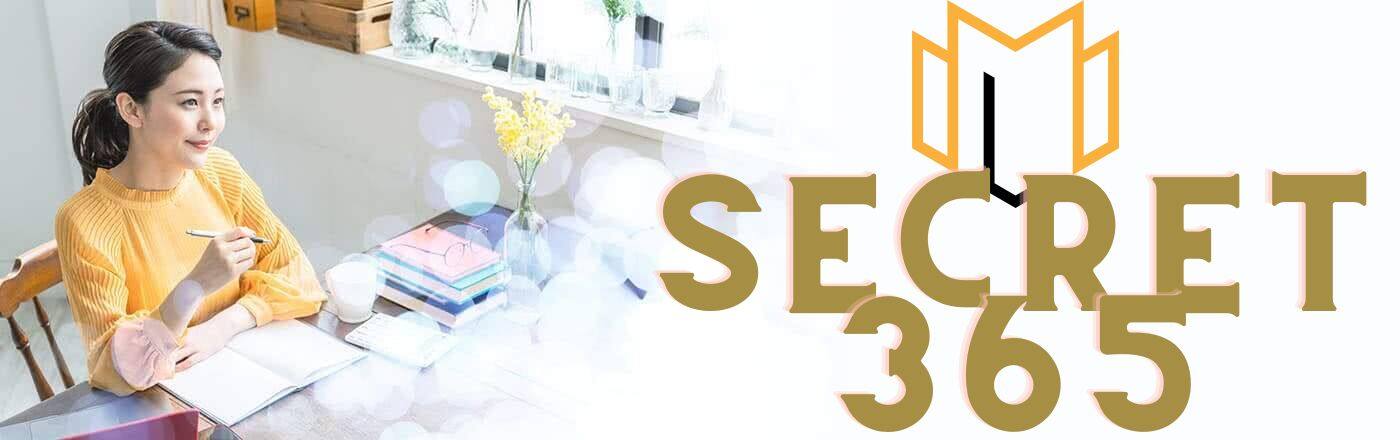
Master Python for Machine Learning with this Beginner's Guide.
A Beginner's Guide to Python for Machine Learning is a comprehensive introduction to using the Python programming language for machine learning tasks. This guide is designed for individuals who are new to both Python and machine learning, providing a step-by-step approach to learning the basics of Python and how to apply it to machine learning algorithms. By the end of this guide, readers will have a solid foundation in Python programming and be able to start building their own machine learning models.
Python has become one of the most popular programming languages for machine learning due to its simplicity and versatility. Whether you are a beginner or an experienced programmer, Python provides a user-friendly environment for developing machine learning models. In this article, we will provide a beginner's guide to Python for machine learning, starting with an introduction to the language itself.
Python is an interpreted, high-level programming language that was first released in 1991. It was designed with readability and simplicity in mind, making it an ideal choice for beginners. One of the key advantages of Python is its extensive library support, which includes libraries specifically tailored for machine learning, such as NumPy, Pandas, and Scikit-learn.
Before diving into machine learning, it is important to have a basic understanding of Python syntax and data structures. Python uses indentation to define blocks of code, which makes it easy to read and understand. Variables in Python do not need to be explicitly declared and can be assigned values of different types, such as integers, floats, strings, and booleans.
Python provides a wide range of data structures, including lists, tuples, sets, and dictionaries. Lists are ordered collections of elements, while tuples are similar to lists but are immutable. Sets are unordered collections of unique elements, and dictionaries are key-value pairs. Understanding these data structures is crucial for manipulating and analyzing data in machine learning.
In addition to its built-in data structures, Python offers powerful libraries for data manipulation and analysis. NumPy, short for Numerical Python, provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. Pandas, on the other hand, offers data structures and functions for efficient data manipulation and analysis, such as data frames and series.
Once you have a good grasp of Python syntax and data structures, you can start exploring machine learning libraries. Scikit-learn is a popular library that provides a wide range of machine learning algorithms and tools for data preprocessing, model selection, and evaluation. It is built on top of NumPy, SciPy, and Matplotlib, making it easy to integrate with other scientific computing libraries.
Another important library for machine learning in Python is TensorFlow. Developed by Google, TensorFlow is an open-source library for numerical computation and large-scale machine learning. It allows you to build and train deep learning models using a flexible and efficient programming interface. TensorFlow also provides tools for visualizing and debugging models, making it a valuable resource for machine learning practitioners.
In conclusion, Python is an excellent choice for beginners looking to get started with machine learning. Its simplicity, readability, and extensive library support make it a powerful tool for developing machine learning models. By familiarizing yourself with Python syntax, data structures, and machine learning libraries such as Scikit-learn and TensorFlow, you can begin your journey into the exciting world of machine learning.
Python has become one of the most popular programming languages for machine learning due to its simplicity and versatility. With its extensive collection of libraries, Python provides a solid foundation for building machine learning models. In this section, we will explore some essential Python libraries that every beginner should be familiar with when starting their journey into machine learning.
One of the most widely used libraries for machine learning in Python is scikit-learn. Scikit-learn is a powerful and user-friendly library that provides a wide range of algorithms and tools for various machine learning tasks. It includes modules for classification, regression, clustering, dimensionality reduction, and model selection. Scikit-learn also offers a comprehensive set of utilities for data preprocessing, feature extraction, and model evaluation. Its intuitive API and extensive documentation make it an excellent choice for beginners.
