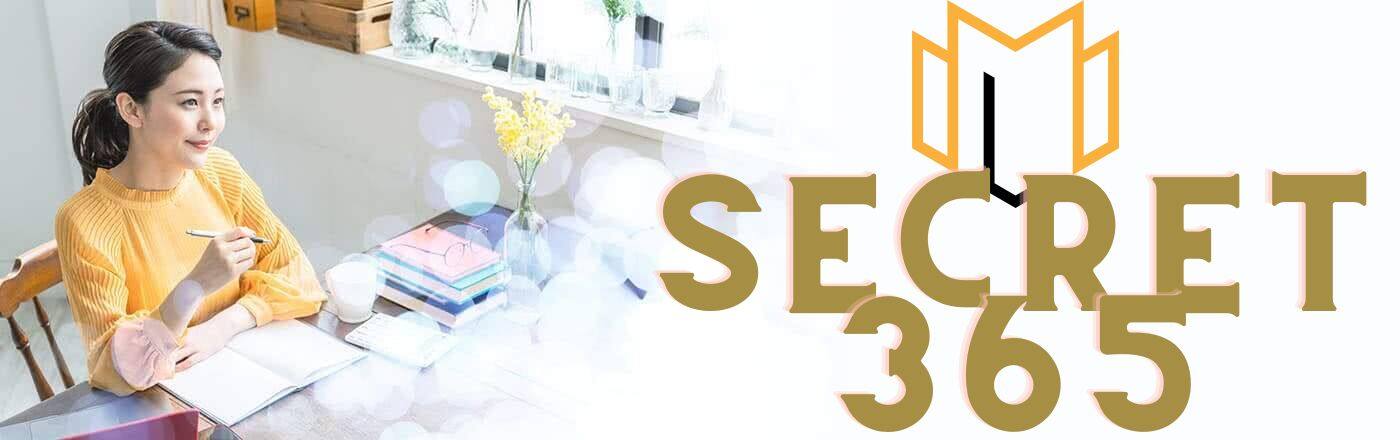
Master the art of advanced data visualization in Python with Plotly and Dash.
This article provides an introduction to advanced data visualization in Python using Plotly and Dash.
Introduction to Advanced Data Visualization in Python with Plotly and Dash
Data visualization is a crucial aspect of data analysis and interpretation. It allows us to present complex information in a visually appealing and easily understandable manner. Python, being a versatile programming language, offers several libraries for data visualization. In this article, we will explore the advanced data visualization capabilities of Python using Plotly and Dash.
Plotly is a powerful open-source library that provides interactive and high-quality visualizations. It supports a wide range of chart types, including scatter plots, line plots, bar charts, and more. With Plotly, you can create visually stunning and interactive plots that can be easily customized to suit your needs.
Dash, on the other hand, is a Python framework for building analytical web applications. It allows you to create interactive dashboards and web-based data visualizations. Dash combines the power of Plotly with the flexibility of web development, making it an excellent choice for creating interactive data visualizations that can be shared and accessed online.
To get started with Plotly and Dash, you will need to install the necessary libraries. You can do this by using the pip package manager, which is the standard tool for installing Python packages. Once you have installed Plotly and Dash, you can import them into your Python script and start creating visualizations.
Plotly provides a simple and intuitive API for creating plots. You can create a basic scatter plot, for example, by specifying the x and y coordinates of the data points. You can then customize the plot by adding labels, titles, and legends. Plotly also allows you to add interactivity to your plots, such as zooming, panning, and hovering over data points to display additional information.
Dash, on the other hand, allows you to create interactive web-based visualizations by defining a layout and adding components to it. You can create a dashboard with multiple plots, tables, and other interactive elements. Dash provides a wide range of components, such as sliders, dropdowns, and buttons, that you can use to create interactive controls for your visualizations.
One of the key advantages of using Plotly and Dash is their ability to handle large datasets. Plotly can handle millions of data points without sacrificing performance, thanks to its WebGL-based rendering engine. Dash, on the other hand, can handle large datasets by using efficient data caching and pagination techniques.
Another advantage of using Plotly and Dash is their support for collaboration and sharing. You can easily share your visualizations with others by exporting them as HTML files or embedding them in web pages. Plotly also provides a cloud-based platform called Plotly Cloud, where you can store and share your visualizations online.
In conclusion, Python offers powerful libraries for advanced data visualization, such as Plotly and Dash. These libraries allow you to create interactive and visually appealing plots and dashboards. Whether you are analyzing data for business purposes or conducting research, Plotly and Dash can help you present your findings in a compelling and informative manner. So why not give them a try and take your data visualization skills to the next level?
Python is a versatile programming language that is widely used for data analysis and visualization. While Python offers several libraries for data visualization, one library that stands out is Plotly. Plotly is a powerful and interactive data visualization library that allows users to create stunning visualizations with ease. In this article, we will explore the features and capabilities of Plotly and how it can be used to create advanced data visualizations in Python.
Plotly is an open-source library that provides a wide range of chart types, including scatter plots, line plots, bar charts, and more. It offers a simple and intuitive syntax, making it easy for users to create visually appealing charts. With Plotly, users can customize every aspect of their visualizations, from colors and fonts to axis labels and legends.
