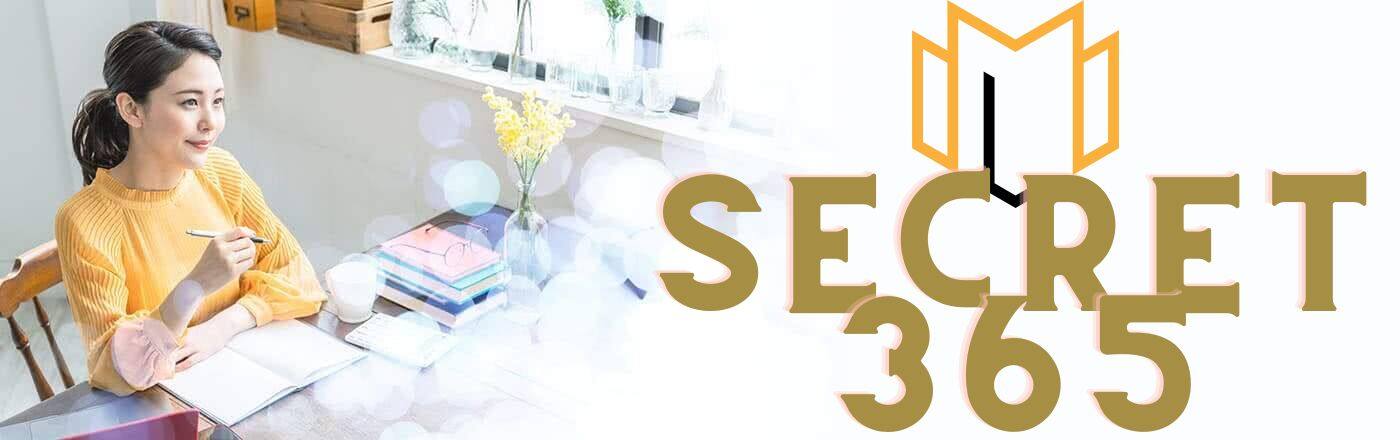
Unlock the power of TypeScript with decorators
Understanding TypeScript Decorators is essential for developers who want to leverage the power of decorators in their TypeScript projects. Decorators provide a way to modify or enhance the behavior of classes, methods, properties, or parameters at design time. They allow developers to add metadata, apply mixins, create decorators for dependency injection, and much more. This introduction aims to provide a brief overview of TypeScript decorators and their usage in modern web development.
TypeScript decorators are a powerful feature that allows developers to add metadata and modify the behavior of classes, methods, properties, and parameters at design time. They provide a way to extend and enhance the functionality of existing code without modifying its source code directly. In this article, we will explore the basics of TypeScript decorators and understand how they can be used to improve the maintainability and extensibility of our code.
To begin with, let's understand what decorators are. Decorators are special functions that can be attached to classes, methods, properties, or parameters using the `@` symbol followed by the decorator name. When a decorator is applied to a target, it receives information about the target and can perform actions based on that information. This allows us to modify the behavior of the target or add additional functionality to it.
One of the most common use cases of decorators is to add metadata to classes, methods, or properties. Metadata is additional information that describes the target and can be used by other parts of the code to make decisions or perform specific actions. For example, we can use decorators to add information about the validation rules for a property or to mark a method as deprecated.
Decorators can also be used to modify the behavior of a target. For example, we can use a decorator to log method calls, measure the execution time of a method, or add caching to a method. By applying decorators to our code, we can separate cross-cutting concerns from the core logic and improve the modularity and reusability of our code.
In addition to modifying the behavior of a target, decorators can also be used to replace or wrap the target with a new implementation. This is known as aspect-oriented programming (AOP) and allows us to add cross-cutting functionality to our code without modifying its source code directly. For example, we can use decorators to add authentication or authorization checks to methods or to implement transaction management.
TypeScript decorators can be applied to classes, methods, properties, or parameters. When a decorator is applied to a class, it receives the constructor function of the class as its target. This allows us to modify the class itself or add additional functionality to it. When a decorator is applied to a method, property, or parameter, it receives the prototype of the class as its target. This allows us to modify the behavior of the method or property or add additional functionality to it.
To create a decorator, we simply define a function that takes the target as its parameter and performs the desired actions. The function can return a new value or modify the target directly. The decorator function can also accept additional parameters to customize its behavior. For example, we can pass configuration options to a decorator or specify the metadata to be added.
In conclusion, TypeScript decorators are a powerful feature that allows developers to add metadata and modify the behavior of classes, methods, properties, and parameters at design time. They provide a way to extend and enhance the functionality of existing code without modifying its source code directly. By using decorators, we can improve the maintainability and extensibility of our code and separate cross-cutting concerns from the core logic. In the next sections, we will explore different types of decorators and learn how to create and use them in our TypeScript projects.
Common Use Cases for TypeScript Decorators
TypeScript decorators are a powerful feature that allows developers to add metadata and modify the behavior of classes, methods, properties, and parameters at runtime. They provide a way to extend and enhance the functionality of existing code without modifying its source. In this article, we will explore some common use cases for TypeScript decorators and how they can be leveraged to solve real-world problems.
One of the most common use cases for decorators is logging. By applying a decorator to a method, you can easily log information about its execution, such as the arguments passed in and the return value. This can be particularly useful for debugging and performance monitoring. With decorators, you can add logging functionality to multiple methods across different classes with minimal code duplication.
