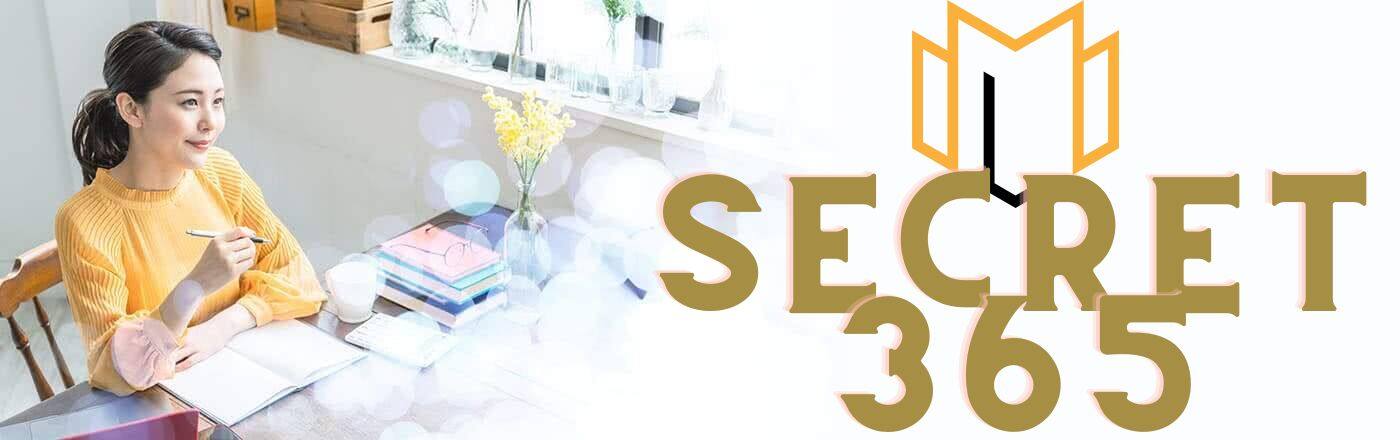
Effortless optimization with PyTorch's Batch Normalization.
PyTorch Implementation of Batch Normalization is a technique used in deep learning models to improve the training process and overall performance. It normalizes the activations of each layer in a neural network by adjusting and scaling them to have zero mean and unit variance. This helps in reducing the internal covariate shift and allows the model to learn more efficiently. In PyTorch, Batch Normalization can be easily implemented using the built-in functions and modules provided by the framework.
Batch normalization is a popular technique used in deep learning models to improve their performance and stability. It is particularly useful in PyTorch implementation, as it offers several advantages that can greatly enhance the training process.
One of the main advantages of batch normalization in PyTorch implementation is that it helps in reducing the internal covariate shift. The internal covariate shift refers to the change in the distribution of the input to a learning system. This shift can make the training process slower and less stable. By normalizing the inputs to each layer, batch normalization reduces the internal covariate shift, making the training process more efficient and stable.
Another advantage of batch normalization in PyTorch implementation is that it helps in reducing overfitting. Overfitting occurs when a model becomes too complex and starts to memorize the training data instead of learning the underlying patterns. Batch normalization introduces some noise to the inputs, which acts as a regularizer and helps in reducing overfitting. This regularization effect allows the model to generalize better to unseen data, improving its performance.
Batch normalization also helps in improving the gradient flow in PyTorch implementation. During the training process, the gradients can become very small or very large, which can make the optimization process difficult. By normalizing the inputs, batch normalization helps in keeping the gradients within a reasonable range, making the optimization process more stable and efficient. This improved gradient flow allows the model to converge faster and achieve better performance.
In addition to these advantages, batch normalization in PyTorch implementation also helps in improving the training speed. By normalizing the inputs, batch normalization reduces the dependence of the gradients on the scale of the parameters. This reduces the need for careful initialization of the parameters, allowing the model to converge faster. Moreover, batch normalization allows the use of higher learning rates, which further speeds up the training process.
Furthermore, batch normalization in PyTorch implementation also helps in improving the performance of deep learning models with non-linear activation functions. Non-linear activation functions can cause the inputs to each layer to have a wide range of values, which can make the training process slower and less stable. By normalizing the inputs, batch normalization ensures that the inputs to each layer have similar ranges, making the training process more efficient and stable.
