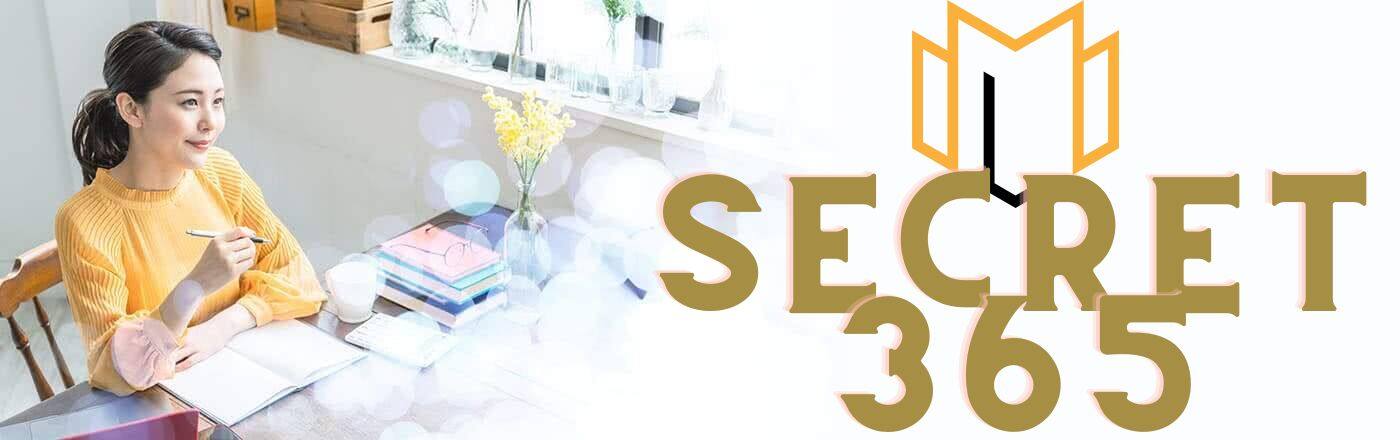
Master the SOLID principles and unlock the power of clean and maintainable code.
The SOLID principles are a set of design principles that aim to make software systems more maintainable, flexible, and scalable. They provide guidelines for writing clean, modular, and robust code. Understanding and applying these principles is crucial for software developers to create high-quality software solutions. In this article, we will explore the SOLID principles in detail and discuss their importance in software development.
Do you truly comprehend the SOLID principles? If you are a software developer, understanding these principles is crucial for writing clean, maintainable, and scalable code. The SOLID principles are a set of five design principles that were introduced by Robert C. Martin, also known as Uncle Bob, in the early 2000s. These principles provide guidelines for writing software that is easy to understand, flexible, and robust.
The first principle, the Single Responsibility Principle (SRP), states that a class should have only one reason to change. In other words, a class should have only one responsibility or job. This principle encourages developers to create classes that are focused and have a clear purpose. By adhering to the SRP, code becomes easier to understand, test, and maintain.
The Open-Closed Principle (OCP) is the second principle in the SOLID set. It states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means that you should be able to add new functionality to a system without modifying its existing code. By following the OCP, you can avoid introducing bugs or breaking existing functionality when making changes to your code.
Next, we have the Liskov Substitution Principle (LSP). This principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. In simpler terms, this means that subclasses should be able to be used interchangeably with their parent classes. By adhering to the LSP, you can ensure that your code is flexible and can be easily extended without introducing unexpected behavior.
The Interface Segregation Principle (ISP) emphasizes that clients should not be forced to depend on interfaces they do not use. This principle encourages developers to create small, focused interfaces that are tailored to the specific needs of the clients. By following the ISP, you can avoid creating bloated interfaces that are difficult to implement and maintain.
Lastly, we have the Dependency Inversion Principle (DIP). This principle states that high-level modules should not depend on low-level modules. Instead, both should depend on abstractions. This principle promotes loose coupling between modules, making it easier to change or replace one module without affecting others. By adhering to the DIP, you can create code that is more flexible, reusable, and testable.
Understanding and applying the SOLID principles can greatly improve the quality of your code. By following these principles, you can create software that is easier to understand, maintain, and extend. Your code will be more robust, less prone to bugs, and more adaptable to changing requirements. Additionally, adhering to the SOLID principles can make your code more testable, allowing you to write comprehensive unit tests that ensure the correctness of your software.
In conclusion, the SOLID principles are a set of design principles that provide guidelines for writing clean, maintainable, and scalable code. By understanding and applying these principles, you can improve the quality of your software and become a more effective software developer. So, take the time to truly comprehend the SOLID principles and start writing code that is solid.
Do you truly comprehend the SOLID principles?
When it comes to software development, adhering to best practices is crucial for creating maintainable and scalable code. One set of principles that has gained significant popularity in recent years is the SOLID principles. These principles, first introduced by Robert C. Martin, provide guidelines for writing clean and modular code. In this article, we will explore the five SOLID principles and their practical applications.
The first principle, the Single Responsibility Principle (SRP), states that a class should have only one reason to change. In other words, a class should have a single responsibility or job. By adhering to this principle, we can ensure that our classes are focused and have a clear purpose. This makes our code easier to understand, test, and maintain. For example, if we have a class that handles both user authentication and database operations, we can refactor it into two separate classes, each with its own responsibility.