One of the key features of Plotly is its interactivity. Plotly charts are interactive by default, allowing users to zoom in and out, pan, and hover over data points to view additional information. This interactivity makes it easier for users to explore and analyze their data, as they can quickly drill down into specific areas of interest.
In addition to its interactivity, Plotly also offers a wide range of customization options. Users can add annotations, shapes, and images to their charts, making it easier to highlight important data points or add additional context to the visualization. Plotly also supports animations, allowing users to create dynamic visualizations that can help tell a story or convey complex information.
Another powerful feature of Plotly is its ability to create dashboards and web applications using Dash, a Python framework for building analytical web applications. With Dash, users can create interactive dashboards that allow them to explore and analyze their data in real-time. Dash provides a simple and declarative syntax for building web applications, making it easy for users to create complex and interactive dashboards without having to write a lot of code.
Plotly and Dash are both highly customizable, allowing users to create visually stunning and interactive dashboards that can be easily shared with others. Dash also provides support for deploying applications to the web, making it easy for users to share their dashboards with a wider audience.
In conclusion, Plotly is a powerful data visualization library for Python that offers a wide range of chart types, customization options, and interactivity. With Plotly, users can create visually appealing and interactive visualizations that allow them to explore and analyze their data more effectively. Additionally, with the integration of Dash, users can create interactive dashboards and web applications that can be easily shared with others. Whether you are a data scientist, analyst, or developer, Plotly is a valuable tool to have in your data visualization toolkit.
Building Interactive Dashboards with Dash: A Python Framework for Web Applications
In the world of data visualization, Python has emerged as a powerful tool for creating stunning and interactive visualizations. With its vast array of libraries and frameworks, Python offers developers a wide range of options to choose from. One such framework that has gained popularity in recent years is Dash.
Dash is a Python framework for building web applications. It allows developers to create interactive dashboards and data visualizations using Python, HTML, and CSS. With Dash, you can easily build and deploy web applications that are not only visually appealing but also highly functional.
One of the key features of Dash is its ability to create interactive visualizations using the Plotly library. Plotly is a graphing library that allows you to create a wide variety of charts and graphs, including scatter plots, bar charts, and line graphs. With Plotly, you can easily customize the appearance of your visualizations and add interactive features such as zooming and panning.
To get started with Dash and Plotly, you first need to install the necessary libraries. You can do this by running the following command in your terminal:
```
pip install dash plotly
```
Once you have installed the libraries, you can start building your interactive dashboard. The first step is to import the necessary modules:
```python
import dash
import dash_core_components as dcc
import dash_html_components as html
import plotly.graph_objs as go
```
Next, you need to create an instance of the Dash class:
```python
app = dash.Dash(__name__)
```
Now, you can start building the layout of your dashboard. Dash uses a declarative syntax, which means that you define the layout of your application using Python code. For example, to create a scatter plot, you can use the following code:
```python
app.layout = html.Div(children=[
html.H1(children='My Dashboard'),
dcc.Graph(
id='scatter-plot',
figure={
'data': [
go.Scatter(
x=[1, 2, 3],
y=[4, 1, 2],
mode='markers',
marker={
'size': 15,
'color': 'rgb(255, 0, 0)',
'symbol': 'circle'
}
)
],
'layout': go.Layout(
title='Scatter Plot',
xaxis={'title': 'X-axis'},
yaxis={'title': 'Y-axis'}
)
}
)
])
```
In this example, we create a scatter plot with three data points. We specify the x and y coordinates of the points, as well as the size, color, and symbol of the markers. We also define the layout of the plot, including the title and axis labels.
Once you have defined the layout of your dashboard, you can run the application by calling the `run_server` method:
```python
if __name__ == '__main__':
app.run_server(debug=True)
```
This will start a local web server and open your dashboard in a web browser. You can then interact with the visualizations and explore the data.
Dash also provides a wide range of components and features that allow you to create more complex and interactive dashboards. For example, you can add dropdown menus, sliders, and buttons to control the data displayed in your visualizations. You can also add callbacks to update the visualizations in real-time based on user input.
In conclusion, Dash is a powerful Python framework for building interactive dashboards and data visualizations. With its integration with Plotly, you can create stunning and interactive visualizations with ease. Whether you are a data scientist, a web developer, or a business analyst, Dash provides a flexible and intuitive platform for building web applications that bring your data to life.
1. What is the topic of the teaching material?
The topic is advanced data visualization in Python using Plotly and Dash.
2. What programming language is used in the teaching material?
Python is used in the teaching material.
3. What are the specific libraries used for data visualization?
The specific libraries used for data visualization are Plotly and Dash.
In conclusion, 教學:Python的進階資料視覺化- 使用Plotly與Dash is a tutorial that focuses on advanced data visualization techniques using Plotly and Dash in Python. It provides step-by-step instructions and examples to help users learn how to create interactive and visually appealing plots and dashboards. This tutorial is a valuable resource for individuals looking to enhance their data visualization skills in Python.