Another essential library for machine learning in Python is TensorFlow. Developed by Google, TensorFlow is an open-source library that provides a flexible and efficient framework for building and training deep learning models. It offers a high-level API called Keras, which simplifies the process of building neural networks. TensorFlow also supports distributed computing, allowing you to train models on multiple GPUs or even across multiple machines. With its extensive community support and vast ecosystem, TensorFlow is a must-have tool for any machine learning practitioner.
PyTorch is another popular library for deep learning in Python. Developed by Facebook's AI research lab, PyTorch provides a dynamic computational graph that allows for more flexibility and ease of use compared to TensorFlow. It offers a seamless integration with NumPy, a fundamental library for numerical computing in Python. PyTorch also provides a high-level API called torchvision, which includes pre-trained models and datasets for computer vision tasks. With its intuitive interface and extensive documentation, PyTorch is an excellent choice for beginners in deep learning.
Pandas is a powerful library for data manipulation and analysis in Python. It provides data structures and functions for efficiently handling structured data, such as CSV files or SQL tables. Pandas allows you to load, clean, transform, and analyze data with ease. It also integrates well with other libraries, such as scikit-learn and TensorFlow, making it an essential tool for any machine learning project.
NumPy is another fundamental library for numerical computing in Python. It provides a powerful N-dimensional array object, along with a collection of functions for performing mathematical operations on arrays. NumPy's array operations are significantly faster than traditional Python lists, making it an essential library for handling large datasets. It also provides tools for linear algebra, Fourier transforms, and random number generation, making it a versatile tool for machine learning.
In conclusion, Python offers a rich ecosystem of libraries that are essential for machine learning. Scikit-learn, TensorFlow, and PyTorch provide powerful tools for building and training machine learning models. Pandas and NumPy offer efficient data manipulation and numerical computing capabilities. By familiarizing yourself with these essential libraries, you will have a solid foundation for exploring more advanced topics in machine learning. So, start your journey into Python for machine learning by mastering these essential libraries.
Python has become one of the most popular programming languages for machine learning due to its simplicity and versatility. In this section, we will explore the process of building machine learning models with Python, providing a beginner's guide to help you get started.
The first step in building a machine learning model is to gather and prepare the data. Python offers a wide range of libraries, such as Pandas and NumPy, that make data manipulation and preprocessing tasks easier. These libraries allow you to load datasets, handle missing values, and perform feature engineering, among other things.
Once the data is ready, the next step is to choose a machine learning algorithm. Python provides a vast array of libraries, such as Scikit-learn and TensorFlow, that offer a wide range of algorithms for different types of problems. These libraries make it easy to implement and train machine learning models with just a few lines of code.
Before training a model, it is essential to split the data into training and testing sets. This step helps evaluate the model's performance on unseen data and prevents overfitting. Python provides functions and classes to split the data, such as train_test_split from Scikit-learn.
After splitting the data, it's time to train the model. Python's libraries offer various algorithms, including linear regression, decision trees, and support vector machines, among others. Each algorithm has its strengths and weaknesses, so it's crucial to understand the problem at hand and choose the most appropriate one.
During the training process, the model learns from the training data by adjusting its internal parameters. Python's libraries provide functions and methods to fit the model to the training data, such as fit from Scikit-learn. The training process aims to minimize the difference between the model's predictions and the actual values in the training set.
Once the model is trained, it's time to evaluate its performance. Python offers a range of metrics, such as accuracy, precision, and recall, to assess how well the model performs on the testing data. These metrics help determine if the model is underfitting, overfitting, or performing well.
If the model's performance is not satisfactory, it may be necessary to fine-tune its parameters. Python's libraries provide functions and classes, such as GridSearchCV from Scikit-learn, that allow you to search for the best combination of hyperparameters for your model. Fine-tuning the parameters can significantly improve the model's performance.
Once the model is trained and fine-tuned, it can be used to make predictions on new, unseen data. Python's libraries provide functions and methods to apply the model to new data, such as predict from Scikit-learn. These predictions can be used for various purposes, such as making business decisions or solving real-world problems.
In conclusion, Python is an excellent choice for building machine learning models due to its simplicity and versatility. The process of building a machine learning model with Python involves gathering and preparing the data, choosing an algorithm, splitting the data, training the model, evaluating its performance, fine-tuning its parameters, and making predictions. Python's libraries offer a wide range of functions and classes that make these tasks easier and more efficient. By following this beginner's guide, you can start building your machine learning models with Python and unlock the power of data-driven decision-making.
1. What is "A Beginner's Guide to Python for Machine Learning"?
It is a guidebook aimed at beginners to learn Python programming language specifically for machine learning applications.
2. Who is the target audience for this guide?
The target audience is beginners who are interested in learning Python for machine learning purposes.
3. What topics are covered in this guide?
The guide covers the basics of Python programming, data manipulation and analysis, machine learning algorithms, and how to implement them using Python libraries such as NumPy, Pandas, and Scikit-learn.
In conclusion, "A Beginner's Guide to Python for Machine Learning" is a valuable resource for individuals who are new to both Python programming and machine learning. The guide provides a comprehensive introduction to Python, covering the basics of syntax, data types, control flow, and functions. It also delves into the fundamentals of machine learning, explaining key concepts such as supervised and unsupervised learning, regression, classification, and clustering. The guide offers practical examples and exercises to reinforce learning, making it an excellent starting point for beginners interested in Python for machine learning.