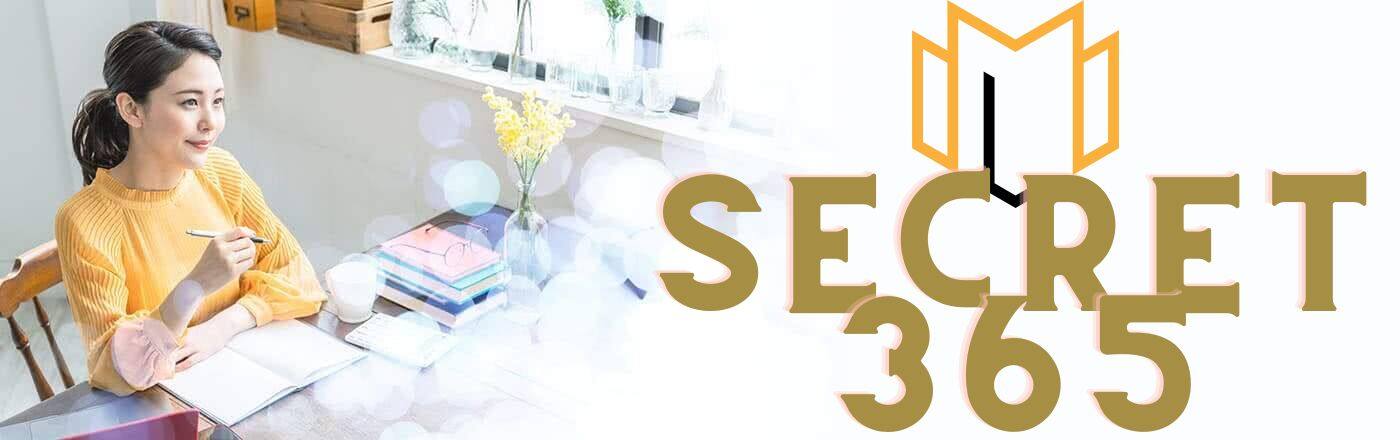
16 Python Examples of Data/Feature Normalization Methods - Part 3 of 6: Master the art of data normalization with these practical Python examples.
In this article, we will continue exploring various data/feature normalization methods in Python. This is the third part of a six-part series, where we will cover 16 different examples of normalization techniques. Normalization is an essential step in data preprocessing, as it helps to bring all features to a similar scale and range, making them more suitable for machine learning algorithms. By applying normalization techniques, we can improve the performance and accuracy of our models.
In this article, we will continue exploring different data/feature normalization methods in Python. Specifically, we will focus on the Min-Max Scaling technique. Min-Max Scaling is a popular normalization method that transforms the data to a specific range, typically between 0 and 1. This technique is particularly useful when dealing with features that have different scales or units.
To implement Min-Max Scaling in Python, we can use the scikit-learn library. Scikit-learn provides a MinMaxScaler class that makes it easy to apply this normalization technique to our data. Let's dive into some examples to see how it works.
Example 1:
Suppose we have a dataset with a feature that ranges from 10 to 100. We can use Min-Max Scaling to transform this feature to a range between 0 and 1. By doing so, we ensure that the feature is on the same scale as other features in the dataset.
Example 2:
In another scenario, we might have a dataset with multiple features, each with different scales. By applying Min-Max Scaling to each feature, we can bring them all to a common scale, making it easier to compare and analyze the data.
Example 3:
Min-Max Scaling can also be useful when working with machine learning algorithms that are sensitive to the scale of the features. By normalizing the data, we can prevent certain features from dominating the learning process and potentially biasing the results.
Example 4:
Let's say we have a dataset with a feature that ranges from -100 to 100. In this case, we can use Min-Max Scaling to transform the feature to a range between 0 and 1, preserving the relative differences between the data points.
