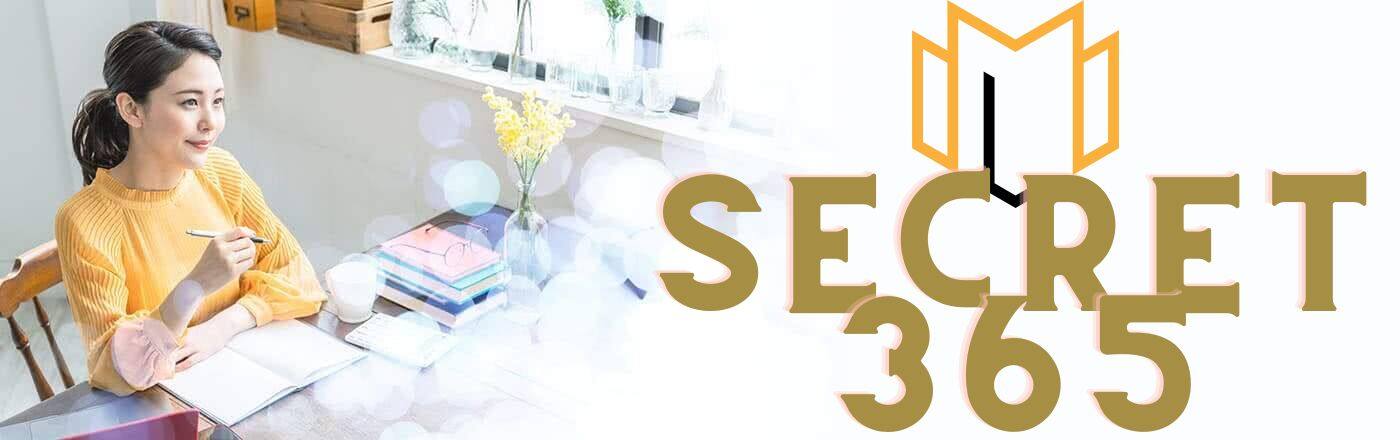
"Master the power of arrays in C and unlock limitless possibilities."
Introduction to Arrays in C:
In the C programming language, an array is a collection of elements of the same data type, stored in contiguous memory locations. Arrays provide a convenient way to store and access multiple values of the same type using a single variable name. Each element in an array is accessed by its index, which represents its position within the array. Arrays in C are widely used for various purposes, such as storing a list of numbers, characters, or even complex data structures. Understanding arrays is fundamental to becoming proficient in C programming.
Arrays are an essential concept in programming, and understanding how they work is crucial for any aspiring programmer. In the C programming language, arrays play a fundamental role in storing and manipulating data. In this article, we will delve into the basics of arrays in C, exploring their definition, declaration, and usage.
To put it simply, an array is a collection of elements of the same data type. These elements are stored in contiguous memory locations, allowing for easy access and manipulation. Arrays provide a convenient way to store and retrieve multiple values using a single variable name.
In C, arrays are declared by specifying the data type of the elements they will hold, followed by the array name and the size of the array in square brackets. For example, to declare an array of integers with a size of 5, we would write:
int myArray[5];
This declaration tells the compiler to allocate memory for five integers and associate it with the variable name "myArray." It is important to note that arrays in C are zero-indexed, meaning the first element is accessed using an index of 0, the second element with an index of 1, and so on.
Once an array is declared, we can assign values to its elements using the assignment operator (=) and the index of the element we want to modify. For instance, to assign the value 10 to the first element of "myArray," we would write:
myArray[0] = 10;
Similarly, we can retrieve the value of an element by using its index. To print the value of the third element in "myArray," we would write:
printf("%d", myArray[2]);
Arrays can also be initialized at the time of declaration. This means that we can assign values to the elements of an array when we declare it. To initialize "myArray" with the values 1, 2, 3, 4, and 5, we would write:
int myArray[5] = {1, 2, 3, 4, 5};
It is worth noting that if we initialize an array with fewer values than its size, the remaining elements will be automatically set to zero. For example, if we initialize an array of size 10 with only three values, the remaining seven elements will be set to zero.
Arrays in C can be used in various ways, such as storing a collection of numbers, characters, or even user-defined data types. They are particularly useful when dealing with large amounts of data or when performing repetitive tasks.
One common use of arrays is in loops. By using a loop, we can iterate over the elements of an array and perform operations on each element. This allows us to avoid writing repetitive code and makes our programs more efficient.
In conclusion, arrays are a fundamental concept in C programming. They provide a way to store and manipulate multiple values using a single variable name. By understanding how to declare, initialize, and use arrays, programmers can efficiently work with large amounts of data and perform repetitive tasks.
Arrays are an essential data structure in programming, allowing us to store multiple values of the same type in a single variable. In the C programming language, arrays are widely used and offer a convenient way to manage and manipulate data efficiently. In this article, we will explore the basics of arrays in C, starting with declaring and initializing arrays.
To declare an array in C, we need to specify the data type of the elements it will hold, followed by the name of the array. The syntax for declaring an array is as follows:
```
data_type array_name[array_size];
```
Here, `data_type` represents the type of elements the array will store, such as integers, characters, or floats. `array_name` is the identifier we choose for the array, and `array_size` indicates the number of elements the array can hold. It's important to note that the array size must be a positive integer.
