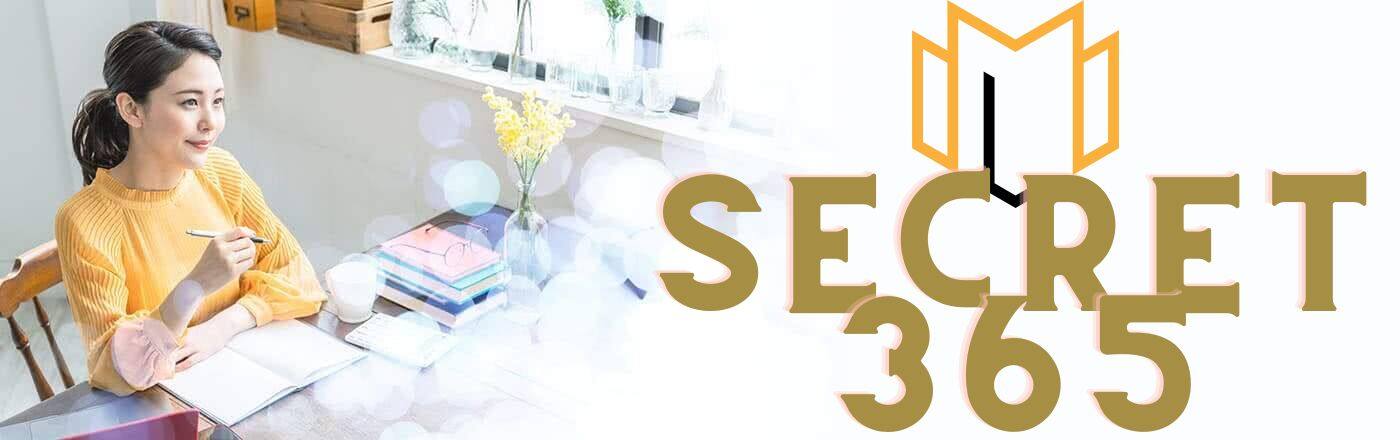
Mastering Data Structures and Algorithms in 45 Days: Unlock the Power of Efficient Problem Solving.
Mastering Data Structures and Algorithms in 45 Days is a comprehensive guide designed to help individuals develop a strong foundation in data structures and algorithms within a relatively short timeframe. This book provides a structured approach to learning, with a focus on practical implementation and problem-solving techniques. By following the suggested study plan and completing the exercises and coding challenges, readers can enhance their understanding and proficiency in this crucial area of computer science. Whether you are a beginner or an experienced programmer looking to refresh your knowledge, this book offers a concise and effective path to mastering data structures and algorithms in just 45 days.
Mastering Data Structures and Algorithms in 45 Days
Data structures and algorithms are fundamental concepts in computer science and play a crucial role in solving complex problems efficiently. Whether you are a beginner or an experienced programmer, understanding these concepts is essential for writing efficient and optimized code. In this article, we will provide an introduction to data structures and algorithms and discuss how you can master them in just 45 days.
To begin with, let's define what data structures and algorithms are. Data structures are containers that hold and organize data in a specific way, allowing for efficient storage, retrieval, and manipulation of data. On the other hand, algorithms are step-by-step procedures or instructions for solving a specific problem. They utilize data structures to perform operations on the data.
Now that we have a basic understanding of data structures and algorithms, let's delve into some commonly used data structures. One of the most fundamental data structures is an array, which is a collection of elements stored in contiguous memory locations. Arrays are widely used due to their simplicity and constant-time access to elements. Another commonly used data structure is a linked list, where each element contains a reference to the next element, forming a chain-like structure. Linked lists are efficient for insertion and deletion operations but have slower access times compared to arrays.
Moving on, let's explore some essential algorithms. One such algorithm is the sorting algorithm, which arranges elements in a specific order. There are various sorting algorithms, such as bubble sort, insertion sort, and quicksort, each with its own advantages and disadvantages. Another important algorithm is the searching algorithm, which looks for a specific element within a collection of data. Binary search is a commonly used searching algorithm that efficiently finds an element in a sorted array.
