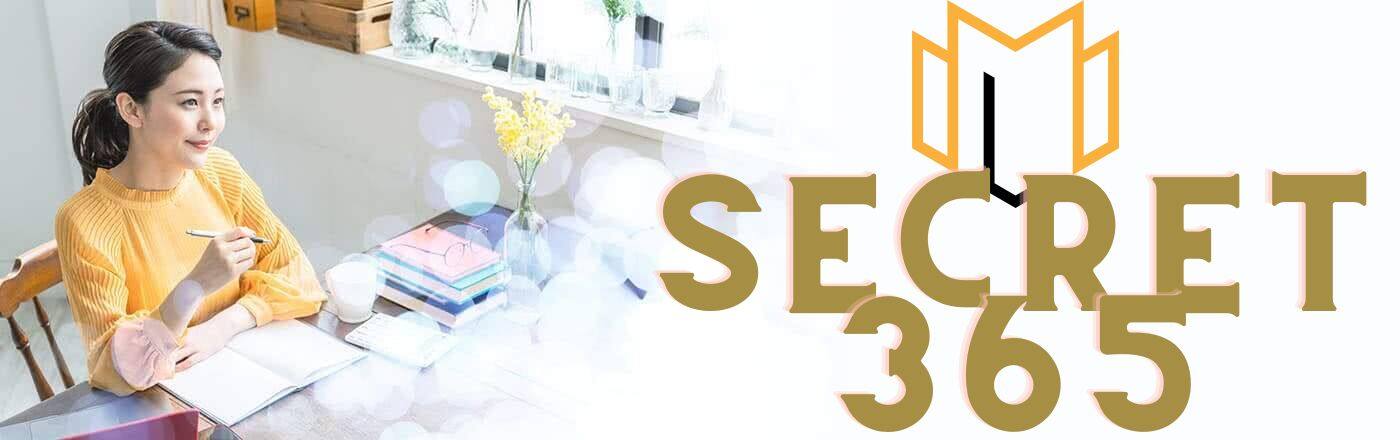
Unleash the Power of Rust Enums: Exploring Limitless Possibilities
Rust is a modern programming language that offers a powerful feature called enums. Enums in Rust provide a way to define a type by enumerating its possible values. This versatility of Rust enums allows developers to handle complex scenarios and create more expressive and concise code. In this article, we will explore the various ways in which Rust enums can be used, highlighting their flexibility and usefulness in different programming tasks.
Rust is a powerful programming language that offers a wide range of features to developers. One of these features is the ability to use enums, or enumerations, which provide a way to define a type by enumerating its possible values. Enums in Rust are incredibly versatile and can be used in a variety of ways to enhance the functionality and readability of your code.
At its core, an enum in Rust is a type that can have a fixed set of values. These values are called variants, and each variant can have associated data. Enums are defined using the `enum` keyword, followed by the name of the enum and a list of its variants. Each variant can optionally have associated data, which can be of any type.
One of the most common use cases for enums is to represent a set of related values. For example, you could define an enum called `Color` with variants for different colors like `Red`, `Green`, and `Blue`. This allows you to create variables of type `Color` and assign them one of the defined variants. Enums make it easy to work with a limited set of values and ensure that only valid values are used.
Enums can also be used to define complex data structures. For example, you could define an enum called `Shape` with variants for different shapes like `Circle`, `Rectangle`, and `Triangle`. Each variant can have associated data, such as the radius for a circle or the width and height for a rectangle. This allows you to create variables of type `Shape` and easily access the associated data for each variant.
Another powerful feature of enums in Rust is pattern matching. Pattern matching allows you to match against the different variants of an enum and execute different code based on the matched variant. This can be incredibly useful for handling different cases or implementing different behaviors based on the value of an enum. Pattern matching in Rust is exhaustive, meaning that you must handle all possible variants of an enum, ensuring that your code is robust and error-free.
Enums can also be used to define error types. Rust encourages the use of the `Result` type for error handling, which is an enum with variants for `Ok` and `Err`. The `Ok` variant represents a successful result, while the `Err` variant represents an error. This allows you to handle errors in a structured and consistent way, making your code more reliable and easier to maintain.
In addition to these basic use cases, enums in Rust can be combined with other language features to create even more powerful constructs. For example, you can use enums with generics to create generic data structures that can work with different types. You can also use enums with traits to define behavior that can be implemented by different types. These advanced use cases allow you to write flexible and reusable code that can adapt to different scenarios.
In conclusion, enums in Rust are a versatile feature that can be used in a variety of ways to enhance your code. Whether you need to represent a set of related values, define complex data structures, handle errors, or create advanced constructs, enums provide a powerful and flexible solution. By understanding the basics of Rust enums and exploring their various use cases, you can take full advantage of this feature and write more robust and maintainable code.
Exploring the Versatility of Rust Enums
Rust is a powerful programming language that offers a wide range of features to developers. One of the most interesting and versatile features of Rust is its support for enums. Enums, short for enumerations, allow developers to define a type that can have a fixed set of values. This article will explore the advanced features of Rust enums and how they can be used to write more expressive and efficient code.
