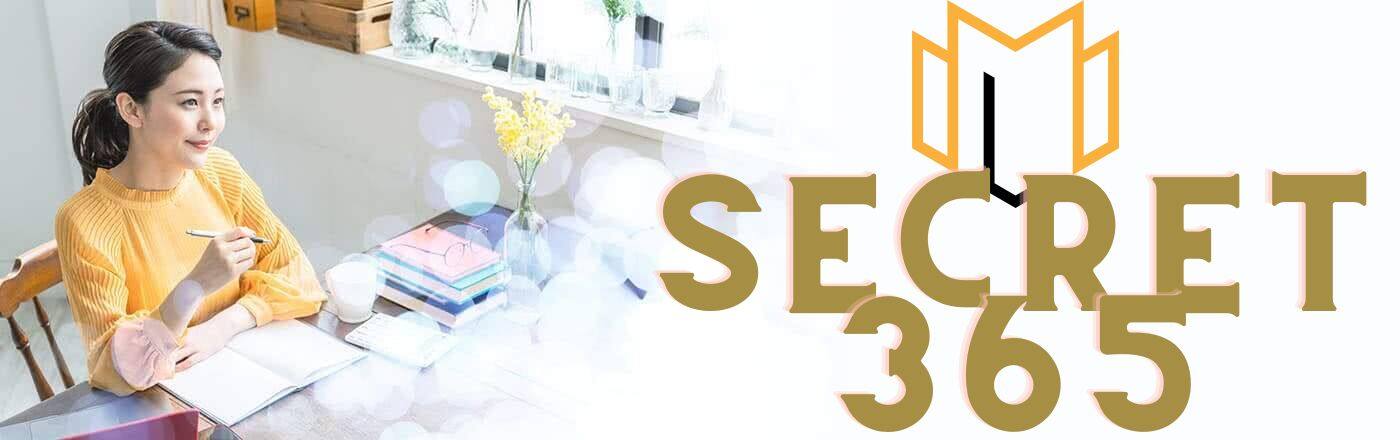
"Efficiency at its core: Experience seamless task execution with First Come First Serve CPU Scheduling Algorithm in Java."
The First Come First Serve (FCFS) CPU Scheduling Algorithm is a simple scheduling algorithm used in operating systems. It works on the principle of serving the processes in the order they arrive. In this algorithm, the process that arrives first gets executed first. This article will discuss the implementation of the FCFS CPU Scheduling Algorithm in Java.
The First Come First Serve (FCFS) CPU Scheduling Algorithm is one of the simplest and most straightforward algorithms used in operating systems. It is a non-preemptive algorithm, meaning that once a process is allocated the CPU, it will continue to run until it completes or voluntarily releases the CPU. In this article, we will discuss the implementation of the FCFS CPU Scheduling Algorithm in Java.
To implement the FCFS algorithm, we need to consider a few key components. First, we need a data structure to represent the processes. We can create a Process class that contains attributes such as process ID, arrival time, burst time, and completion time. We can also include methods to calculate the waiting time and turnaround time for each process.
Next, we need to create a queue to hold the processes. In Java, we can use the LinkedList class to implement a queue. The LinkedList class provides methods such as add(), remove(), and peek() that allow us to add processes to the end of the queue, remove processes from the front of the queue, and retrieve the first process in the queue, respectively.
Once we have our data structure and queue set up, we can start implementing the FCFS algorithm. The first step is to sort the processes based on their arrival time. We can use the Collections.sort() method in Java to sort the processes in ascending order of arrival time.
After sorting the processes, we can iterate through the queue and allocate the CPU to each process in the order they arrived. To simulate the execution of the processes, we can use a loop that runs until the queue is empty. In each iteration of the loop, we remove the first process from the queue, update its completion time, waiting time, and turnaround time, and then move on to the next process.
To calculate the waiting time and turnaround time for each process, we need to keep track of the current time. We can initialize a variable called currentTime to 0 before starting the loop. For each process, we can calculate its waiting time by subtracting its arrival time from the current time. We can then update the current time by adding the process's burst time. The turnaround time can be calculated by adding the burst time and waiting time.
Once we have processed all the processes, we can calculate the average waiting time and average turnaround time by summing up the waiting times and turnaround times of all processes and dividing them by the total number of processes.
In conclusion, the FCFS CPU Scheduling Algorithm is a simple yet effective algorithm for allocating the CPU to processes in the order they arrive. By implementing this algorithm in Java, we can simulate the execution of processes and calculate important metrics such as waiting time and turnaround time. The FCFS algorithm serves as a foundation for more complex scheduling algorithms and provides a starting point for understanding the concepts of CPU scheduling in operating systems.
The First Come First Serve (FCFS) CPU scheduling algorithm is one of the simplest and most straightforward algorithms used in operating systems. It is a non-preemptive algorithm, meaning that once a process is allocated the CPU, it will continue to run until it completes or voluntarily gives up the CPU. In this article, we will discuss the step-by-step implementation of the FCFS CPU scheduling algorithm in Java.
To begin with, we need to define a Process class that represents a process in our system. This class should have attributes such as process ID, arrival time, burst time, and completion time. We also need to define a constructor to initialize these attributes.
Next, we need to create a list of processes that will be scheduled by our algorithm. We can use an ArrayList to store these processes. We can either hardcode the processes or take user input to dynamically create them.
