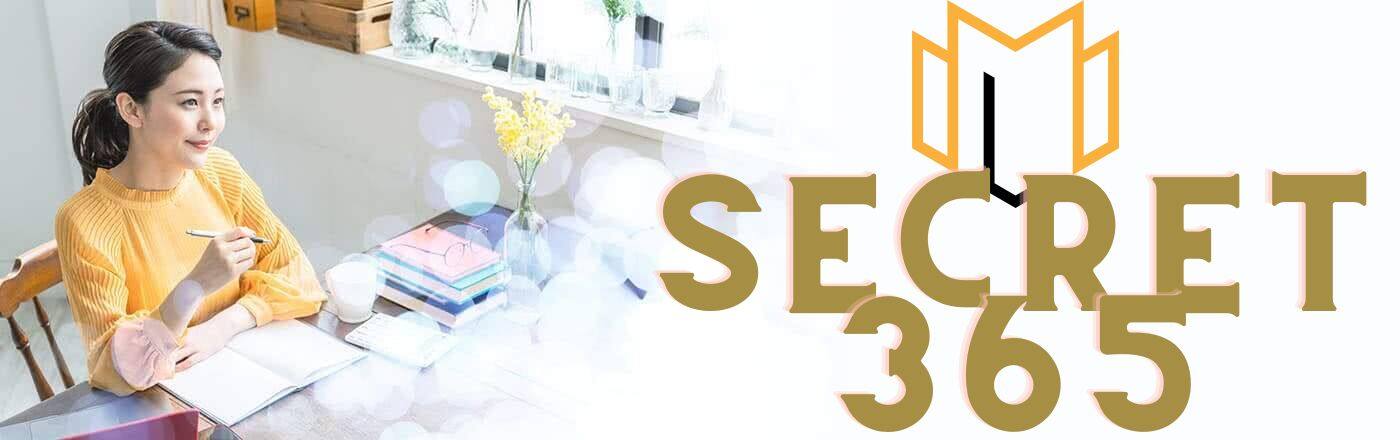
Unleash the power of combinations in programming.
An Introduction to Combinatorial Mathematics in Programming is a field of study that focuses on the application of combinatorial mathematics principles and techniques in computer programming. It involves the analysis and manipulation of discrete objects and structures, such as permutations, combinations, graphs, and networks, to solve complex problems in various domains. This introduction provides an overview of the fundamental concepts and methodologies used in combinatorial mathematics and highlights its significance in programming.
Combinatorial mathematics is a branch of mathematics that deals with the study of combinations, permutations, and arrangements of objects. In the field of programming, combinatorial mathematics plays a crucial role in solving complex problems and optimizing algorithms. By understanding the basics of combinatorial mathematics, programmers can develop more efficient and effective solutions to various computational problems.
One fundamental concept in combinatorial mathematics is the notion of permutations. A permutation is an arrangement of objects in a specific order. For example, given a set of three objects {A, B, C}, the possible permutations are ABC, ACB, BAC, BCA, CAB, and CBA. The number of permutations of a set of n objects is given by n factorial, denoted as n!. For instance, the number of permutations of a set of three objects is 3! = 3 x 2 x 1 = 6.
Another important concept in combinatorial mathematics is combinations. A combination is a selection of objects without regard to the order in which they are arranged. For instance, if we have a set of three objects {A, B, C}, the possible combinations are {A}, {B}, {C}, {A, B}, {A, C}, and {B, C}. The number of combinations of a set of n objects taken r at a time is given by the binomial coefficient, denoted as nCr. The formula for calculating the binomial coefficient is n! / (r! * (n - r)!). For example, the number of combinations of a set of three objects taken two at a time is 3! / (2! * (3 - 2)!) = 3.
Combinatorial mathematics also involves the study of arrangements. An arrangement is a permutation of a subset of objects taken from a larger set. For instance, if we have a set of four objects {A, B, C, D}, the possible arrangements of three objects taken at a time are ABC, ABD, ACD, BCD. The number of arrangements of a set of n objects taken r at a time is given by nPr, which is calculated as n! / (n - r)!. In the example above, the number of arrangements of a set of four objects taken three at a time is 4! / (4 - 3)! = 4.
