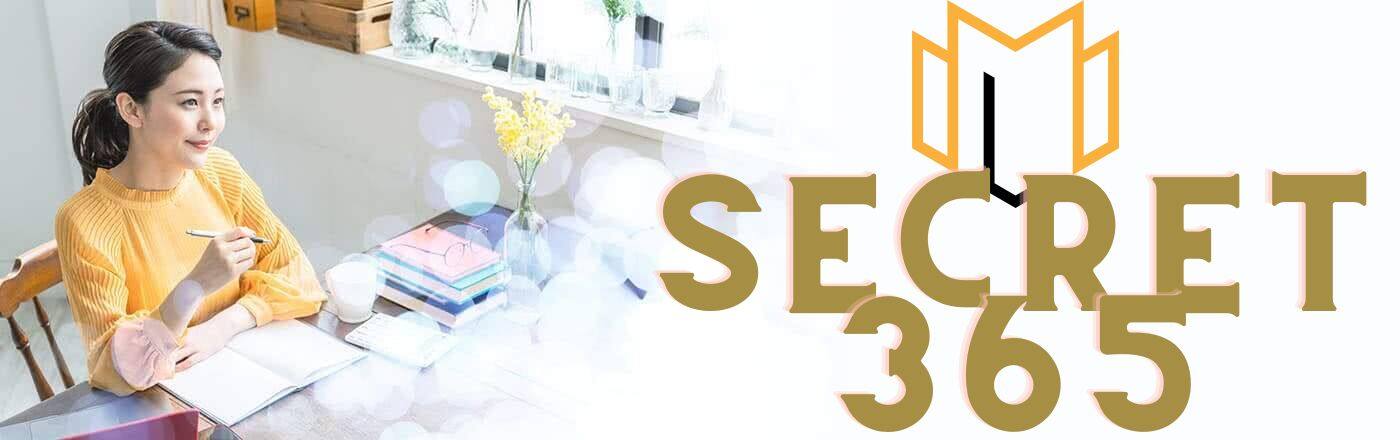
Master the essentials of Python for Data and AI.
Introduction:
Python is a versatile and powerful programming language widely used in the field of data analysis and artificial intelligence (AI). Understanding the essential concepts of Python is crucial for anyone looking to work with data and AI. This article will provide an overview of the fundamental Python concepts that are essential for mastering the basics of data analysis and AI. By grasping these concepts, you will be equipped with the necessary foundation to explore and manipulate data, build machine learning models, and develop AI applications using Python.
Python is a versatile programming language that has gained immense popularity in recent years, especially in the field of data analysis and artificial intelligence (AI). Its simplicity, readability, and extensive libraries make it an ideal choice for beginners and experts alike. In this article, we will explore some essential Python concepts that are crucial for mastering the basics of data and AI applications.
First and foremost, understanding variables is fundamental in any programming language, and Python is no exception. Variables are used to store data, and they can hold different types of values such as numbers, strings, or even more complex data structures like lists or dictionaries. Python is a dynamically typed language, which means that you don't need to explicitly declare the type of a variable. Instead, Python infers the type based on the value assigned to it.
Next, let's delve into the concept of control flow. Control flow refers to the order in which statements are executed in a program. Python provides various control flow structures, such as if-else statements and loops, to control the flow of execution based on certain conditions. If-else statements allow you to execute different blocks of code depending on whether a condition is true or false. Loops, on the other hand, enable you to repeat a block of code multiple times until a certain condition is met.
Another crucial concept in Python is functions. Functions are reusable blocks of code that perform a specific task. They allow you to break down complex problems into smaller, more manageable pieces. Python provides built-in functions, such as print() or len(), but you can also define your own functions. Defining functions not only makes your code more organized and modular but also enhances code reusability and maintainability.
Lists and dictionaries are two essential data structures in Python. A list is an ordered collection of items, while a dictionary is an unordered collection of key-value pairs. Lists are versatile and can hold different types of data, making them ideal for storing and manipulating large amounts of information. Dictionaries, on the other hand, provide a convenient way to store and retrieve data based on unique keys.
