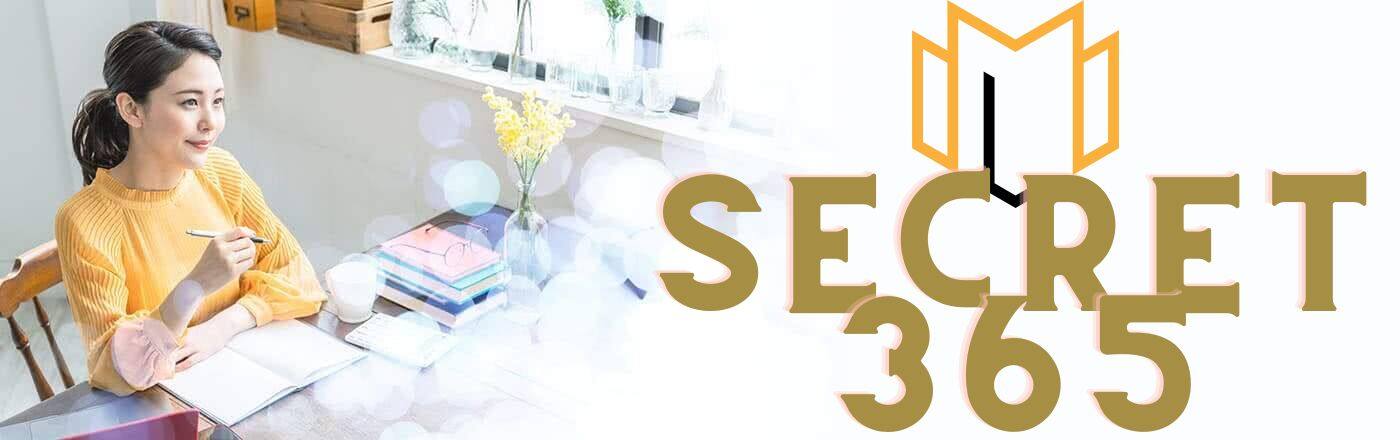
Master the power of ECMAScript with our comprehensive guide.
Introduction:
A Comprehensive Guide to ECMAScript (ES): Part 1 is a detailed resource that aims to provide a comprehensive understanding of ECMAScript, commonly known as ES. This guide is designed for developers who want to enhance their knowledge and skills in JavaScript, as ECMAScript is the standardized specification that JavaScript is based on. Part 1 of this guide covers the basics of ECMAScript, its history, key features, and its relationship with JavaScript. By the end of this guide, readers will have a solid foundation in ECMAScript and be well-equipped to utilize its powerful features in their JavaScript projects.
ECMAScript (ES) is a standardized scripting language that forms the basis for many popular programming languages, including JavaScript. In this comprehensive guide, we will explore the various aspects of ECMAScript, from its history to its features and applications. This article serves as an introduction to ECMAScript, providing a brief history and overview of this powerful scripting language.
To understand ECMAScript, it is essential to delve into its origins. The language was first introduced in 1997 by Ecma International, a standards organization responsible for developing and maintaining various technology standards. The goal was to create a standardized scripting language that could be implemented across different platforms and browsers. This standardization was crucial to ensure compatibility and interoperability among different web applications.
Initially, ECMAScript was designed to be a lightweight scripting language for web browsers. It provided a way to add interactivity and dynamic behavior to web pages. JavaScript, which is the most widely used implementation of ECMAScript, quickly gained popularity due to its versatility and ease of use. As web development evolved, ECMAScript continued to evolve as well, with new versions being released periodically.
One of the defining features of ECMAScript is its backward compatibility. This means that newer versions of the language are designed to be compatible with older versions, ensuring that existing codebases can be easily migrated to newer versions without breaking functionality. This backward compatibility has been a key factor in the widespread adoption of ECMAScript.
Over the years, ECMAScript has undergone several major revisions, each introducing new features and improvements. ECMAScript 6, also known as ES6 or ES2015, was a significant milestone in the evolution of the language. It introduced many new features, such as arrow functions, classes, modules, and enhanced syntax for working with arrays and objects. These additions made ECMAScript more powerful and expressive, enabling developers to write cleaner and more maintainable code.
Since ES6, new versions of ECMAScript have been released annually, with each version bringing new features and enhancements. ECMAScript 7 (ES7) introduced features like async/await, which simplified asynchronous programming. ES8 introduced features like async iterators and shared memory, while ES9 brought improvements to regular expressions and introduced new methods for working with arrays.
The latest version of ECMAScript, as of the time of writing, is ECMAScript 2021 (ES12). This version introduced features like logical assignment operators, string methods, and the Promise.any method. It also included improvements to the regular expression engine and introduced new syntax for private class fields.
In conclusion, ECMAScript is a standardized scripting language that has played a crucial role in the development of web applications. Its history dates back to 1997 when it was introduced as a lightweight scripting language for web browsers. Over the years, ECMAScript has evolved, with new versions being released regularly, each introducing new features and improvements. This comprehensive guide will explore the various aspects of ECMAScript, providing a deeper understanding of this powerful scripting language. In the next part of this series, we will delve into the core features of ECMAScript and explore how they can be used in practical applications.
ECMAScript (ES) is a standardized scripting language that forms the basis for many popular programming languages, including JavaScript. In this comprehensive guide, we will explore the key features and syntax of ECMAScript, providing you with a solid understanding of this powerful language.
One of the fundamental features of ECMAScript is its support for object-oriented programming. Objects are the building blocks of ECMAScript, and they allow developers to create complex data structures and manipulate them easily. Objects in ECMAScript are collections of key-value pairs, where the keys are strings and the values can be any data type, including other objects.