In programming, combinatorial mathematics is often used to solve problems related to permutations, combinations, and arrangements. For instance, in a scenario where a program needs to generate all possible combinations of a set of elements, combinatorial mathematics provides the necessary tools to accomplish this task efficiently. By utilizing the formulas and algorithms derived from combinatorial mathematics, programmers can develop algorithms that generate all possible combinations without having to explicitly list them.
Furthermore, combinatorial mathematics is also employed in optimizing algorithms. By understanding the principles of combinatorial mathematics, programmers can identify patterns and structures within a problem and devise algorithms that exploit these patterns to achieve better performance. For example, in the field of graph theory, combinatorial mathematics is used to find the shortest path between two nodes in a graph, which is a fundamental problem in many applications.
In conclusion, combinatorial mathematics is a vital tool in programming that enables programmers to solve complex problems and optimize algorithms. By understanding the basics of permutations, combinations, and arrangements, programmers can develop more efficient and effective solutions to various computational problems. Combinatorial mathematics provides the necessary tools and techniques to generate all possible combinations, identify patterns, and optimize algorithms. Therefore, a solid understanding of combinatorial mathematics is essential for any programmer seeking to excel in the field of programming.
Applications of Combinatorial Mathematics in Programming
Combinatorial mathematics is a branch of mathematics that deals with the study of combinations, permutations, and arrangements of objects. It has numerous applications in various fields, including computer programming. In this article, we will explore some of the key applications of combinatorial mathematics in programming.
One of the most common applications of combinatorial mathematics in programming is in the field of algorithm design. Algorithms are step-by-step procedures used to solve problems or perform specific tasks. Combinatorial mathematics provides programmers with the tools and techniques to design efficient algorithms for a wide range of problems.
For example, in the field of network optimization, combinatorial mathematics is used to design algorithms that find the shortest path between two points in a network. This is a fundamental problem in computer science and has applications in various domains, such as transportation and logistics.
Another application of combinatorial mathematics in programming is in the field of cryptography. Cryptography is the practice of securing communication by converting information into a form that is unreadable to unauthorized users. Combinatorial mathematics plays a crucial role in designing encryption algorithms that are resistant to attacks.
In cryptography, combinatorial mathematics is used to design algorithms that generate secure keys for encryption and decryption. These algorithms rely on the properties of combinatorial structures, such as permutations and combinations, to ensure the security of the encrypted data.
Combinatorial mathematics also finds applications in the field of data mining and machine learning. Data mining is the process of discovering patterns and relationships in large datasets, while machine learning is the field of study that gives computers the ability to learn without being explicitly programmed.
In data mining, combinatorial mathematics is used to design algorithms that efficiently search for patterns in large datasets. These algorithms use combinatorial techniques, such as association rules and frequent itemsets, to identify interesting patterns and relationships in the data.
In machine learning, combinatorial mathematics is used to design algorithms that learn from data and make predictions or decisions. Combinatorial optimization algorithms, such as genetic algorithms and simulated annealing, are used to find optimal solutions to complex problems in machine learning.
Combinatorial mathematics also has applications in the field of computer graphics and image processing. Computer graphics is the field of study that deals with the creation, manipulation, and rendering of images and visual content. Image processing, on the other hand, is the field of study that deals with the analysis and manipulation of digital images.
In computer graphics, combinatorial mathematics is used to design algorithms that generate realistic and visually appealing images. These algorithms use combinatorial techniques, such as ray tracing and polygonal mesh generation, to model and render complex scenes.
In image processing, combinatorial mathematics is used to design algorithms that enhance and analyze digital images. These algorithms use combinatorial techniques, such as image segmentation and feature extraction, to extract meaningful information from images.