Python's extensive library ecosystem is one of its greatest strengths. Libraries like NumPy, Pandas, and Matplotlib provide powerful tools for data manipulation, analysis, and visualization. NumPy, for instance, offers efficient numerical operations and multi-dimensional arrays, while Pandas provides data structures and functions for data manipulation and analysis. Matplotlib, on the other hand, enables you to create high-quality visualizations to gain insights from your data.
Lastly, error handling is an essential skill for any programmer. Python provides a robust mechanism for handling errors through exception handling. Exceptions are raised when an error occurs during the execution of a program, and they can be caught and handled using try-except blocks. Exception handling allows you to gracefully handle errors and prevent your program from crashing.
In conclusion, mastering the basics of Python programming is crucial for anyone interested in data analysis and AI applications. Understanding variables, control flow, functions, data structures, libraries, and error handling are essential concepts that form the foundation of Python programming. By familiarizing yourself with these concepts and practicing them in real-world scenarios, you will be well-equipped to tackle more complex data and AI challenges. So, dive into the world of Python and start exploring its vast possibilities!
Python is a versatile programming language that has gained immense popularity in the field of data analysis and artificial intelligence (AI). Whether you are a beginner or an experienced programmer, understanding the essential concepts of Python is crucial for mastering data and AI. In this article, we will explore some of the fundamental data structures and algorithms in Python that are essential for anyone working in these domains.
One of the most basic data structures in Python is the list. A list is an ordered collection of elements, which can be of any type. Lists are mutable, meaning that you can modify their contents. They are incredibly useful for storing and manipulating data. To create a list, you simply enclose the elements within square brackets, separated by commas. For example, [1, 2, 3, 4, 5] is a list of integers.
Another important data structure in Python is the dictionary. A dictionary is an unordered collection of key-value pairs. Unlike lists, dictionaries are not indexed by integers but by keys. Keys can be of any immutable type, such as strings or numbers. To create a dictionary, you enclose the key-value pairs within curly braces, separated by commas. For example, {'name': 'John', 'age': 25} is a dictionary with two key-value pairs.
Python also provides sets, which are unordered collections of unique elements. Sets are useful when you want to eliminate duplicate values from a collection or perform mathematical set operations such as union, intersection, and difference. To create a set, you enclose the elements within curly braces, separated by commas. For example, {1, 2, 3, 4, 5} is a set of integers.
Now that we have covered some essential data structures, let's move on to algorithms. An algorithm is a step-by-step procedure for solving a problem or performing a task. In Python, you can implement algorithms using functions. Functions are reusable blocks of code that perform a specific task. They take input arguments, perform some operations, and return a result. To define a function in Python, you use the def keyword followed by the function name and a set of parentheses containing the input arguments. For example, def add_numbers(a, b): return a + b defines a function called add_numbers that takes two input arguments and returns their sum.
One of the most commonly used algorithms in data and AI is sorting. Sorting is the process of arranging elements in a specific order, such as ascending or descending. Python provides a built-in function called sorted() that can be used to sort lists, sets, and other iterable objects. For example, sorted([3, 1, 4, 2, 5]) returns [1, 2, 3, 4, 5].
Another important algorithm is searching. Searching is the process of finding a specific element in a collection. Python provides several searching algorithms, such as linear search and binary search. Linear search is a simple algorithm that checks each element in a collection until it finds the desired element. Binary search, on the other hand, is a more efficient algorithm that works on sorted collections by repeatedly dividing the search space in half. These algorithms are essential for efficiently retrieving data from large datasets.
In conclusion, understanding essential data structures and algorithms in Python is crucial for mastering data and AI. Lists, dictionaries, and sets are fundamental data structures that allow you to store and manipulate data efficiently. Functions and algorithms, such as sorting and searching, enable you to perform complex operations on your data. By mastering these basic concepts, you will be well-equipped to tackle more advanced topics in data analysis and AI using Python.