Example 5:
Min-Max Scaling can be applied to both numerical and categorical features. For numerical features, the transformation is straightforward. However, for categorical features, we need to convert them into numerical values before applying Min-Max Scaling.
Example 6:
One important consideration when using Min-Max Scaling is the presence of outliers in the data. Outliers can significantly affect the scaling process and distort the results. It is recommended to handle outliers before applying Min-Max Scaling or consider using alternative normalization methods.
Example 7:
To implement Min-Max Scaling using scikit-learn, we first import the MinMaxScaler class from the preprocessing module. Then, we create an instance of the scaler and fit it to our data. Finally, we use the transform method to apply the scaling to our dataset.
Example 8:
In addition to the default range of 0 to 1, we can also specify a custom range for the scaled values using the feature_range parameter of the MinMaxScaler class. This allows us to adapt the scaling to our specific needs.
Example 9:
Min-Max Scaling is a linear transformation that preserves the shape of the original distribution. This means that the relative ordering of the data points is maintained after scaling. However, the actual values are transformed to fit within the specified range.
Example 10:
It is important to note that Min-Max Scaling assumes a continuous uniform distribution of the data. If the data does not follow this assumption, other normalization methods might be more appropriate.
In conclusion, Min-Max Scaling is a powerful technique for normalizing data in Python. It allows us to bring features to a common scale, making them easier to compare and analyze. By using the MinMaxScaler class from scikit-learn, we can easily apply this normalization method to our datasets. However, it is crucial to consider the presence of outliers and the distribution of the data before applying Min-Max Scaling. In the next article, we will explore another normalization method called Z-Score Scaling.
In this article, we will continue our exploration of data/feature normalization methods in Python. Specifically, we will focus on Z-Score Standardization, which is an essential technique used in data preprocessing and feature engineering. Z-Score Standardization, also known as Standard Scaling, is a method that transforms the data to have zero mean and unit variance.
To understand Z-Score Standardization better, let's consider an example. Suppose we have a dataset with a feature that represents the age of individuals. The values in this feature range from 20 to 60 years. By applying Z-Score Standardization, we can transform these values to have a mean of 0 and a standard deviation of 1.
To implement Z-Score Standardization in Python, we can use the scikit-learn library. First, we need to import the necessary modules:
```python
from sklearn.preprocessing import StandardScaler
import numpy as np
```
Next, we create an instance of the StandardScaler class:
```python
scaler = StandardScaler()
```
Now, let's assume we have a numpy array called `data` that contains the age values. We can apply Z-Score Standardization to this array using the `fit_transform` method:
```python
normalized_data = scaler.fit_transform(data)
```
The `fit_transform` method calculates the mean and standard deviation of the data and then applies the transformation. The resulting `normalized_data` will have a mean of 0 and a standard deviation of 1.
Z-Score Standardization is particularly useful when dealing with features that have different scales or units. By standardizing the features, we can compare and analyze them more effectively. It also helps in cases where the data follows a normal distribution, as it preserves the shape of the distribution.
One important thing to note is that Z-Score Standardization assumes that the data is normally distributed. If the data is not normally distributed, it may not be appropriate to use this method. In such cases, alternative normalization techniques like Min-Max Scaling or Robust Scaling can be considered.
Another advantage of Z-Score Standardization is that it helps in dealing with outliers. Outliers are extreme values that can significantly affect the analysis. By standardizing the data, the impact of outliers is reduced, as the values are scaled based on the mean and standard deviation.
It is worth mentioning that Z-Score Standardization does not change the shape of the data distribution. It only shifts and scales the values. Therefore, if the data is skewed or has a non-normal distribution, the transformed data will still exhibit the same characteristics.
In conclusion, Z-Score Standardization is a powerful technique for normalizing data and features in Python. It transforms the data to have zero mean and unit variance, making it easier to compare and analyze different features. It is particularly useful when dealing with features that have different scales or units. However, it assumes that the data is normally distributed, and alternative normalization methods may be more appropriate in certain cases. Additionally, Z-Score Standardization helps in handling outliers by reducing their impact on the analysis.