Once we have our list of processes, we need to sort them based on their arrival time. This step is crucial as the FCFS algorithm schedules processes based on their arrival time. We can use the Collections.sort() method in Java to sort our list of processes.
After sorting the processes, we can start implementing the FCFS algorithm. We need to create a variable called currentTime to keep track of the current time. We also need to create a variable called totalWaitingTime to calculate the total waiting time of all processes.
We can iterate over our list of processes and for each process, we can calculate its waiting time by subtracting its arrival time from the current time. We can then update the current time by adding the burst time of the current process.
To calculate the completion time of each process, we can add the burst time to the waiting time. We can also update the total waiting time by adding the waiting time of the current process.
Once we have calculated the waiting time and completion time for each process, we can print out the results. We can iterate over our list of processes and for each process, we can print its process ID, arrival time, burst time, waiting time, and completion time.
Finally, we can calculate the average waiting time by dividing the total waiting time by the number of processes. We can then print out the average waiting time to provide a measure of the efficiency of our FCFS algorithm.
In conclusion, the FCFS CPU scheduling algorithm is a simple yet effective algorithm used in operating systems. By following the step-by-step implementation in Java, we can schedule processes based on their arrival time and calculate their waiting and completion times. This algorithm provides a basic understanding of how CPU scheduling works and can serve as a foundation for more complex scheduling algorithms.
Performance Analysis and Comparison of FCFS CPU Scheduling Algorithm in Java
In the world of computer science, CPU scheduling algorithms play a crucial role in determining the efficiency and performance of a system. One such algorithm is the First Come First Serve (FCFS) CPU scheduling algorithm. In this article, we will explore the implementation of the FCFS algorithm in Java and analyze its performance.
To begin with, let's understand the basic concept behind the FCFS algorithm. As the name suggests, this algorithm assigns the CPU to the processes in the order they arrive. The first process that arrives is the first one to be executed, and subsequent processes are executed in the order of their arrival. This simple and intuitive approach makes FCFS one of the easiest scheduling algorithms to implement.
Now, let's delve into the implementation of the FCFS algorithm in Java. To simulate the CPU scheduling, we can create a Process class that represents a process with attributes such as arrival time, burst time, and completion time. We can then create a list of processes and sort them based on their arrival time using the Collections.sort() method.
Once the processes are sorted, we can iterate through the list and calculate the completion time for each process. The completion time of a process is the sum of its burst time and the completion time of the previous process. We can store the completion time in a separate array for further analysis.
After calculating the completion time for all processes, we can calculate the waiting time and turnaround time. The waiting time of a process is the difference between its completion time and its burst time, while the turnaround time is the difference between the completion time and the arrival time. We can then calculate the average waiting time and average turnaround time by summing up the respective values and dividing by the number of processes.
Now that we have implemented the FCFS algorithm in Java, let's analyze its performance. The FCFS algorithm is known for its simplicity and fairness, as it ensures that every process gets a fair share of the CPU. However, it suffers from a major drawback known as the "convoy effect." In this effect, a long process that arrives early can hold up the CPU, causing shorter processes to wait for a longer time.
To compare the performance of the FCFS algorithm, we can implement other scheduling algorithms such as Shortest Job Next (SJN) or Round Robin (RR) and analyze their results. SJN aims to minimize the waiting time by executing the shortest job first, while RR assigns a fixed time quantum to each process, allowing them to take turns executing.
By comparing the average waiting time and average turnaround time of different scheduling algorithms, we can determine which algorithm performs better in terms of efficiency and fairness. It is important to note that the performance of a scheduling algorithm may vary depending on the characteristics of the processes and the workload of the system.
In conclusion, the implementation of the FCFS CPU scheduling algorithm in Java provides a simple and intuitive approach to scheduling processes. While it ensures fairness, it may suffer from the convoy effect, which can impact the overall performance of the system. By analyzing and comparing the performance of different scheduling algorithms, we can make informed decisions on which algorithm to use in different scenarios.
1. How can the First Come First Serve (FCFS) CPU scheduling algorithm be implemented in Java?
- The FCFS algorithm can be implemented in Java by maintaining a queue data structure to hold the processes. The processes are added to the queue in the order they arrive, and the CPU executes them one by one in the same order.
2. What is the basic logic behind the FCFS CPU scheduling algorithm?
- The basic logic of the FCFS algorithm is to execute the processes in the order they arrive. The CPU starts executing the first process in the queue, and once it completes, it moves on to the next process in the queue.
3. What are the advantages and disadvantages of the FCFS CPU scheduling algorithm?
- Advantages: It is simple to understand and implement, and there is no starvation as every process gets a chance to execute.
- Disadvantages: It may result in poor average waiting time, especially if long processes arrive first (known as the "convoy effect"). It does not consider the burst time or priority of processes, which can lead to inefficient utilization of CPU resources.
In conclusion, the First Come First Serve (FCFS) CPU scheduling algorithm has been successfully implemented in Java. This algorithm operates by executing processes in the order they arrive, without considering their burst time or priority. It is a simple and easy-to-understand algorithm, but it may lead to longer waiting times for processes with longer burst times. The implementation in Java involves maintaining a queue data structure to store the processes and executing them one by one in the order they are added to the queue. Overall, the FCFS algorithm can be a suitable choice for certain scenarios where fairness and simplicity are prioritized over efficiency.