Python has become one of the most popular programming languages for data manipulation and analysis, particularly in the field of artificial intelligence (AI). Its simplicity, versatility, and extensive libraries make it an ideal choice for data scientists and AI practitioners. In this article, we will explore some essential Python concepts that are crucial for mastering the basics of data manipulation and analysis.
One of the key libraries in Python for data manipulation is Pandas. Pandas provides data structures and functions that allow for efficient data manipulation and analysis. It introduces two primary data structures: Series and DataFrame. A Series is a one-dimensional array-like object that can hold any data type, while a DataFrame is a two-dimensional table-like structure that can hold multiple data types. These data structures enable easy indexing, slicing, and filtering of data, making data manipulation a breeze.
Another important library for data manipulation in Python is NumPy. NumPy provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. It is the foundation for many other libraries in Python, including Pandas. NumPy's array object is more efficient and convenient than Python's built-in list, especially when dealing with large datasets. It also offers various functions for array manipulation, such as reshaping, sorting, and concatenating arrays.
When it comes to data visualization, Matplotlib is a powerful library in Python. It provides a wide range of plotting options, allowing users to create various types of charts, graphs, and plots. Matplotlib's pyplot module provides a simple interface for creating and customizing plots. With just a few lines of code, you can generate visually appealing visualizations to better understand your data.
For more advanced data analysis and modeling, the Scikit-learn library is a must-have in your Python toolkit. Scikit-learn offers a comprehensive set of tools for machine learning and statistical modeling. It includes various algorithms for classification, regression, clustering, and dimensionality reduction. Scikit-learn also provides utilities for data preprocessing, model evaluation, and model selection. With Scikit-learn, you can easily build and deploy machine learning models for your data analysis and AI projects.
In addition to these libraries, Python also offers a rich ecosystem of specialized libraries and frameworks for specific data analysis tasks. For example, TensorFlow and PyTorch are popular libraries for deep learning and neural network modeling. These libraries provide high-level APIs for building and training complex neural networks. They also offer tools for distributed computing and GPU acceleration, making them suitable for large-scale AI projects.
To effectively utilize these libraries and frameworks, it is essential to have a solid understanding of Python's core concepts. This includes knowledge of data types, control flow structures, functions, and object-oriented programming. Python's simplicity and readability make it easy to learn and use, even for beginners. However, mastering these fundamental concepts will greatly enhance your ability to manipulate and analyze data in Python.
In conclusion, Python is a versatile and powerful programming language for data manipulation and analysis, particularly in the field of AI. By mastering essential Python concepts and familiarizing yourself with key libraries and frameworks, you can unlock the full potential of Python for your data analysis and AI projects. Whether you are a beginner or an experienced data scientist, Python provides the tools and resources you need to succeed in the world of data and AI.
1. What are some essential Python concepts for data and AI?
Some essential Python concepts for data and AI include variables, data types, control flow statements (such as if-else and loops), functions, libraries (such as NumPy and Pandas), and object-oriented programming.
2. Why are these concepts important for data and AI?
These concepts are important for data and AI because they provide the foundation for manipulating and analyzing data, implementing algorithms, and building machine learning models. Understanding these concepts allows developers to effectively work with data and leverage Python's capabilities for data science and AI tasks.
3. How can one master the basics of Python for data and AI?
To master the basics of Python for data and AI, one can start by learning the fundamentals of Python programming, including variables, data types, and control flow statements. Then, they can explore libraries specifically designed for data science and AI, such as NumPy, Pandas, and scikit-learn. Practicing coding exercises, working on small projects, and studying relevant resources and tutorials can also help in mastering these concepts.
In conclusion, mastering the basics of essential Python concepts is crucial for individuals working with data and AI. Understanding concepts such as variables, data types, control flow, functions, and libraries is essential for effectively manipulating and analyzing data. Additionally, knowledge of Python's object-oriented programming principles and its extensive library ecosystem is vital for developing AI applications. By mastering these fundamental concepts, individuals can lay a strong foundation for further exploration and advancement in the field of data and AI.