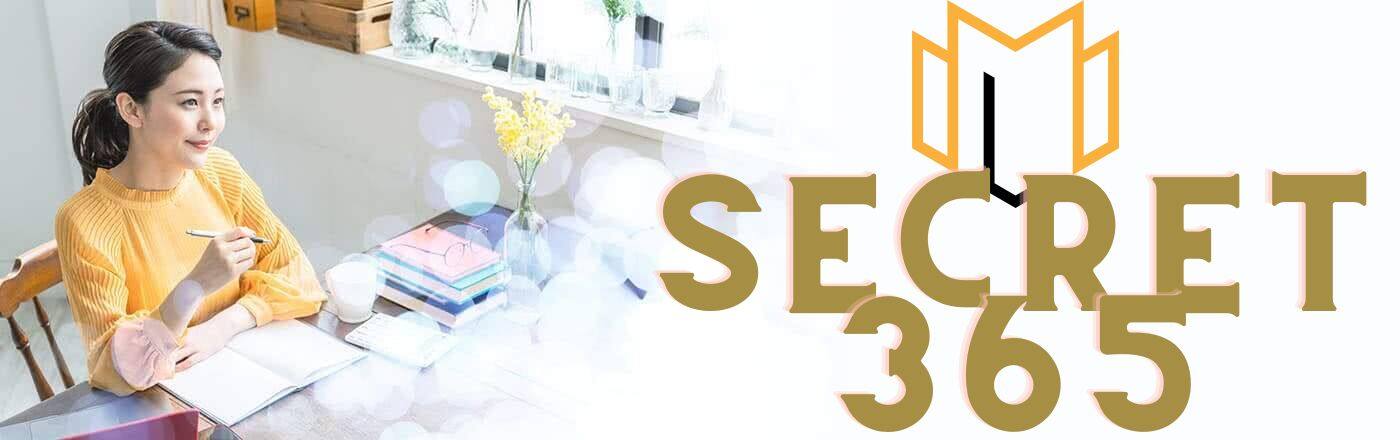
Unlocking the Power of Development: Essential Concepts for Developers
Introduction:
Essential Concepts for Developers refer to the fundamental principles, ideas, and knowledge that every developer should possess to excel in their field. These concepts serve as building blocks for understanding and implementing various programming languages, frameworks, and technologies. By grasping these essential concepts, developers can enhance their problem-solving skills, write efficient and maintainable code, and stay updated with the ever-evolving landscape of software development. In this article, we will explore some of the key essential concepts that developers should be familiar with to succeed in their careers.
Object-Oriented Programming (OOP) is a fundamental concept that every developer should understand. It provides a structured approach to software development, allowing for the creation of modular and reusable code. In this article, we will explore some essential concepts of OOP that developers need to grasp in order to write efficient and maintainable code.
One of the key principles of OOP is encapsulation. Encapsulation refers to the bundling of data and methods into a single unit, known as a class. This allows for the hiding of internal details and provides a clean interface for interacting with the class. By encapsulating data and methods, developers can ensure that the internal state of an object remains consistent and that changes to the implementation do not affect other parts of the code.
Inheritance is another important concept in OOP. It allows for the creation of new classes based on existing ones, inheriting their properties and behaviors. This promotes code reuse and allows for the creation of more specialized classes. For example, a developer can create a base class called "Animal" and then create derived classes such as "Dog" and "Cat" that inherit the common properties and behaviors of an animal. Inheritance helps to reduce code duplication and makes the code more maintainable.
Polymorphism is closely related to inheritance and is another essential concept in OOP. It allows objects of different classes to be treated as objects of a common superclass. This means that a method can be defined in the superclass and then overridden in the derived classes to provide different implementations. Polymorphism enables developers to write code that is more flexible and adaptable to different scenarios. For example, a developer can create a method called "makeSound" in the superclass "Animal" and then override it in the derived classes "Dog" and "Cat" to produce different sounds.
Abstraction is a concept that allows developers to focus on the essential features of an object while hiding unnecessary details. It provides a simplified view of an object, making it easier to understand and work with. Abstraction is achieved through the use of abstract classes and interfaces. Abstract classes define common properties and behaviors that derived classes must implement, while interfaces define a contract that classes must adhere to. By using abstraction, developers can create code that is more modular and easier to maintain.
Finally, OOP promotes the concept of composition, which is the building of complex objects by combining simpler objects. This allows for the creation of more flexible and reusable code. Instead of inheriting properties and behaviors from a superclass, objects are composed of other objects. This approach promotes loose coupling and makes it easier to change the behavior of an object without affecting other parts of the code.
In conclusion, understanding the essential concepts of Object-Oriented Programming is crucial for developers. Encapsulation, inheritance, polymorphism, abstraction, and composition are all fundamental concepts that enable developers to write efficient and maintainable code. By applying these concepts, developers can create modular and reusable code that is easier to understand and maintain. So, whether you are a beginner or an experienced developer, make sure to grasp these concepts and apply them in your software development projects.
Understanding Data Structures and Algorithms for Efficient Development
In the world of software development, efficiency is key. Developers are constantly striving to create programs that are fast, reliable, and scalable. One of the fundamental concepts that developers must grasp to achieve this is understanding data structures and algorithms.
Data structures are the building blocks of any program. They are the way in which data is organized, stored, and accessed. Choosing the right data structure for a particular task can have a significant impact on the performance of a program. There are many different types of data structures, each with its own strengths and weaknesses.