One of the key advantages of using enums in Rust is their ability to represent a set of related values. This makes them particularly useful when dealing with situations where a variable can have multiple possible states. For example, consider a program that models the state of a game. The game can be in one of three states: "playing", "paused", or "game over". By defining an enum with these three states, developers can ensure that the game state is always valid and can easily handle different scenarios based on the current state.
Enums in Rust can also have associated values, which further enhances their versatility. Associated values allow developers to attach additional data to each variant of an enum. This can be useful in situations where different variants of an enum require different types of data. For instance, consider an enum that represents different types of shapes. Each variant of the enum can have associated values that represent the specific properties of that shape, such as its size or color. This allows developers to work with different shapes in a more flexible and concise manner.
Another powerful feature of Rust enums is pattern matching. Pattern matching allows developers to match against the different variants of an enum and execute specific code based on the matched variant. This can be particularly useful when dealing with complex data structures or handling different cases in a program. For example, consider a program that processes different types of events. By using pattern matching on an enum that represents these events, developers can easily handle each event type separately and write more concise and readable code.
Rust enums also support the use of generics, which adds another layer of flexibility to their usage. Generics allow developers to write code that can work with different types without sacrificing type safety. By using generics with enums, developers can create more reusable and generic code that can handle a wide range of scenarios. For example, consider an enum that represents different types of containers. By using generics, developers can write code that can work with any type of container, such as vectors or linked lists, without having to write separate code for each type.
In conclusion, Rust enums are a powerful and versatile feature that can greatly enhance the expressiveness and efficiency of code. They allow developers to represent a set of related values, attach associated values, and use pattern matching to handle different cases. Additionally, the support for generics adds another layer of flexibility to their usage. By leveraging these advanced features of Rust enums, developers can write more expressive, concise, and reusable code. So, the next time you find yourself in a situation where you need to represent a fixed set of values or handle different cases, consider using Rust enums to make your code more robust and efficient.
Rust is a powerful programming language that offers a wide range of features to developers. One of the most versatile features of Rust is its support for enums. Enums, short for enumerations, allow developers to define a type by enumerating its possible values. This article will explore the practical examples of utilizing Rust enums in real-world applications.
One common use case for Rust enums is representing the state of an object or a system. For example, imagine a simple traffic light system. The traffic light can be in one of three states: red, yellow, or green. In Rust, we can define an enum called "TrafficLight" with three variants: Red, Yellow, and Green. This allows us to easily represent and manipulate the state of the traffic light in our code.
Enums can also be used to represent different types of errors in a program. In Rust, error handling is an important aspect of writing robust and reliable code. By using enums, we can define a custom error type that can represent different kinds of errors that may occur during the execution of our program. This makes it easier to handle and propagate errors in a consistent and structured manner.
Another practical example of utilizing Rust enums is in parsing and processing data. Let's say we have a CSV file containing information about employees in a company. Each line in the file represents a different employee, and each field is separated by a comma. In Rust, we can define an enum called "Employee" with different variants representing the different fields in the CSV file. This allows us to easily parse and process the data, as we can pattern match on the enum variants to extract the relevant information.
Enums can also be used to represent different options or choices in a program. For example, imagine a command-line tool that allows users to perform different operations on a file. The tool can have different modes of operation, such as "read", "write", or "delete". In Rust, we can define an enum called "Operation" with different variants representing the different modes of operation. This allows us to easily handle and process the user's choice in a structured and type-safe manner.
In addition to these practical examples, Rust enums offer many other possibilities for developers. They can be used to represent different types of data structures, such as linked lists or binary trees. They can also be used to define custom iterators or state machines. The versatility of Rust enums is truly remarkable, and it is one of the reasons why Rust is gaining popularity among developers.
In conclusion, Rust enums are a powerful feature that can be utilized in a wide range of real-world applications. Whether it is representing the state of an object, handling errors, parsing data, or making choices, enums provide a structured and type-safe way to model and manipulate data in Rust. By understanding and harnessing the power of enums, developers can write more robust, reliable, and maintainable code in Rust.
1. What is a Rust enum?
A Rust enum is a data type that allows for the definition of a type that can have a fixed set of values, known as variants.
2. How can Rust enums be used to explore versatility?
Rust enums can be used to represent different states or options within a program, allowing for more expressive and concise code. They can also be used in pattern matching to handle different cases or behaviors.
3. What are some advantages of using Rust enums?
Using Rust enums can help prevent bugs by enforcing exhaustive handling of all possible cases. They also provide a clear and self-documenting way to represent different options or states, making the code more readable and maintainable.
In conclusion, exploring the versatility of Rust enums allows developers to create powerful and expressive data structures. Rust enums provide a flexible way to define a type that can have multiple variants, each with its own set of associated data. This versatility enables developers to handle complex scenarios and improve code readability and maintainability. By leveraging pattern matching and exhaustive matching, Rust enums offer a robust mechanism for handling different cases and ensuring code correctness. Overall, understanding and utilizing the versatility of Rust enums can greatly enhance the development experience and enable the creation of more efficient and reliable software.