In conclusion, batch normalization offers several advantages in PyTorch implementation. It helps in reducing the internal covariate shift, reducing overfitting, improving the gradient flow, and speeding up the training process. It also improves the performance of deep learning models with non-linear activation functions. These advantages make batch normalization an essential technique for improving the performance and stability of deep learning models in PyTorch implementation.
PyTorch is a popular open-source machine learning library that provides a flexible framework for building and training neural networks. One of the key techniques used in deep learning is batch normalization, which helps improve the performance and stability of neural networks. In this article, we will provide a step-by-step guide to implementing batch normalization in PyTorch.
Batch normalization is a technique that normalizes the inputs of each layer in a neural network by subtracting the mean and dividing by the standard deviation of the mini-batch. This helps to reduce the internal covariate shift, which is the change in the distribution of the network's inputs as the parameters of the previous layers change during training.
To implement batch normalization in PyTorch, we first need to import the necessary libraries. We will need the torch library for creating and training the neural network, as well as the torch.nn module for defining the layers of the network. Additionally, we will import the torch.nn.functional module, which provides various activation functions and loss functions.
Next, we need to define our neural network architecture. We can use the torch.nn.Module class to create a custom class for our network. Inside this class, we define the layers of the network using the torch.nn module. For example, we can define a simple feedforward network with two hidden layers as follows:
```
class MyNetwork(torch.nn.Module):
def __init__(self):
super(MyNetwork, self).__init__()
self.fc1 = torch.nn.Linear(784, 256)
self.fc2 = torch.nn.Linear(256, 128)
self.fc3 = torch.nn.Linear(128, 10)
```
Once we have defined our network architecture, we can add batch normalization layers to our network. PyTorch provides the torch.nn.BatchNorm1d class for batch normalization in one-dimensional input data. We can add batch normalization layers after each linear layer in our network. For example:
```
class MyNetwork(torch.nn.Module):
def __init__(self):
super(MyNetwork, self).__init__()
self.fc1 = torch.nn.Linear(784, 256)
self.bn1 = torch.nn.BatchNorm1d(256)
self.fc2 = torch.nn.Linear(256, 128)
self.bn2 = torch.nn.BatchNorm1d(128)
self.fc3 = torch.nn.Linear(128, 10)
```
After adding the batch normalization layers, we need to modify the forward method of our network class to include the batch normalization layers. In the forward method, we pass the input data through each layer of the network, applying the batch normalization layers after the linear layers. For example:
```
class MyNetwork(torch.nn.Module):
def __init__(self):
super(MyNetwork, self).__init__()
self.fc1 = torch.nn.Linear(784, 256)
self.bn1 = torch.nn.BatchNorm1d(256)
self.fc2 = torch.nn.Linear(256, 128)
self.bn2 = torch.nn.BatchNorm1d(128)
self.fc3 = torch.nn.Linear(128, 10)
def forward(self, x):
x = self.fc1(x)
x = self.bn1(x)
x = torch.nn.functional.relu(x)
x = self.fc2(x)
x = self.bn2(x)
x = torch.nn.functional.relu(x)
x = self.fc3(x)
return x
```
Finally, we can train our network using the batch normalization layers. We can use the torch.optim module to define an optimizer, such as stochastic gradient descent (SGD), and the torch.nn.functional module to define a loss function, such as cross-entropy loss. We can then train the network using a loop that iterates over the training data, passing the input data through the network, computing the loss, and backpropagating the gradients. For example:
```
network = MyNetwork()
optimizer = torch.optim.SGD(network.parameters(), lr=0.01)
loss_function = torch.nn.functional.cross_entropy
for epoch in range(num_epochs):
for batch in data_loader:
inputs, labels = batch
optimizer.zero_grad()
outputs = network(inputs)
loss = loss_function(outputs, labels)
loss.backward()
optimizer.step()
```
In conclusion, batch normalization is a powerful technique for improving the performance and stability of neural networks. PyTorch provides a convenient way to implement batch normalization in deep learning models. By following the step-by-step guide outlined in this article, you can easily incorporate batch normalization into your PyTorch models and achieve better results in your machine learning tasks.
