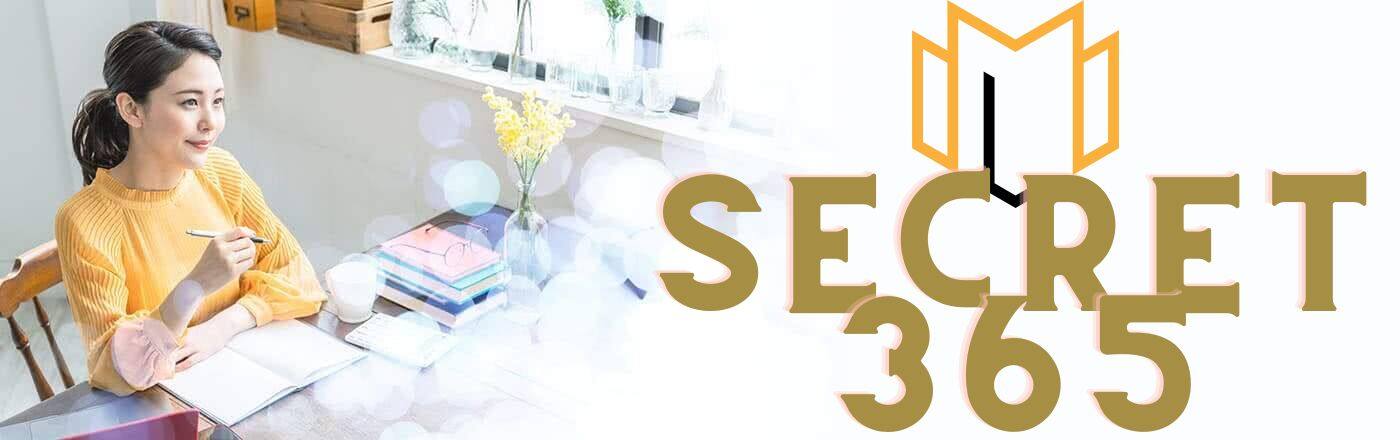
"Master React Development: Avoid Common Mistakes, Build Flawless Apps"
In React development, it is important to be aware of and avoid common mistakes that can hinder the efficiency and effectiveness of your code. By understanding these mistakes and taking proactive measures to avoid them, you can ensure a smoother development process and improve the overall quality of your React applications. In this article, we will discuss some of the most common mistakes in React development and provide tips on how to avoid them.
React is a popular JavaScript library that allows developers to build user interfaces efficiently. However, like any other technology, it is not without its challenges. One area where developers often make mistakes is in the structure of their React components. In this article, we will discuss some best practices for organizing and structuring React components to avoid common mistakes.
One common mistake that developers make is creating components that are too large and do too many things. This can make the code difficult to understand and maintain. Instead, it is recommended to break down components into smaller, reusable pieces. This not only makes the code more modular but also improves reusability and testability.
Another mistake to avoid is having too many levels of nesting in component structure. While nesting can be useful for organizing code, excessive nesting can lead to a complex and hard-to-maintain codebase. It is important to strike a balance between nesting and simplicity. If a component becomes too deeply nested, it may be a sign that it needs to be broken down into smaller components.
Furthermore, it is important to follow a consistent naming convention for components. This makes it easier for other developers to understand the codebase and reduces confusion. A good practice is to use descriptive and meaningful names that accurately represent the purpose of the component. Additionally, it is recommended to use PascalCase for component names to distinguish them from regular HTML elements.
Separating concerns is another best practice for structuring React components. It is important to keep the presentation logic separate from the business logic. This can be achieved by using container components and presentational components. Container components handle the data and state management, while presentational components focus on rendering the UI. This separation of concerns improves code maintainability and reusability.
In addition to separating concerns, it is also important to keep the component's state as minimal as possible. The state should only contain data that is necessary for the component to render and function correctly. Keeping the state minimal reduces complexity and makes it easier to reason about the component's behavior. If a piece of data is not needed for rendering or functionality, it is better to store it in a parent component or a global state management solution like Redux.
Another best practice is to avoid using inline styles in components. While inline styles may seem convenient, they can quickly become difficult to manage and maintain as the codebase grows. Instead, it is recommended to use CSS-in-JS libraries like styled-components or CSS modules. These libraries allow you to write CSS styles in JavaScript, making it easier to manage styles and keep them separate from the component logic.
Lastly, it is important to consider performance when structuring React components. One common mistake is re-rendering components unnecessarily. React provides lifecycle methods like shouldComponentUpdate and PureComponent to optimize rendering. By implementing these methods and using memoization techniques, you can prevent unnecessary re-renders and improve performance.
In conclusion, structuring React components properly is crucial for building maintainable and efficient applications. By following best practices such as breaking down components, avoiding excessive nesting, using consistent naming conventions, separating concerns, keeping state minimal, avoiding inline styles, and optimizing performance, developers can avoid common mistakes and create high-quality React applications.
Effective State Management in React Applications
React is a popular JavaScript library used for building user interfaces. One of the key aspects of React development is state management. State refers to the data that determines how a component renders and behaves. Managing state effectively is crucial for creating robust and efficient React applications. In this article, we will discuss some common mistakes in state management and provide tips on how to avoid them.
One common mistake in React development is directly mutating the state. React components are designed to be immutable, meaning that their state should not be modified directly. Modifying the state directly can lead to unexpected behavior and make it difficult to track changes. Instead, it is recommended to use the `setState` method provided by React to update the state. This ensures that React can properly handle the state updates and trigger re-renders as needed.
