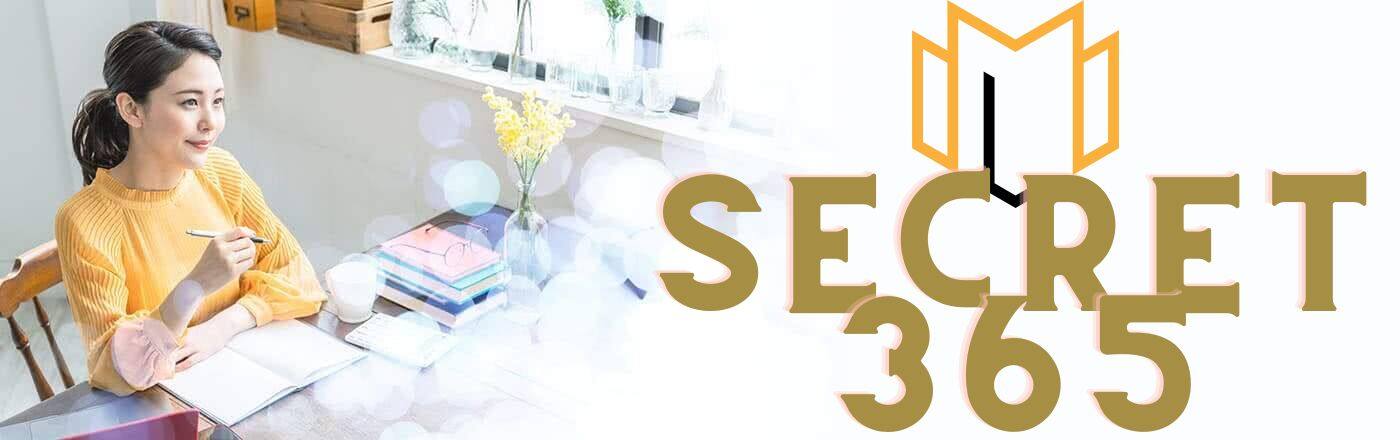
Your go-to resource for mastering Bash syntax quickly.
A Quick Reference Guide for Bash Syntax is a concise and handy resource that provides a summary of the essential syntax and commands used in the Bash scripting language. It serves as a quick and easy reference for both beginners and experienced users, helping them navigate through the various aspects of Bash scripting efficiently. This guide covers a wide range of topics, including variable declaration, control structures, command-line arguments, file manipulation, and more. Whether you need a quick reminder or a comprehensive overview, this reference guide is designed to assist you in writing efficient and effective Bash scripts.
Bash, short for "Bourne Again SHell," is a command language interpreter that is widely used in Unix-based operating systems. It is the default shell for most Linux distributions and macOS. Understanding the syntax of Bash is essential for anyone working with the command line interface. In this quick reference guide, we will provide an overview of the basic syntax used in Bash scripting.
At its core, Bash is a scripting language that allows users to automate tasks and execute commands. It uses a combination of built-in commands, external commands, and shell scripts to perform various operations. The syntax of Bash is similar to other programming languages, with a few unique features.
One of the fundamental components of Bash syntax is the command line prompt. The prompt is the text that appears on the screen, indicating that the shell is ready to accept commands. By default, the prompt in Bash is represented by a dollar sign ($). However, it can be customized to display additional information, such as the current directory or the username.
Commands in Bash are executed by typing their name followed by any necessary arguments or options. Arguments are values that provide additional information to the command, while options modify the behavior of the command. Options are typically preceded by a hyphen (-) or two hyphens (--).
Bash supports various types of variables, which are used to store and manipulate data. Variables can be assigned values using the assignment operator (=). It is important to note that Bash is a weakly typed language, meaning that variables do not have a specific data type. They can hold any type of data, such as numbers, strings, or even arrays.
To access the value of a variable, you can use the dollar sign ($) followed by the variable name. For example, if a variable named "name" contains the value "John," you can display it on the screen by typing echo $name. Additionally, you can perform operations on variables, such as concatenation or arithmetic calculations.
Conditional statements are another crucial aspect of Bash syntax. They allow you to control the flow of your script based on certain conditions. The most common conditional statement in Bash is the if statement. It evaluates a condition and executes a block of code if the condition is true. The syntax of an if statement is as follows:
if [ condition ]; then
# code to be executed if the condition is true
fi
In Bash, conditions are typically expressed using comparison operators, such as -eq (equal), -ne (not equal), -lt (less than), -gt (greater than), and so on. You can also use logical operators, such as && (and) and || (or), to combine multiple conditions.
Loops are another powerful feature of Bash syntax. They allow you to repeat a block of code multiple times. The most commonly used loop in Bash is the for loop. It iterates over a list of values and executes a block of code for each value. The syntax of a for loop is as follows:
for variable in list; do
# code to be executed for each value
done
In addition to the for loop, Bash also supports the while loop and the until loop, which execute a block of code as long as a condition is true or false, respectively.
In conclusion, understanding the syntax of Bash is essential for anyone working with the command line interface. This quick reference guide provided an overview of the basic syntax used in Bash scripting, including commands, variables, conditional statements, and loops. By mastering these concepts, you will be able to write efficient and powerful Bash scripts to automate tasks and streamline your workflow.
Bash, short for "Bourne Again SHell," is a command language interpreter that is widely used in Unix-based operating systems. It provides a powerful and flexible way to interact with the operating system and execute commands. Whether you are a beginner or an experienced user, having a quick reference guide for Bash syntax can be incredibly helpful. In this article, we will provide you with a comprehensive overview of essential Bash commands, covering everything from basic commands to more advanced features.
