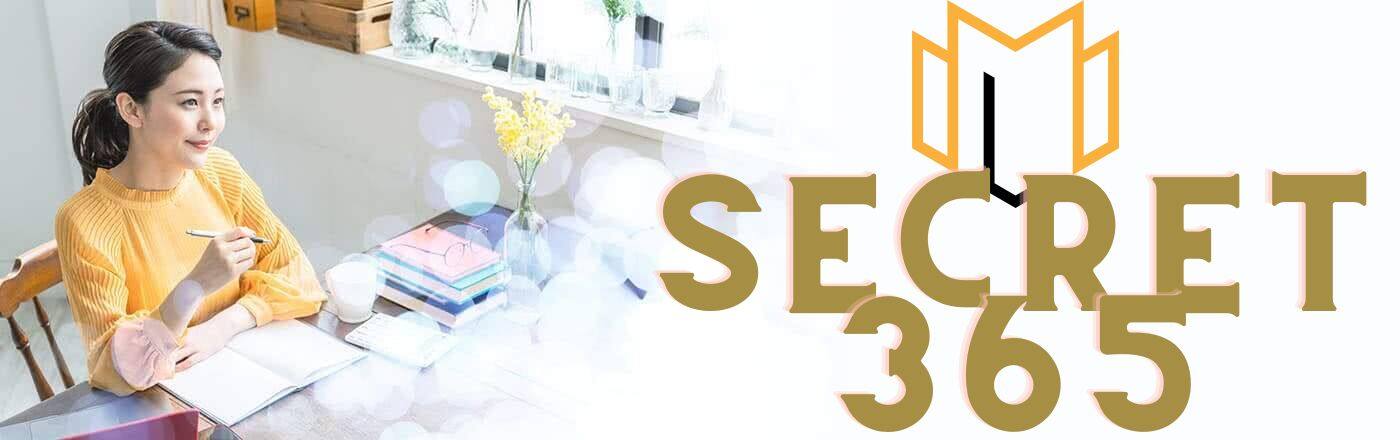
Master ES with a comprehensive walkthrough.
In this article, we will provide a comprehensive walkthrough of ECMAScript (ES) Part-2. ECMAScript is a standardized scripting language that forms the basis of JavaScript. Part-2 of ECMAScript covers additional features and enhancements introduced in newer versions of the language. This walkthrough will explore these features in detail, providing explanations and examples to help you understand and utilize them effectively. So, let's dive into the world of ECMAScript Part-2 and discover its powerful capabilities.
ECMAScript, commonly known as ES, is a standardized scripting language that forms the basis of many popular programming languages, including JavaScript. In this article, we will delve deeper into the evolution of ECMAScript, focusing on ES6 and beyond.
ES6, also known as ECMAScript 2015, was a major milestone in the development of ECMAScript. It introduced several new features and syntax enhancements that greatly improved the language's capabilities. One of the most notable additions in ES6 was the introduction of let and const keywords for variable declaration. These keywords provided block scoping, allowing developers to declare variables that are limited to a specific block of code, rather than the entire function.
Another significant enhancement in ES6 was the introduction of arrow functions. Arrow functions provide a concise syntax for writing function expressions, making the code more readable and reducing the need for explicit function binding. Additionally, arrow functions have lexical scoping of this, which means that they inherit the this value from the surrounding code.
ES6 also introduced classes, which are a syntactical sugar over the existing prototype-based inheritance model in JavaScript. Classes provide a more familiar syntax for defining objects and their behavior, making it easier for developers coming from other object-oriented languages to work with JavaScript.
In addition to these language enhancements, ES6 also introduced several new built-in objects and methods. For example, the Set and Map objects were introduced to provide efficient data structures for storing unique values and key-value pairs, respectively. The Promise object was also introduced to simplify asynchronous programming by providing a standardized way to handle asynchronous operations.
Following the release of ES6, ECMAScript adopted a yearly release cycle, with new versions being released annually. This allowed for a more rapid evolution of the language, with each new version introducing new features and improvements.
ES7, also known as ECMAScript 2016, introduced several new features, including the exponentiation operator (**), the Array.prototype.includes method, and the Object.values and Object.entries methods. These additions further enhanced the language's capabilities and made it easier for developers to write clean and concise code.
ES8, or ECMAScript 2017, introduced even more features, such as async/await, which simplified asynchronous programming by providing a more synchronous-like syntax for handling asynchronous operations. It also introduced the Object.getOwnPropertyDescriptors method, which allows developers to retrieve all property descriptors of an object.
ES9, or ECMAScript 2018, introduced several new features, including rest/spread properties for objects, asynchronous iteration, and Promise.prototype.finally method. These additions further improved the language's capabilities and made it even more powerful and flexible.
ES10, or ECMAScript 2019, introduced several new features, including Array.prototype.flat and Array.prototype.flatMap methods, optional catch binding, and the globalThis object. These additions provided developers with more tools and options for writing clean and efficient code.
In conclusion, the evolution of ECMAScript has been a continuous process, with each new version introducing new features and improvements. ES6 was a major milestone in the development of ECMAScript, introducing several new features and syntax enhancements. Subsequent versions, such as ES7, ES8, ES9, and ES10, further enhanced the language's capabilities and made it more powerful and flexible. As ECMAScript continues to evolve, it is important for developers to stay updated with the latest features and best practices to make the most out of the language.
ECMAScript (ES) is a standardized scripting language that forms the basis of JavaScript. In the previous article, we explored the fundamentals of ECMAScript and its evolution over the years. In this article, we will delve into ES modules, a powerful feature introduced in ECMAScript 6 (ES6) that allows developers to organize and import JavaScript code more efficiently.