Another mistake is not considering the performance implications of state updates. React components re-render whenever their state or props change. If the state is updated frequently, it can lead to unnecessary re-renders and impact the performance of the application. To avoid this, it is important to optimize state updates by batching them together when possible. This can be done by using the `setState` method with a callback function, which allows multiple state updates to be applied in a single batch.
In addition, it is important to avoid unnecessary re-renders by properly managing the component's lifecycle. React provides lifecycle methods that allow developers to control when a component should update. By implementing shouldComponentUpdate or using React's PureComponent, unnecessary re-renders can be prevented. This can greatly improve the performance of the application, especially for components with complex rendering logic.
Another mistake to avoid is tightly coupling state management with component logic. It is common for developers to store all the state within a single component, leading to a large and complex component. This can make the code difficult to maintain and test. Instead, it is recommended to separate the state into smaller, more manageable pieces. This can be achieved by using React's context API or by using state management libraries like Redux or MobX. By decoupling the state from the component logic, it becomes easier to reason about the application's state and make changes without affecting other parts of the code.
Furthermore, it is important to handle asynchronous state updates correctly. React provides lifecycle methods like componentDidMount and componentDidUpdate to handle side effects and asynchronous operations. It is important to properly handle these operations to avoid race conditions and ensure that the component's state is updated correctly. Using promises or async/await syntax can help simplify asynchronous state updates and make the code more readable.
Lastly, it is crucial to properly test the state management logic in React applications. Testing helps ensure that the state is updated correctly and that the component behaves as expected. Unit tests can be written to cover different scenarios and edge cases. Additionally, integration tests can be used to test the interaction between components and their state. By writing comprehensive tests, developers can catch bugs early and maintain the stability of the application.
In conclusion, effective state management is essential for building robust and efficient React applications. By avoiding common mistakes like directly mutating the state, optimizing state updates, decoupling state management from component logic, handling asynchronous updates correctly, and properly testing the state management logic, developers can create high-quality React applications that are easy to maintain and scale.
Optimizing Performance in React Development
React has become one of the most popular JavaScript libraries for building user interfaces. Its component-based architecture and virtual DOM make it efficient and flexible. However, like any technology, there are common mistakes that developers can make when working with React that can negatively impact performance. In this article, we will explore some of these mistakes and provide tips on how to avoid them.
One common mistake is not properly managing state in React components. State is an important concept in React, as it allows components to store and manage data that can change over time. However, if state is not managed correctly, it can lead to unnecessary re-renders and decreased performance.
To avoid this mistake, it is important to only store necessary data in state and to update it efficiently. Avoid storing large amounts of data in state that is not needed for rendering. Additionally, use the shouldComponentUpdate lifecycle method or React's PureComponent to prevent unnecessary re-renders when state or props haven't changed.
Another mistake that can impact performance is not optimizing the rendering of lists in React. When rendering a list of items, it is common to use the map function to iterate over the data and create a component for each item. However, if the list is large, this can lead to performance issues.
To optimize the rendering of lists, use the key prop when rendering components in a loop. The key prop helps React identify which components have changed, allowing it to update only the necessary components instead of re-rendering the entire list. Additionally, consider using libraries like react-virtualized or react-window to efficiently render large lists by only rendering the visible items.
Improperly handling events can also impact performance in React. When handling events, it is important to avoid creating new functions on every render, as this can lead to unnecessary re-renders and decreased performance.
To handle events efficiently, use the useCallback hook or bind event handlers in the constructor to prevent unnecessary function creations. This ensures that the same function instance is used across renders, reducing the number of re-renders and improving performance.
Lastly, not properly optimizing the size of React bundles can also impact performance. When building a React application, it is common to use tools like webpack or Parcel to bundle the code. However, if the bundle size is too large, it can lead to longer load times and decreased performance.
To optimize bundle size, consider using code splitting techniques to split the code into smaller chunks that can be loaded on-demand. This can be done using dynamic imports or tools like React.lazy and Suspense. Additionally, minify and compress the bundled code to reduce its size further.
In conclusion, optimizing performance in React development requires avoiding common mistakes that can negatively impact the user experience. Properly managing state, optimizing the rendering of lists, handling events efficiently, and optimizing bundle size are all important considerations. By following these tips, developers can ensure that their React applications are fast and responsive, providing a great user experience.
1. What are some common mistakes to avoid in React development?
- Not using proper state management techniques
- Overcomplicating component hierarchy
- Neglecting to optimize performance
- Failing to handle errors and edge cases properly
2. How can I avoid overcomplicating component hierarchy in React?
- Keep components small and focused on a single responsibility
- Use composition and reusable components to avoid nesting too many levels deep
- Utilize React's context API or state management libraries to share state between components efficiently
3. What are some best practices for optimizing performance in React?
- Use React's memoization techniques like React.memo and useMemo to prevent unnecessary re-renders
- Implement lazy loading and code splitting to load components and resources only when needed
- Optimize network requests by reducing unnecessary API calls and using caching strategies
- Use performance profiling tools like React DevTools to identify and address performance bottlenecks.
In conclusion, avoiding common mistakes in React development is crucial for building efficient and maintainable applications. By following best practices such as proper component structure, state management, and code organization, developers can minimize bugs, improve performance, and enhance the overall user experience. Additionally, staying updated with the latest React features and tools, as well as seeking feedback from peers, can help developers identify and rectify any potential mistakes early on in the development process. Ultimately, by being mindful of these common mistakes and continuously improving their skills, React developers can create high-quality applications that meet the needs of their users.