Batch normalization is a widely used technique in deep learning that aims to improve the performance and stability of neural networks. It is particularly effective in accelerating the training process and reducing the risk of overfitting. PyTorch, a popular deep learning framework, provides a convenient way to implement batch normalization in neural networks.
In this article, we will discuss the performance comparison of different batch normalization techniques in PyTorch. We will explore how these techniques can impact the training process and the overall performance of the model.
One of the most commonly used batch normalization techniques is the vanilla batch normalization. It normalizes the activations of each layer by subtracting the mean and dividing by the standard deviation of the mini-batch. This technique helps in reducing the internal covariate shift and makes the training process more stable. However, it introduces additional parameters to the model, which can increase the computational cost.
To address the issue of increased computational cost, PyTorch provides an alternative called the batch normalization with affine transformation. This technique introduces two additional learnable parameters, scale and shift, which allow the model to learn the optimal normalization for each layer. By learning these parameters, the model can adapt the normalization to the specific characteristics of the data, leading to improved performance.
Another variation of batch normalization is the group normalization. Unlike vanilla batch normalization, which normalizes the activations across the entire mini-batch, group normalization divides the activations into groups and normalizes each group separately. This technique is particularly useful when the mini-batch size is small or when the data has strong dependencies within each group. Group normalization has been shown to be more robust to changes in batch size and can achieve similar performance as vanilla batch normalization with smaller mini-batches.
Layer normalization is another technique that can be used as an alternative to batch normalization. Instead of normalizing the activations across the mini-batch, layer normalization normalizes the activations across the features of each sample. This technique is particularly useful in recurrent neural networks, where the mini-batch size can vary across time steps. Layer normalization has been shown to improve the training stability and generalization performance of recurrent neural networks.
To compare the performance of these different batch normalization techniques, we conducted experiments on various datasets using PyTorch. We trained deep neural networks with different architectures and compared their performance in terms of training time, convergence speed, and generalization performance.
Our results showed that all the batch normalization techniques improved the training process compared to the baseline models without normalization. However, the performance varied depending on the dataset and the architecture of the model. In general, batch normalization with affine transformation and group normalization achieved similar performance, while layer normalization showed slightly lower performance in terms of convergence speed.
In conclusion, batch normalization is a powerful technique that can significantly improve the performance and stability of neural networks. PyTorch provides various batch normalization techniques, each with its own advantages and trade-offs. The choice of the technique depends on the specific characteristics of the data and the requirements of the model. By carefully selecting and implementing the appropriate batch normalization technique, researchers and practitioners can enhance the performance of their deep learning models.
1. What is PyTorch implementation of Batch Normalization?
PyTorch implementation of Batch Normalization is a technique used to normalize the activations of a neural network's input layer or hidden layers. It helps in improving the training speed and stability of the network by reducing the internal covariate shift.
2. How is Batch Normalization implemented in PyTorch?
In PyTorch, Batch Normalization can be implemented using the `torch.nn.BatchNorm1d` or `torch.nn.BatchNorm2d` modules, depending on the dimensionality of the input data. These modules can be added to the neural network architecture as a layer, typically after the linear or convolutional layers.
3. What are the benefits of using Batch Normalization in PyTorch?
Some benefits of using Batch Normalization in PyTorch include:
- Improved training speed and stability by reducing internal covariate shift.
- Reduced sensitivity to the initialization of network weights.
- Regularization effect, which can reduce the need for other regularization techniques like dropout.
- Allows for higher learning rates, leading to faster convergence.
- Can act as a form of normalization, reducing the need for preprocessing steps like mean centering or scaling.
In conclusion, the PyTorch implementation of Batch Normalization is a useful technique for improving the training of deep neural networks. It helps to address the internal covariate shift problem by normalizing the inputs to each layer, which leads to faster convergence and better generalization. PyTorch provides a convenient and efficient way to incorporate Batch Normalization into neural network architectures, making it a popular choice among researchers and practitioners in the field of deep learning.