The second principle, the Open-Closed Principle (OCP), emphasizes the importance of code extensibility. According to this principle, software entities should be open for extension but closed for modification. This means that we should be able to add new functionality to our code without modifying existing code. By using techniques such as inheritance and interfaces, we can achieve this goal. For instance, if we have a class that calculates the area of different shapes, we can make it open for extension by creating a Shape interface and implementing it in various shape classes.
The third principle, the Liskov Substitution Principle (LSP), deals with the concept of substitutability. It states that objects of a superclass should be able to be replaced with objects of its subclasses without affecting the correctness of the program. In simpler terms, this principle ensures that inheritance is used correctly. By following this principle, we can create code that is more flexible and easier to maintain. For example, if we have a class hierarchy for different types of animals, we should be able to substitute any animal object with another animal object without breaking the code.
The fourth principle, the Interface Segregation Principle (ISP), focuses on the idea of creating specific interfaces for clients instead of having a single large interface. This principle encourages us to design fine-grained interfaces that are tailored to the needs of individual clients. By doing so, we can avoid forcing clients to depend on methods they don't need. For instance, if we have a class that provides various services, we can split it into multiple interfaces, each catering to a specific client's requirements.
The fifth and final principle, the Dependency Inversion Principle (DIP), promotes loose coupling between classes by inverting the traditional dependency relationships. According to this principle, high-level modules should not depend on low-level modules; both should depend on abstractions. This allows for easier code maintenance and testing. For example, if we have a class that depends on a specific database implementation, we can invert the dependency by introducing an interface and having the class depend on the interface instead.
In conclusion, understanding and applying the SOLID principles can greatly improve the quality of our code. By following the Single Responsibility Principle, Open-Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle, we can create code that is modular, extensible, and maintainable. These principles provide a solid foundation for writing clean and scalable software. So, the next time you embark on a software development project, make sure you truly comprehend the SOLID principles and apply them to your code.
Do you truly comprehend the SOLID principles? Common Misconceptions and Challenges in Implementing the SOLID Principles.
The SOLID principles are a set of five design principles that aim to make software systems more maintainable, flexible, and scalable. While these principles have gained popularity in the software development community, there are still common misconceptions and challenges that developers face when trying to implement them effectively.
One common misconception is that the SOLID principles are rigid rules that must be followed without question. In reality, the principles are guidelines that can be adapted and applied in different ways depending on the specific context of a project. It is important to understand the underlying principles behind each of the SOLID principles and use them as a foundation for making informed design decisions.
Another challenge in implementing the SOLID principles is the lack of understanding of the dependencies between different components of a system. The SOLID principles emphasize the importance of minimizing dependencies and promoting loose coupling between classes. However, identifying and managing dependencies can be a complex task, especially in large and complex systems. It requires a deep understanding of the system's architecture and careful consideration of the potential impact of changes.
One specific challenge that developers often face is the violation of the Single Responsibility Principle (SRP). This principle states that a class should have only one reason to change. However, it is not always easy to determine what constitutes a single responsibility. Different developers may have different interpretations of what constitutes a single responsibility, leading to inconsistent application of the principle. It requires clear communication and collaboration among team members to ensure a shared understanding of the responsibilities of each class.
The Open-Closed Principle (OCP) is another principle that can be challenging to implement. This principle states that software entities should be open for extension but closed for modification. In other words, it promotes the use of abstraction and interfaces to allow for easy extension without modifying existing code. However, identifying the right abstractions and designing interfaces that are flexible and extensible can be difficult. It requires careful planning and foresight to anticipate future changes and design the system accordingly.
The Liskov Substitution Principle (LSP) is often misunderstood and misapplied. This principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. However, it is not enough to simply inherit from a superclass and override methods. The subclasses must adhere to the contract defined by the superclass and not introduce any new behaviors or violate any assumptions made by the superclass. This requires a deep understanding of the semantics and behavior of the superclass and careful consideration of the design of the subclasses.
The Interface Segregation Principle (ISP) can also be challenging to implement, especially in systems with complex and interconnected components. This principle states that clients should not be forced to depend on interfaces they do not use. It promotes the use of small, cohesive interfaces that are tailored to the specific needs of clients. However, identifying the right set of interfaces and managing the dependencies between components can be difficult. It requires careful analysis of the interactions between components and the needs of clients to design interfaces that are both flexible and easy to use.
In conclusion, while the SOLID principles provide valuable guidelines for designing maintainable and flexible software systems, there are common misconceptions and challenges that developers face when trying to implement them effectively. It is important to understand the underlying principles behind each of the SOLID principles and adapt them to the specific context of a project. Clear communication, collaboration, and careful planning are essential to overcome these challenges and reap the benefits of SOLID design principles.
1. What are the SOLID principles?
The SOLID principles are a set of five design principles in object-oriented programming that aim to make software systems more maintainable, flexible, and scalable.
2. What does SOLID stand for?
SOLID is an acronym that stands for Single Responsibility Principle, Open-Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle.
3. Why are the SOLID principles important?
The SOLID principles help developers create software that is easier to understand, modify, and extend. They promote modular design, reduce code duplication, and enhance code reusability, leading to more robust and maintainable software systems.
In conclusion, understanding the SOLID principles requires a deep comprehension of the concepts and their application in software development. It is essential to grasp the principles individually and as a whole to effectively design and maintain robust, flexible, and maintainable software systems.