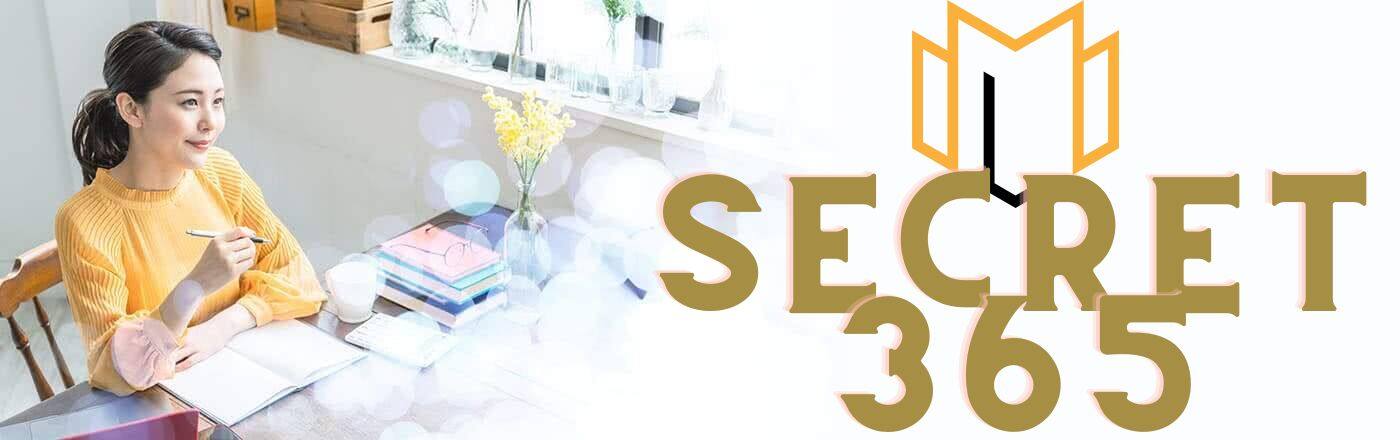
"Unlock the power of organized data with Array in Data Structures: Part 1."
Introduction:
An array is a fundamental data structure in computer programming that allows us to store and manipulate a collection of elements of the same type. It provides a way to organize and access data efficiently by assigning a unique index to each element in the array. Arrays are widely used in various algorithms and applications due to their simplicity and versatility. In this two-part series, we will explore the concept of arrays in data structures, covering their properties, operations, and common use cases.
An array is a fundamental data structure in computer science that allows us to store and access a collection of elements. It is a linear data structure that consists of a fixed-size sequence of elements of the same type. In this article, we will explore the basics of arrays in data structures.
Arrays are widely used in programming because of their simplicity and efficiency. They provide a way to store multiple values of the same type in a contiguous block of memory. This allows for efficient access to elements using their indices.
One of the key characteristics of arrays is their fixed size. Once an array is created, its size cannot be changed. This means that we need to know the number of elements we want to store in advance. If we need to store more elements than the array can accommodate, we would need to create a new array with a larger size and copy the existing elements into it.
Arrays can be used to store various types of data, such as integers, floating-point numbers, characters, or even objects. The elements in an array are typically accessed using their indices, which start from 0 and go up to the size of the array minus one. For example, in an array of size 5, the indices would range from 0 to 4.
Accessing elements in an array is a constant-time operation, meaning that it takes the same amount of time regardless of the size of the array. This is because the memory locations of the elements are contiguous, and we can calculate the address of any element using simple arithmetic.
Arrays also provide efficient random access to elements. Given an index, we can directly access the corresponding element without having to traverse the entire array. This makes arrays suitable for scenarios where we need to access elements in a non-sequential manner.
Another important concept related to arrays is the concept of multidimensional arrays. A multidimensional array is an array of arrays, where each element can be accessed using multiple indices. For example, a two-dimensional array can be visualized as a table with rows and columns. Each element in the table can be accessed using two indices, one for the row and one for the column.
In addition to accessing elements, arrays also support various operations such as insertion, deletion, and searching. However, these operations can be less efficient compared to accessing elements. Inserting or deleting an element in the middle of an array requires shifting all the subsequent elements, which can be time-consuming for large arrays.
In conclusion, arrays are a fundamental data structure in computer science that allow us to store and access a collection of elements. They provide efficient random access to elements and are widely used in programming. Arrays have a fixed size and can store various types of data. They also support multidimensional arrays and various operations such as insertion, deletion, and searching. In the next part of this series, we will delve deeper into the implementation and manipulation of arrays in data structures.
Arrays are a fundamental data structure used in computer programming. They provide a way to store and organize a collection of elements of the same type. In this article, we will explore the benefits and limitations of using arrays in data structures.
One of the main advantages of using arrays is their simplicity. Arrays are easy to understand and use, making them a popular choice for beginners. They provide a straightforward way to store and access data, as each element in the array is assigned a unique index. This allows for efficient retrieval of elements, as accessing an element by its index has a constant time complexity.