Now that we have covered the basics of data structures and algorithms, let's discuss how you can master them in just 45 days. The key to mastering these concepts lies in a structured and consistent approach. Start by dedicating a specific amount of time each day to study and practice. Set realistic goals for each day, focusing on understanding and implementing different data structures and algorithms.
Begin by studying the theory behind each data structure and algorithm. Understand their properties, advantages, and disadvantages. Once you have a solid theoretical foundation, move on to implementing them in a programming language of your choice. Practice writing code for different operations on each data structure and algorithm. This hands-on experience will help solidify your understanding and improve your problem-solving skills.
Additionally, make use of online resources, such as tutorials, videos, and coding platforms, to enhance your learning experience. Participate in coding challenges and competitions to test your skills and gain practical exposure. Collaborate with fellow programmers and discuss different approaches and solutions to problems. This will broaden your perspective and help you learn from others' experiences.
In conclusion, data structures and algorithms are essential concepts in computer science that every programmer should master. By dedicating focused time each day, studying the theory, implementing them in code, and practicing regularly, you can become proficient in data structures and algorithms in just 45 days. Remember, consistency and perseverance are key to mastering these concepts. So, start your journey today and unlock the power of efficient problem-solving through data structures and algorithms.
Mastering Data Structures and Algorithms in 45 Days
Data structures and algorithms are fundamental concepts in computer science. They form the backbone of efficient and optimized software development. Whether you are a beginner or an experienced programmer, understanding and mastering these concepts is crucial for solving complex problems and creating robust applications. In this article, we will explore the essential data structures that are commonly used in algorithms and how they contribute to efficient programming.
One of the most basic and widely used data structures is the array. An array is a collection of elements of the same type, stored in contiguous memory locations. It allows for efficient access to elements using their indices. Arrays are particularly useful when the size of the collection is known in advance and when random access to elements is required. However, arrays have a fixed size, which means that adding or removing elements can be costly in terms of time and memory.
To overcome the limitations of arrays, linked lists are often used. A linked list is a collection of nodes, where each node contains a value and a reference to the next node. Unlike arrays, linked lists can dynamically grow and shrink, making them more flexible. However, accessing elements in a linked list is slower compared to arrays, as it requires traversing the list from the beginning.
Another important data structure is the stack. A stack is a Last-In-First-Out (LIFO) data structure, where elements are added and removed from the top. It follows the principle of "last in, first out," similar to a stack of plates. Stacks are commonly used in algorithms that require backtracking or maintaining a history of operations. They are also used in implementing recursive algorithms and parsing expressions.
On the other hand, a queue is a First-In-First-Out (FIFO) data structure. Elements are added at the rear and removed from the front. Queues are useful in scenarios where the order of processing is important, such as scheduling tasks or handling requests. They can be implemented using arrays or linked lists, depending on the specific requirements of the application.
Binary trees are another essential data structure for efficient algorithms. A binary tree is a hierarchical structure where each node has at most two children, referred to as the left child and the right child. Binary trees are used in various algorithms, such as searching, sorting, and traversing. They provide efficient access and manipulation of data, especially when the data is sorted or partially sorted.
Graphs are yet another crucial data structure in algorithms. A graph is a collection of nodes, also known as vertices, connected by edges. Graphs are used to model relationships between objects or entities. They are widely used in various applications, including social networks, routing algorithms, and recommendation systems. Graphs can be represented using adjacency matrices or adjacency lists, depending on the specific requirements of the problem.
In conclusion, mastering data structures is essential for efficient algorithm design and implementation. The array, linked list, stack, queue, binary tree, and graph are some of the fundamental data structures that every programmer should be familiar with. Each data structure has its own advantages and trade-offs, and understanding when and how to use them is crucial for solving complex problems. By gaining a deep understanding of these data structures and their associated algorithms, you can become a more proficient programmer and tackle challenging problems with ease. So, start your journey to mastering data structures and algorithms today!