Once we have declared an array, we can initialize it by assigning values to its elements. There are two ways to initialize an array in C: static initialization and dynamic initialization.
Static initialization involves explicitly assigning values to each element of the array at the time of declaration. We enclose the values within curly braces `{}` and separate them with commas. The number of values provided must match the size of the array. For example:
```
int numbers[5] = {1, 2, 3, 4, 5};
```
In this example, we declare an integer array named `numbers` with a size of 5. We initialize it with the values 1, 2, 3, 4, and 5.
Dynamic initialization allows us to assign values to the array elements after declaration using a loop or other logic. We can use the assignment operator `=` to assign values to individual elements. For instance:
```
int numbers[5];
numbers[0] = 1;
numbers[1] = 2;
numbers[2] = 3;
numbers[3] = 4;
numbers[4] = 5;
```
Here, we declare an integer array named `numbers` with a size of 5. Then, we assign values to each element using the index notation `array_name[index]`. The index starts from 0 and goes up to `array_size - 1`.
It's worth mentioning that we can also declare and initialize an array in a single line. This is known as combined declaration and initialization. For example:
```
int numbers[] = {1, 2, 3, 4, 5};
```
In this case, we omit the array size, and the compiler automatically determines it based on the number of values provided within the curly braces.
In addition to initializing arrays with constant values, we can also use variables or expressions. This allows us to create more flexible and dynamic arrays. For instance:
```
int size = 5;
int numbers[size] = {1, 2, 3, 4, 5};
```
Here, we declare an integer variable `size` and assign it the value 5. Then, we declare an array named `numbers` with a size equal to the value of `size`. Finally, we initialize the array with the values 1, 2, 3, 4, and 5.
In conclusion, declaring and initializing arrays in C is a fundamental concept that lays the foundation for working with arrays effectively. By understanding the syntax and techniques for declaring and initializing arrays, we can create arrays of different sizes and populate them with values. This knowledge is crucial for building more complex programs that require efficient data storage and manipulation.
Accessing and Manipulating Array Elements in C
In the previous section, we introduced the concept of arrays in the C programming language. We discussed how arrays are a collection of elements of the same data type, stored in contiguous memory locations. Now, let's delve deeper into how we can access and manipulate these elements in C.
To access an element in an array, we use the array name followed by the index of the element we want to access enclosed in square brackets. The index of the first element in an array is always 0, and the index of the last element is the size of the array minus one. For example, if we have an array called "numbers" with 5 elements, we can access the third element using the expression "numbers[2]".
It is important to note that accessing an element outside the bounds of an array results in undefined behavior. This means that the program may crash, produce incorrect results, or exhibit any other unpredictable behavior. Therefore, it is crucial to ensure that we always access array elements within their valid range.
Once we have accessed an array element, we can manipulate its value by assigning a new value to it. For example, if we want to change the value of the third element in the "numbers" array, we can use the assignment operator (=) like this: "numbers[2] = 10;". This statement assigns the value 10 to the third element of the array.
We can also perform various operations on array elements, such as arithmetic operations or applying functions. For instance, if we have an array called "grades" that stores the grades of students, we can calculate the average grade by summing up all the elements and dividing by the number of elements. This can be achieved using a loop to iterate over the array and accumulate the sum of the grades.
In addition to accessing individual elements, we can also access and manipulate multiple elements of an array simultaneously. This can be done using loops, such as the for loop or the while loop. These loops allow us to iterate over the array and perform operations on each element. For example, we can multiply all the elements of an array by a constant factor or find the maximum or minimum value in an array.
Another important aspect of manipulating arrays is swapping elements. Swapping two elements in an array involves exchanging their values. This operation is often used in sorting algorithms or when reordering elements based on certain criteria. To swap two elements, we need to use a temporary variable to hold the value of one element while we assign the value of the other element to it.
In conclusion, accessing and manipulating array elements in C is a fundamental skill that every programmer should master. By understanding how to access elements using their indices and how to manipulate their values, we can perform a wide range of operations on arrays. Whether it is calculating averages, sorting elements, or performing arithmetic operations, arrays provide a powerful tool for organizing and manipulating data in C. However, it is crucial to always ensure that we access array elements within their valid range to avoid undefined behavior.
1. What is an array in C?
An array in C is a collection of elements of the same data type, stored in contiguous memory locations, and accessed using an index.
2. How do you declare an array in C?
To declare an array in C, you specify the data type of the elements followed by the name of the array and the size of the array in square brackets. For example, int numbers[5]; declares an array named "numbers" that can hold 5 integers.
3. How do you access elements in an array in C?
You can access elements in an array in C by using the array name followed by the index of the element you want to access in square brackets. For example, numbers[2] would access the third element in the "numbers" array. The index starts from 0, so the first element is accessed using numbers[0].
In conclusion, the introduction to arrays in C provides a fundamental understanding of how arrays work and their importance in programming. It covers topics such as array declaration, initialization, accessing elements, and manipulating array data. Arrays are powerful data structures that allow for efficient storage and retrieval of multiple values of the same data type. Understanding arrays is crucial for developing efficient and effective C programs.