In conclusion, combinatorial mathematics plays a vital role in various applications in programming. From algorithm design to cryptography, data mining to machine learning, and computer graphics to image processing, combinatorial mathematics provides programmers with the tools and techniques to solve complex problems efficiently. As technology continues to advance, the importance of combinatorial mathematics in programming will only continue to grow.
Combinatorial mathematics is a branch of mathematics that deals with counting, arranging, and selecting objects. It plays a crucial role in programming, as it provides techniques to solve complex problems efficiently. In this article, we will introduce you to the basics of combinatorial mathematics and explore some advanced techniques used in programming.
At its core, combinatorial mathematics is concerned with counting the number of ways to arrange or select objects. This can be useful in various programming scenarios, such as generating permutations, combinations, or subsets of a given set. By understanding the principles of combinatorial mathematics, programmers can optimize their algorithms and improve the efficiency of their code.
One fundamental concept in combinatorial mathematics is the factorial. The factorial of a non-negative integer n, denoted as n!, is the product of all positive integers less than or equal to n. For example, 5! is equal to 5 × 4 × 3 × 2 × 1, which is 120. Factorials are often used to calculate the number of permutations or arrangements of a set of objects.
Permutations are arrangements of objects in a specific order. For example, given the set {A, B, C}, the permutations would be ABC, ACB, BAC, BCA, CAB, and CBA. The number of permutations of a set with n objects is given by n!. In programming, generating permutations can be useful in various scenarios, such as solving puzzles or finding all possible orderings of a set of elements.
Combinations, on the other hand, are selections of objects without considering their order. For example, given the set {A, B, C}, the combinations would be AB, AC, and BC. The number of combinations of a set with n objects taken r at a time is given by n! / (r! × (n - r)!). Combinations are often used in programming to solve problems that involve selecting a subset of elements from a larger set.
Another important concept in combinatorial mathematics is the binomial coefficient. The binomial coefficient, denoted as nCr, represents the number of combinations of n objects taken r at a time. It can be calculated using the formula n! / (r! × (n - r)!). Binomial coefficients are used in various programming scenarios, such as calculating probabilities, generating Pascal's triangle, or solving problems related to combinations.
In programming, combinatorial mathematics can be applied to solve a wide range of problems. For example, it can be used to generate all possible combinations of a set of elements, find the shortest path in a graph, or optimize the allocation of resources. By leveraging the principles of combinatorial mathematics, programmers can develop efficient algorithms and improve the performance of their code.
In conclusion, combinatorial mathematics is a powerful tool in programming that allows programmers to solve complex problems efficiently. By understanding the principles of combinatorial mathematics, programmers can generate permutations, combinations, and subsets of objects, optimize algorithms, and improve the efficiency of their code. Whether it's solving puzzles, calculating probabilities, or optimizing resource allocation, combinatorial mathematics provides valuable techniques for programmers to tackle a wide range of programming challenges.
1. What is combinatorial mathematics in programming?
Combinatorial mathematics in programming involves the study and application of mathematical techniques to solve problems related to combinations, permutations, and other combinatorial structures in computer programming.
2. Why is combinatorial mathematics important in programming?
Combinatorial mathematics provides tools and techniques to efficiently solve problems involving combinations, permutations, and other combinatorial structures. It helps in optimizing algorithms, designing efficient data structures, and solving complex optimization problems in various domains of programming.
3. What are some practical applications of combinatorial mathematics in programming?
Combinatorial mathematics finds applications in various areas of programming, such as cryptography, network optimization, scheduling, data compression, artificial intelligence, and algorithm design. It is used to solve problems like finding the shortest path in a graph, optimizing resource allocation, generating permutations or combinations, and solving constraint satisfaction problems.
In conclusion, combinatorial mathematics plays a crucial role in programming by providing techniques and tools to solve complex problems involving combinations, permutations, and arrangements. It helps programmers optimize algorithms, analyze data structures, and design efficient solutions. Understanding the principles of combinatorial mathematics can greatly enhance a programmer's ability to tackle challenging programming tasks and improve the overall efficiency and effectiveness of their programs.