Mastering Data Structures and Algorithms in 45 Days
Advanced Algorithms and Optimization Techniques
Data structures and algorithms are fundamental concepts in computer science and play a crucial role in solving complex problems efficiently. As a programmer, it is essential to have a strong understanding of these concepts to excel in the field. In this section, we will explore advanced algorithms and optimization techniques that will help you master data structures and algorithms in just 45 days.
One of the key topics in advanced algorithms is graph algorithms. Graphs are widely used to represent relationships between objects, and understanding how to traverse and manipulate them efficiently is vital. Some popular graph algorithms include breadth-first search (BFS) and depth-first search (DFS), which allow you to explore a graph and find the shortest path between two nodes. Additionally, algorithms like Dijkstra's algorithm and Bellman-Ford algorithm help find the shortest path in weighted graphs.
Another important concept in advanced algorithms is dynamic programming. Dynamic programming is a technique used to solve problems by breaking them down into smaller overlapping subproblems. By solving these subproblems and storing their solutions, you can avoid redundant computations and improve the overall efficiency of your algorithm. Understanding dynamic programming will enable you to solve a wide range of optimization problems efficiently.
In addition to graph algorithms and dynamic programming, advanced algorithms also include techniques like divide and conquer and greedy algorithms. Divide and conquer is a problem-solving technique that involves breaking down a problem into smaller subproblems, solving them independently, and then combining their solutions to obtain the final result. Greedy algorithms, on the other hand, make locally optimal choices at each step to find the overall optimal solution. These techniques are widely used in various applications, such as sorting algorithms (e.g., merge sort and quicksort) and searching algorithms (e.g., binary search).
To master these advanced algorithms and optimization techniques in just 45 days, it is crucial to follow a structured learning plan. Start by familiarizing yourself with the basic concepts and principles behind each algorithm. Understand how they work and their time and space complexities. Once you have a solid foundation, move on to implementing these algorithms in your preferred programming language. Practice coding them from scratch and analyze their performance on different inputs.
Additionally, solving a variety of algorithmic problems is essential to reinforce your understanding and improve your problem-solving skills. Online platforms like LeetCode, HackerRank, and Codeforces offer a vast collection of algorithmic problems to solve. Start with easier problems and gradually move on to more challenging ones. Analyze the solutions of others and learn from their approaches. This iterative process of solving problems and learning from others will help you gain confidence and expertise in advanced algorithms.
Furthermore, it is crucial to stay updated with the latest research and advancements in the field of algorithms. Subscribe to newsletters, follow influential researchers and organizations on social media, and participate in online forums and communities. Engaging with the algorithmic community will expose you to new ideas, techniques, and problem-solving approaches.
In conclusion, mastering data structures and algorithms in just 45 days requires a structured learning plan and consistent practice. Advanced algorithms and optimization techniques, such as graph algorithms, dynamic programming, divide and conquer, and greedy algorithms, are essential to solving complex problems efficiently. By following a step-by-step approach, implementing algorithms, solving problems, and staying updated with the latest research, you can become proficient in these concepts within the given timeframe. Remember, practice makes perfect, and with dedication and perseverance, you can become a master of data structures and algorithms.
1. What is the book "Mastering Data Structures and Algorithms in 45 Days" about?
The book is a comprehensive guide that aims to help readers understand and master various data structures and algorithms within a 45-day timeframe.
2. Who is the author of "Mastering Data Structures and Algorithms in 45 Days"?
The author of the book is Hemant Jain.
3. What is the intended audience for "Mastering Data Structures and Algorithms in 45 Days"?
The book is primarily targeted towards programmers, software engineers, and computer science students who want to enhance their understanding and proficiency in data structures and algorithms.
Mastering Data Structures and Algorithms in 45 Days is a comprehensive and practical guide that provides a structured approach to learning and understanding these fundamental concepts. The book covers a wide range of data structures and algorithms, explaining their implementation and analyzing their time and space complexities. With a clear and concise writing style, it offers step-by-step explanations and examples to help readers grasp the concepts effectively. The 45-day timeline provides a systematic plan for studying and practicing these concepts, making it suitable for both beginners and experienced programmers. Overall, this book is a valuable resource for anyone looking to enhance their understanding and proficiency in data structures and algorithms.