Let's start with the basics. The first thing you need to know is how to execute a command in Bash. Simply type the command you want to execute and press Enter. For example, if you want to list the files in the current directory, you can use the "ls" command. To execute it, type "ls" and press Enter.
Now, let's move on to command options. Most commands in Bash have various options that modify their behavior. Options are usually preceded by a hyphen (-) or two hyphens (--). For example, the "ls" command has the "-l" option, which displays detailed information about files and directories. To use this option, type "ls -l" and press Enter.
In addition to options, commands in Bash can also take arguments. Arguments are additional pieces of information that provide further instructions to the command. For example, the "cp" command is used to copy files. To copy a file from one location to another, you need to provide the source file and the destination directory as arguments. The syntax for the "cp" command is "cp [source] [destination]".
Now, let's explore some more advanced features of Bash. One powerful feature is command substitution, which allows you to use the output of one command as an argument to another command. To perform command substitution, enclose the command you want to substitute in backticks (`) or use the dollar sign followed by parentheses ($()). For example, if you want to display the current date and time, you can use the "date" command with command substitution like this: "echo The current date and time is $(date)".
Another useful feature is the ability to redirect input and output. By default, commands in Bash read input from the keyboard and write output to the screen. However, you can redirect input and output to and from files using the greater than (>) and less than (" symbol like this: "command > file.txt".
Lastly, let's talk about pipelines. A pipeline allows you to connect multiple commands together, with the output of one command serving as the input to the next command. To create a pipeline, use the vertical bar (|) symbol. For example, if you want to list all the files in the current directory and then sort them alphabetically, you can use the "ls" command with the "-l" option and pipe the output to the "sort" command like this: "ls -l | sort".
In conclusion, having a quick reference guide for Bash syntax is essential for both beginners and experienced users. It allows you to quickly look up commands, options, and syntax, making your interaction with the operating system more efficient. In this article, we covered the basics of executing commands, using options and arguments, as well as more advanced features like command substitution, input/output redirection, and pipelines. With this knowledge, you will be well-equipped to navigate the world of Bash and unleash its full potential.
Bash, short for "Bourne Again SHell," is a powerful scripting language commonly used in Unix-based operating systems. It provides a command-line interface for users to interact with the system and automate tasks. Understanding the syntax of Bash is essential for writing efficient and effective scripts. In this quick reference guide, we will explore some advanced Bash syntax and provide examples to help you master this scripting language.
One of the fundamental aspects of Bash syntax is variable assignment. Variables in Bash are defined by using the assignment operator (=). For example, to assign the value "Hello, World!" to a variable named greeting, you would write:
greeting="Hello, World!"
To access the value of a variable, you can use the dollar sign ($) followed by the variable name. For instance, to print the value of the greeting variable, you would use the echo command:
echo $greeting
Bash also supports arithmetic operations. You can perform addition, subtraction, multiplication, and division using the let command. For example, to add two numbers and store the result in a variable called sum, you would write:
let sum=5+3
To execute commands conditionally, Bash provides the if statement. The syntax for the if statement is as follows:
if [ condition ]; then
# code to be executed if the condition is true
else
# code to be executed if the condition is false
fi
For instance, to check if a file exists and print a message accordingly, you can use the following code:
if [ -f "myfile.txt" ]; then
echo "File exists."
else
echo "File does not exist."
fi
Bash also supports loops, allowing you to repeat a set of commands multiple times. The for loop is commonly used to iterate over a list of items. Here's an example that prints the numbers from 1 to 5:
for i in 1 2 3 4 5; do
echo $i
done
Another useful loop is the while loop, which executes a block of code as long as a condition is true. For example, the following code prints the numbers from 1 to 5 using a while loop:
counter=1
while [ $counter -le 5 ]; do
echo $counter
let counter=counter+1
done
In addition to variables, arithmetic operations, conditionals, and loops, Bash provides various other features to enhance your scripting capabilities. These include functions, command substitution, string manipulation, and file handling.
Functions allow you to group a set of commands together and execute them as a single unit. Command substitution allows you to capture the output of a command and use it as a variable or argument. String manipulation functions enable you to manipulate and extract information from strings. File handling features allow you to read from and write to files, as well as perform operations such as copying, moving, and deleting files.
Mastering Bash syntax is crucial for advanced Bash scripting. By understanding variables, arithmetic operations, conditionals, loops, and other features, you can write powerful scripts to automate tasks and streamline your workflow. This quick reference guide provides a starting point for exploring the vast capabilities of Bash scripting. With practice and experimentation, you can become proficient in Bash and leverage its power to become a more efficient and effective scripter.
1. What is a Quick Reference Guide for Bash Syntax?
A Quick Reference Guide for Bash Syntax is a concise document that provides a summary of the most commonly used commands, operators, and syntax in the Bash scripting language.
2. What is the purpose of a Quick Reference Guide for Bash Syntax?
The purpose of a Quick Reference Guide for Bash Syntax is to serve as a handy reference tool for Bash scripters, allowing them to quickly look up and refresh their memory on the syntax and usage of various commands and operators.
3. What information can be found in a Quick Reference Guide for Bash Syntax?
A Quick Reference Guide for Bash Syntax typically includes information on basic commands, control structures, variables, input/output redirection, command substitution, conditional statements, loops, and other essential elements of Bash scripting.
In conclusion, a Quick Reference Guide for Bash Syntax is a valuable resource for both beginners and experienced users of the Bash scripting language. It provides a concise and organized overview of the various commands, operators, and syntax used in Bash, making it easier to write and understand scripts. With this guide, users can quickly reference the necessary information to effectively navigate and utilize the power of Bash.