One commonly used data structure is the array. An array is a collection of elements, each identified by an index. Arrays are simple and efficient, allowing for constant-time access to any element. However, they have a fixed size and can be inefficient when it comes to inserting or deleting elements.
Another commonly used data structure is the linked list. Unlike arrays, linked lists can grow or shrink dynamically. Each element in a linked list contains a reference to the next element, forming a chain. Linked lists are efficient for inserting or deleting elements, but accessing a specific element requires traversing the list from the beginning.
Trees are another important data structure. A tree is a hierarchical structure consisting of nodes connected by edges. Each node can have zero or more child nodes. Trees are used to represent hierarchical relationships, such as file systems or organization charts. They are efficient for searching, inserting, and deleting elements, with many algorithms designed specifically for trees.
Understanding algorithms is equally important for efficient development. An algorithm is a step-by-step procedure for solving a problem. It is a set of instructions that takes input and produces output. Algorithms can be simple or complex, and their efficiency can vary greatly.
One commonly used algorithm is the sorting algorithm. Sorting is the process of arranging elements in a specific order, such as ascending or descending. There are many different sorting algorithms, each with its own trade-offs in terms of time complexity and space complexity. Some popular sorting algorithms include bubble sort, insertion sort, and quicksort.
Another important algorithm is the searching algorithm. Searching is the process of finding a specific element within a collection of elements. Like sorting algorithms, there are many different searching algorithms, each with its own trade-offs. Some popular searching algorithms include linear search, binary search, and hash-based search.
Efficient development requires a deep understanding of data structures and algorithms. By choosing the right data structure and algorithm for a specific task, developers can optimize the performance of their programs. They can reduce the time it takes to execute a program, minimize memory usage, and improve overall scalability.
In conclusion, understanding data structures and algorithms is essential for efficient development. Data structures are the building blocks of programs, and choosing the right one can have a significant impact on performance. Algorithms, on the other hand, are step-by-step procedures for solving problems, and their efficiency can vary greatly. By mastering these concepts, developers can create programs that are fast, reliable, and scalable.
Mastering Version Control Systems: A Developer's Essential Toolkit
Version control systems are an indispensable tool for developers, allowing them to efficiently manage and track changes to their codebase. Whether you are working on a solo project or collaborating with a team, understanding the essential concepts of version control systems is crucial for ensuring the success of your development process.
One of the fundamental concepts in version control systems is the repository. A repository is a central location where all the code and its history are stored. It serves as a single source of truth, enabling developers to access and modify the codebase. There are two types of repositories: local and remote. A local repository resides on your local machine, while a remote repository is hosted on a server, allowing multiple developers to collaborate on the same codebase.
To effectively manage changes to the codebase, version control systems utilize the concept of commits. A commit represents a snapshot of the code at a specific point in time. It includes all the changes made since the last commit, along with a unique identifier. Commits provide a detailed history of the codebase, allowing developers to track changes, revert to previous versions, and collaborate seamlessly.
Branching is another essential concept in version control systems. It allows developers to create separate lines of development, known as branches, without affecting the main codebase. Branches are useful for working on new features, bug fixes, or experiments without disrupting the stability of the main codebase. Once the changes in a branch are complete and tested, they can be merged back into the main codebase, ensuring a smooth integration of new features.
Merging is the process of combining changes from one branch into another. When merging branches, version control systems automatically detect and resolve conflicts, ensuring that the final codebase is consistent and error-free. Merging is a critical step in the development process, as it allows developers to collaborate effectively and integrate their work seamlessly.
Another important concept in version control systems is the concept of pull requests. Pull requests provide a mechanism for developers to propose changes to the codebase and request a review from their peers. They allow for a structured and collaborative approach to code review, ensuring that changes are thoroughly examined before being merged into the main codebase. Pull requests also facilitate discussions and feedback, fostering a culture of continuous improvement and learning within the development team.
Version control systems also offer powerful features for managing conflicts. Conflicts occur when two or more developers make conflicting changes to the same file or code section. Version control systems provide tools to detect and resolve conflicts, allowing developers to merge their changes seamlessly. Resolving conflicts requires careful analysis and collaboration, ensuring that the final codebase is consistent and error-free.
In conclusion, mastering version control systems is essential for developers. Understanding the concepts of repositories, commits, branching, merging, pull requests, and conflict resolution is crucial for efficient and collaborative development. By leveraging the power of version control systems, developers can streamline their workflow, track changes effectively, and ensure the stability and quality of their codebase. So, whether you are a solo developer or part of a team, investing time in mastering version control systems is a wise decision that will pay off in the long run.
1. What are essential concepts for developers?
- Object-oriented programming
- Data structures and algorithms
- Version control systems
2. Why are object-oriented programming concepts important for developers?
- They allow for modular and reusable code
- Encourage code organization and maintainability
- Enable code abstraction and encapsulation
3. How do data structures and algorithms benefit developers?
- Efficiently store and manipulate data
- Improve program performance and scalability
- Solve complex problems effectively
In conclusion, understanding essential concepts is crucial for developers. These concepts provide a foundation for building robust and efficient software solutions. Key areas include data structures, algorithms, programming paradigms, software design principles, and system architecture. Additionally, knowledge of programming languages, version control, debugging techniques, and testing methodologies is essential. By mastering these concepts, developers can enhance their problem-solving skills and deliver high-quality software products.