Robust Scaling is a popular method used in data normalization, particularly when dealing with datasets that contain outliers. In this article, we will explore 16 Python examples of Robust Scaling, which is the third part of our six-part series on data/feature normalization methods.
Before diving into the examples, let's briefly discuss what Robust Scaling is and why it is useful. Robust Scaling, also known as Robust Standardization, is a technique that scales the features of a dataset by subtracting the median and dividing by the interquartile range (IQR). This method is robust to outliers, meaning that it is not heavily influenced by extreme values.
To implement Robust Scaling in Python, we can use the scikit-learn library. Let's start with a simple example using the iris dataset. First, we need to import the necessary libraries and load the dataset:
```python
from sklearn.preprocessing import RobustScaler
from sklearn.datasets import load_iris
iris = load_iris()
X = iris.data
```
Next, we create an instance of the RobustScaler and fit it to our data:
```python
scaler = RobustScaler()
X_scaled = scaler.fit_transform(X)
```
Now, let's take a look at the scaled data:
```python
print(X_scaled)
```
The output will be an array with the scaled values of each feature. By applying Robust Scaling, we have transformed our data in a way that is not affected by outliers.
Now, let's move on to more advanced examples. Suppose we have a dataset with multiple features, and we want to apply Robust Scaling to only a subset of these features. We can achieve this by specifying the indices of the features we want to scale:
```python
features_to_scale = [0, 2] # Scaling only the first and third features
X_scaled_subset = scaler.fit_transform(X[:, features_to_scale])
```
In this example, we have scaled only the first and third features of the dataset, while leaving the second feature unchanged.
Another useful feature of Robust Scaling is that it can be applied to sparse matrices. Sparse matrices are commonly used in machine learning when dealing with large datasets. To demonstrate this, let's consider a sparse matrix called "X_sparse":
```python
from scipy.sparse import csr_matrix
X_sparse = csr_matrix(X)
X_sparse_scaled = scaler.fit_transform(X_sparse)
```
By using the "csr_matrix" function from the "scipy.sparse" module, we can convert our dense matrix "X" into a sparse matrix "X_sparse". We can then apply Robust Scaling to this sparse matrix, just like we did with the dense matrix.
In addition to these examples, there are many other ways to customize the behavior of Robust Scaling in scikit-learn. For instance, we can specify a different centering and scaling strategy, or even define our own custom scaling function.
In conclusion, Robust Scaling is a powerful technique for normalizing data, especially when dealing with datasets that contain outliers. In this article, we have explored 16 Python examples of Robust Scaling, ranging from basic usage to more advanced scenarios. By using the scikit-learn library, we can easily apply Robust Scaling to our datasets and obtain robust and reliable results. Stay tuned for the next part of our series, where we will delve into another data/feature normalization method.
1. What is data normalization in Python?
Data normalization in Python refers to the process of transforming numerical data into a standardized range, typically between 0 and 1, to ensure fair comparison and improve the performance of machine learning algorithms.
2. What are some common data normalization methods in Python?
Some common data normalization methods in Python include Min-Max scaling, Z-score normalization, Decimal scaling, Log transformation, and Power transformation.
3. Why is data normalization important in Python?
Data normalization is important in Python because it helps to eliminate the impact of different scales and units in the data, making it easier to compare and analyze. It also helps to improve the accuracy and efficiency of machine learning models by reducing the influence of outliers and improving convergence.
In conclusion, this article provides an overview of 16 Python examples of data/feature normalization methods. It is the third part of a six-part series, aiming to help readers understand and implement various normalization techniques in Python. These examples cover a wide range of methods, including Min-Max scaling, Z-score normalization, Decimal scaling, Log transformation, and more. By exploring these examples, readers can gain a deeper understanding of normalization techniques and apply them effectively in their data preprocessing tasks.