Another benefit of arrays is their efficiency in terms of memory usage. Arrays allocate a contiguous block of memory to store their elements, which allows for efficient memory management. This means that arrays have a fixed size, which is determined at the time of their creation. This can be advantageous in situations where the size of the data is known in advance, as it eliminates the need for dynamic memory allocation.
Arrays also offer efficient random access to elements. As mentioned earlier, accessing an element by its index has a constant time complexity. This makes arrays a suitable choice when frequent random access to elements is required. For example, arrays are commonly used in algorithms that involve searching or sorting elements.
However, arrays also have some limitations that need to be considered. One major limitation is their fixed size. Once an array is created, its size cannot be changed. This means that if the number of elements exceeds the size of the array, it becomes necessary to create a new array with a larger size and copy the elements from the old array to the new one. This process, known as resizing, can be time-consuming and inefficient, especially if it needs to be done frequently.
Another limitation of arrays is their lack of flexibility. As mentioned earlier, arrays can only store elements of the same type. This can be restrictive in situations where a data structure needs to store elements of different types. In such cases, other data structures, such as linked lists or hash tables, may be more suitable.
In addition, arrays have a fixed order, meaning that the elements are stored in a specific sequence. This can be a limitation in situations where the order of the elements needs to be changed frequently. For example, if elements need to be inserted or deleted in the middle of the array, it requires shifting all the subsequent elements, which can be time-consuming and inefficient.
In conclusion, arrays are a simple and efficient data structure that offer benefits such as simplicity, efficient memory usage, and random access to elements. However, they also have limitations, including their fixed size, lack of flexibility, and fixed order. It is important to consider these factors when deciding whether to use arrays in a particular data structure. In the next part of this series, we will explore other data structures that can be used as alternatives to arrays.
An array is a fundamental data structure that stores a fixed-size sequence of elements of the same type. It is widely used in computer programming due to its simplicity and efficiency. In this article, we will explore common operations and algorithms for arrays in data structures.
One of the most basic operations on an array is accessing its elements. Each element in an array is assigned a unique index, starting from zero. This allows for constant-time access to any element in the array. For example, if we have an array of integers called "numbers," we can access the element at index 2 by using the expression "numbers[2]." This operation is essential for manipulating and processing data stored in arrays.
Another common operation is inserting an element into an array. There are two scenarios to consider: inserting an element at the beginning of the array and inserting an element at a specific position within the array. When inserting an element at the beginning, all existing elements need to be shifted to the right to make room for the new element. This operation has a time complexity of O(n), where n is the number of elements in the array. On the other hand, inserting an element at a specific position requires shifting all elements from that position onwards to the right. This operation also has a time complexity of O(n).
Similarly, deleting an element from an array can be done in two ways: deleting an element from the beginning and deleting an element from a specific position. When deleting an element from the beginning, all remaining elements need to be shifted to the left to fill the gap. This operation also has a time complexity of O(n). Deleting an element from a specific position requires shifting all elements from that position onwards to the left. Again, this operation has a time complexity of O(n).
Searching for an element in an array is another common operation. The simplest approach is to iterate through each element in the array and compare it with the target element. If a match is found, the index of the element is returned. However, this approach has a time complexity of O(n) in the worst case, where n is the number of elements in the array. To improve the efficiency of searching, various algorithms like binary search can be used. Binary search is a divide-and-conquer algorithm that works on sorted arrays. It has a time complexity of O(log n), which is significantly faster than linear search.
Sorting an array is a crucial operation in many applications. There are numerous sorting algorithms available, each with its own advantages and disadvantages. Some popular sorting algorithms include bubble sort, insertion sort, selection sort, merge sort, and quicksort. The choice of sorting algorithm depends on factors such as the size of the array, the distribution of the elements, and the desired time complexity. Sorting an array can have a time complexity ranging from O(n log n) to O(n^2), depending on the algorithm used.
In conclusion, arrays are versatile data structures that support various operations and algorithms. They provide efficient access to elements, but inserting, deleting, searching, and sorting elements can be time-consuming depending on the size of the array and the chosen algorithm. Understanding these common operations and algorithms is essential for effectively working with arrays in data structures. In the next part of this series, we will delve deeper into advanced operations and algorithms for arrays.
1. What is an array in data structures?
An array is a data structure that stores a fixed-size sequence of elements of the same type in contiguous memory locations.
2. What are the advantages of using arrays?
Arrays provide constant-time access to elements, as they can be accessed using their index. They also allow efficient memory allocation and deallocation, and support random access to elements.
3. What are the limitations of arrays?
Arrays have a fixed size, which means they cannot be easily resized once created. Additionally, inserting or deleting elements in the middle of an array can be inefficient, as it requires shifting all subsequent elements.
In conclusion, arrays are an essential data structure in computer science. They provide a way to store and access multiple elements of the same data type in a contiguous memory block. Arrays offer efficient random access to elements and are widely used in various algorithms and applications. Understanding arrays and their properties is crucial for developing efficient and optimized programs.