Another common use case for decorators is validation. By applying a decorator to a property or method, you can enforce certain rules or constraints on its value or arguments. For example, you can create a decorator that checks if a string property is not empty or if a method argument is of a specific type. This can help catch potential bugs and ensure that your code is more robust and reliable.
Authorization and authentication are also common use cases for decorators. By applying a decorator to a method or class, you can easily restrict access to certain resources or actions based on the user's role or permissions. This can be particularly useful in web applications where different users have different levels of access. With decorators, you can centralize the authorization logic and apply it consistently across your codebase.
Caching is another common use case for decorators. By applying a decorator to a method, you can cache its return value and avoid unnecessary computations or expensive operations. This can greatly improve the performance of your application, especially for methods that are called frequently or have long execution times. With decorators, you can easily add caching functionality to specific methods without modifying their implementation.
Dependency injection is a powerful design pattern that is often used in large-scale applications. With decorators, you can easily implement dependency injection by applying a decorator to a class constructor or property. This allows you to automatically inject dependencies into your classes without manually creating and managing instances. Decorators make it easier to decouple your code and improve its testability and maintainability.
Lastly, decorators can be used for profiling and performance monitoring. By applying a decorator to a method, you can measure its execution time and gather statistics about its performance. This can help identify bottlenecks and optimize your code for better efficiency. With decorators, you can easily add profiling functionality to specific methods without cluttering your code with profiling logic.
In conclusion, TypeScript decorators offer a wide range of possibilities for extending and enhancing the functionality of your code. From logging and validation to authorization and caching, decorators can be used to solve common problems and improve the quality and performance of your applications. By leveraging decorators, you can write cleaner, more maintainable code that is easier to test and debug. So, next time you encounter a scenario where you need to modify the behavior of your code, consider using decorators as a powerful tool in your TypeScript arsenal.
TypeScript decorators are a powerful feature that allows developers to add metadata and modify the behavior of classes, methods, properties, and parameters at runtime. They provide a way to extend and enhance the functionality of existing code without modifying its source. In this article, we will explore some advanced techniques for working with TypeScript decorators.
One of the most common use cases for decorators is to implement cross-cutting concerns such as logging, caching, and authentication. By applying decorators to classes or methods, you can easily add these functionalities to your codebase without cluttering the implementation logic. For example, you can create a logging decorator that logs method invocations along with their arguments and return values. This can be particularly useful for debugging and performance analysis.
Another powerful feature of decorators is the ability to apply them conditionally based on runtime information. This can be achieved by using factory functions that return the actual decorator based on certain conditions. For instance, you can create a decorator factory that applies a caching decorator only if a certain condition is met, such as the method being called with the same arguments multiple times. This allows you to selectively apply decorators based on the specific requirements of your application.
TypeScript decorators can also be used to implement dependency injection, a design pattern that promotes loose coupling and modularization of code. By applying decorators to constructor parameters, you can automatically inject dependencies into classes without explicitly instantiating them. This can greatly simplify the code and make it more maintainable. For example, you can create a decorator that automatically injects a logger instance into a class, eliminating the need to manually create and pass the logger object.
In addition to modifying the behavior of classes and methods, decorators can also be applied to properties and accessors. This allows you to add additional functionality to individual properties, such as validation or transformation. For example, you can create a decorator that ensures a property is always a positive number by validating its value before assignment. This can help enforce data integrity and prevent bugs caused by invalid values.
TypeScript decorators can also be used to implement aspect-oriented programming (AOP) techniques such as method interception and aspect weaving. Method interception allows you to intercept method calls before and after they are executed, enabling you to perform additional actions or modify the method's behavior. Aspect weaving, on the other hand, allows you to dynamically add functionality to existing methods without modifying their source code. This can be particularly useful for implementing cross-cutting concerns such as security checks or performance monitoring.
In conclusion, TypeScript decorators are a powerful feature that allows developers to extend and enhance the functionality of their codebase without modifying its source. They can be used to implement cross-cutting concerns, apply functionality conditionally, implement dependency injection, add functionality to properties, and implement aspect-oriented programming techniques. By understanding and leveraging these advanced techniques, you can take full advantage of decorators and write more modular, maintainable, and extensible code.
1. What are TypeScript decorators?
TypeScript decorators are a feature that allows you to add metadata and modify the behavior of classes, methods, properties, or parameters at design time.
2. How do TypeScript decorators work?
TypeScript decorators are functions that are prefixed with the `@` symbol and are applied to classes, methods, properties, or parameters using the decorator syntax. They can be used to modify the behavior or add additional functionality to the target element.
3. What are some use cases for TypeScript decorators?
TypeScript decorators can be used for various purposes, such as logging, validation, authentication, dependency injection, and more. They provide a way to separate cross-cutting concerns from the core logic of your code.
In conclusion, TypeScript decorators are a powerful feature that allows developers to add metadata and modify the behavior of classes, methods, properties, and parameters. They provide a way to enhance the functionality of existing code without modifying its original implementation. Decorators can be used for various purposes such as logging, validation, dependency injection, and more. Understanding TypeScript decorators is essential for developers looking to leverage the full potential of the TypeScript language and build more maintainable and extensible applications.