ES modules provide a way to split JavaScript code into separate files, making it easier to manage and maintain large projects. This modular approach promotes code reusability and improves collaboration among developers. To use ES modules, we need to understand how to organize our code and import modules into our project.
To organize our code, we can create separate files for different functionalities or components of our application. For example, we can have a file for handling user authentication, another for managing database operations, and so on. This modular structure allows us to work on different parts of the application independently, making development more manageable.
To import modules into our project, we use the `import` statement followed by the module's path. The module's path can be either a relative or an absolute path. Relative paths are used to import modules within the same project, while absolute paths are used to import modules from external libraries or frameworks.
When importing a module, we can choose to import the entire module or specific parts of it. To import the entire module, we use the `import` statement followed by the module's path. For example, `import * as myModule from './myModule.js'` imports the entire module named `myModule` from the file `myModule.js`. We can then access the exported functionalities of the module using the `myModule` object.
Alternatively, we can import specific functionalities from a module using the `import` statement followed by the module's path and the specific functionality we want to import. For example, `import { myFunction } from './myModule.js'` imports only the `myFunction` from the module `myModule.js`. We can then directly use the imported functionality without accessing it through an object.
ES modules also support default exports, which allow us to export a single functionality as the default export of a module. To import the default export, we use the `import` statement followed by the module's path and assign it to a variable. For example, `import myFunction from './myModule.js'` imports the default export of `myModule.js` and assigns it to the variable `myFunction`. We can then use `myFunction` directly without accessing it through an object.
In addition to importing modules, we can also export functionalities from our own modules. To export a functionality, we use the `export` keyword followed by the functionality we want to export. For example, `export function myFunction() { ... }` exports the function `myFunction` from the current module. We can then import this functionality in other modules using the `import` statement.
ES modules provide a powerful mechanism for organizing and importing JavaScript code. By splitting our code into separate modules, we can improve code maintainability and reusability. With the ability to import specific functionalities or the entire module, we have fine-grained control over what parts of a module we want to use. Whether you are working on a small project or a large-scale application, mastering ES modules is essential for efficient JavaScript development.
In conclusion, ES modules are a valuable addition to ECMAScript that allows developers to organize and import JavaScript code more effectively. By understanding how to organize our code into modules and import them into our projects, we can improve code organization, collaboration, and reusability. With the power of ES modules, JavaScript development becomes more manageable and efficient.
ECMAScript (ES) is a standardized scripting language that forms the foundation of JavaScript. In the previous article, we delved into the basics of ECMAScript and its core features. Now, let's take a step further and explore some of the advanced features that ES has to offer: Promises, Generators, and Async/Await.
Promises are a powerful addition to JavaScript that allow us to handle asynchronous operations more efficiently. In the past, callbacks were commonly used for asynchronous programming, but they often led to callback hell, making the code difficult to read and maintain. Promises provide a more structured approach to handling asynchronous tasks.
A Promise represents the eventual completion or failure of an asynchronous operation and allows us to attach callbacks to handle the result. It has three states: pending, fulfilled, or rejected. When a Promise is pending, it means that the asynchronous operation is still in progress. Once the operation is completed successfully, the Promise is fulfilled, and if an error occurs, it is rejected.
Generators, on the other hand, introduce a new way of writing functions in JavaScript. They allow us to pause and resume the execution of a function, making it possible to write more readable and maintainable code. Generators are defined using the function* syntax and yield statements.
When a generator function is called, it returns an iterator object. We can then use the iterator's next() method to control the execution of the generator function. Each time we call next(), the generator function resumes its execution until it encounters a yield statement. The value yielded by the yield statement is returned by the iterator's next() method.
Async/Await is a syntax sugar built on top of Promises, making asynchronous code look and behave more like synchronous code. It allows us to write asynchronous code in a more sequential and readable manner, without the need for callbacks or chaining promises.
To use async/await, we need to define an async function. Inside this function, we can use the await keyword to pause the execution until a Promise is fulfilled or rejected. The await keyword can only be used inside an async function.
By using async/await, we can avoid the pyramid of doom that often occurs with nested callbacks or chained promises. Instead, we can write our asynchronous code in a linear and intuitive way, making it easier to understand and maintain.
One important thing to note is that async/await is not supported in all browsers and environments. To use it, we need to transpile our code using a tool like Babel or use a runtime that supports async/await, such as Node.js.
In conclusion, ECMAScript (ES) offers advanced features like Promises, Generators, and Async/Await that enhance the capabilities of JavaScript. Promises provide a structured approach to handle asynchronous operations, while Generators allow us to write more readable and maintainable code by pausing and resuming the execution of functions. Async/Await, built on top of Promises, simplifies asynchronous code by making it look and behave more like synchronous code. By understanding and utilizing these advanced features, we can write more efficient and maintainable JavaScript code.
1. What is ECMAScript (ES)?
ECMAScript (ES) is a standardized scripting language specification that forms the basis for JavaScript. It defines the syntax, semantics, and behavior of the language.
2. What does Part-2 of the ECMAScript (ES) walkthrough cover?
Part-2 of the ECMAScript (ES) walkthrough covers various advanced topics such as modules, classes, iterators, generators, and promises.
3. Why is understanding ECMAScript (ES) important for JavaScript developers?
Understanding ECMAScript (ES) is important for JavaScript developers as it allows them to leverage the latest features and enhancements in the language. It helps developers write more efficient, maintainable, and modern JavaScript code.
In conclusion, Part-2 of A Comprehensive Walkthrough of ECMAScript (ES) provides a detailed understanding of various important concepts and features of ECMAScript. It covers topics such as arrow functions, default parameters, rest and spread operators, destructuring assignment, classes, modules, and more. This comprehensive guide serves as a valuable resource for developers looking to enhance their knowledge and proficiency in ECMAScript programming.