In addition to objects, ECMAScript also supports functions, which are blocks of code that can be invoked to perform a specific task. Functions in ECMAScript can be defined using the function keyword, and they can accept parameters and return values. This allows developers to create reusable pieces of code that can be called from different parts of their program.
Another important feature of ECMAScript is its support for variables. Variables are used to store data that can be accessed and manipulated throughout the program. In ECMAScript, variables can be declared using the var, let, or const keywords. The var keyword is used to declare variables with function scope, while the let and const keywords are used to declare variables with block scope.
ECMAScript also provides a wide range of operators that can be used to perform mathematical and logical operations. These operators include arithmetic operators (+, -, *, /), comparison operators (==, !=, >, <), and logical operators (&&, ||, !). Understanding how to use these operators is essential for writing effective ECMAScript code.
In terms of syntax, ECMAScript follows a set of rules that dictate how code should be written. Statements in ECMAScript are terminated with a semicolon (;), and blocks of code are enclosed in curly braces ({ }). Indentation is not required in ECMAScript, but it is recommended for readability purposes.
ECMAScript also supports a wide range of control flow statements, which allow developers to control the execution of their code. These control flow statements include if-else statements, switch statements, for loops, while loops, and do-while loops. By using these control flow statements, developers can create more complex and dynamic programs.
Error handling is another important aspect of ECMAScript. When an error occurs during the execution of a program, ECMAScript provides a mechanism for catching and handling these errors. This is done using try-catch blocks, where the code that might throw an error is placed inside the try block, and the code that handles the error is placed inside the catch block.
In conclusion, understanding the key features and syntax of ECMAScript is essential for any developer working with JavaScript or other languages that are based on ECMAScript. By mastering the concepts discussed in this article, you will be well-equipped to write clean, efficient, and error-free ECMAScript code. Stay tuned for Part 2 of this comprehensive guide, where we will delve deeper into advanced topics and techniques in ECMAScript.
ECMAScript (ES) is a standardized scripting language that forms the basis for many popular programming languages, including JavaScript. It provides a set of rules and guidelines for developers to follow, ensuring consistency and compatibility across different platforms and browsers. In this comprehensive guide, we will explore advanced concepts and best practices in ECMAScript (ES) development.
One of the key concepts in ECMAScript (ES) is the use of modules. Modules allow developers to organize their code into separate files, making it easier to manage and maintain. By breaking down a large codebase into smaller, reusable modules, developers can improve code readability and reusability. This modular approach also promotes better collaboration among team members, as each module can be developed and tested independently.
Another important concept in ECMAScript (ES) is the use of arrow functions. Arrow functions provide a concise syntax for writing functions, making the code more readable and easier to understand. They also have lexical scoping, which means they inherit the context of their surrounding code. This makes arrow functions particularly useful in callback functions and event handlers, where maintaining the correct context is crucial.
In addition to modules and arrow functions, ECMAScript (ES) also introduces several new features and syntax enhancements. One such feature is the spread operator, which allows developers to expand an iterable object into multiple elements. This can be useful when working with arrays or objects, as it simplifies the process of combining or cloning them.
Another notable feature is the async/await syntax, which provides a more intuitive way to work with asynchronous code. Traditionally, developers have used callbacks or promises to handle asynchronous operations. However, with async/await, developers can write asynchronous code that looks and behaves like synchronous code, making it easier to understand and debug.
When it comes to best practices in ECMAScript (ES) development, there are several guidelines that developers should follow. First and foremost, it is important to write clean and readable code. This includes using meaningful variable and function names, properly indenting code blocks, and adding comments to explain complex logic.
Another best practice is to use strict mode, which enforces stricter rules and prevents common mistakes. Strict mode helps catch errors and potential bugs early on, improving code quality and reliability. To enable strict mode, simply add the "use strict" directive at the beginning of your JavaScript file.
Furthermore, it is important to stay up to date with the latest ECMAScript (ES) specifications and features. The ECMAScript standard is constantly evolving, with new features and syntax enhancements being introduced regularly. By keeping up with these updates, developers can take advantage of the latest language features and improve their code.
In conclusion, ECMAScript (ES) is a powerful scripting language that provides developers with a set of rules and guidelines for writing consistent and compatible code. By understanding advanced concepts such as modules and arrow functions, and following best practices, developers can improve code organization, readability, and maintainability. Additionally, staying up to date with the latest ECMAScript (ES) specifications ensures that developers can take advantage of the latest language features and enhance their code. In the next part of this comprehensive guide, we will delve deeper into ECMAScript (ES) development, exploring more advanced concepts and best practices.
1. What is ECMAScript?
ECMAScript is a standardized scripting language specification that forms the basis for JavaScript. It defines the syntax, semantics, and core features of the language.
2. What is the purpose of A Comprehensive Guide to ECMAScript (ES): Part 1?
A Comprehensive Guide to ECMAScript (ES): Part 1 aims to provide a detailed overview of the ECMAScript language, covering its history, features, and usage. It serves as a comprehensive resource for developers looking to understand and utilize ECMAScript effectively.
3. What topics are covered in A Comprehensive Guide to ECMAScript (ES): Part 1?
A Comprehensive Guide to ECMAScript (ES): Part 1 covers various topics including the history of ECMAScript, its relationship with JavaScript, data types, variables, operators, control flow, functions, and objects. It also delves into advanced concepts like closures, prototypes, and modules.
In conclusion, A Comprehensive Guide to ECMAScript (ES): Part 1 provides a detailed overview of ECMAScript, the standardized scripting language used in web development. The guide covers the history, features, and syntax of ECMAScript, as well as its compatibility with different web browsers. It serves as a valuable resource for developers looking to understand and utilize ECMAScript effectively in their projects.