process, and provide better control over the construction of complex objects.
In conclusion, Part 7 of Mastering the Builder Pattern in Software Development provides a comprehensive understanding of the various aspects and implementation techniques of the Builder pattern. It covers advanced topics such as nested builders, fluent interfaces, and customization options, enabling developers to create flexible and maintainable code. By mastering the Builder pattern, software developers can enhance their design skills and improve the overall quality and efficiency of their software projects.