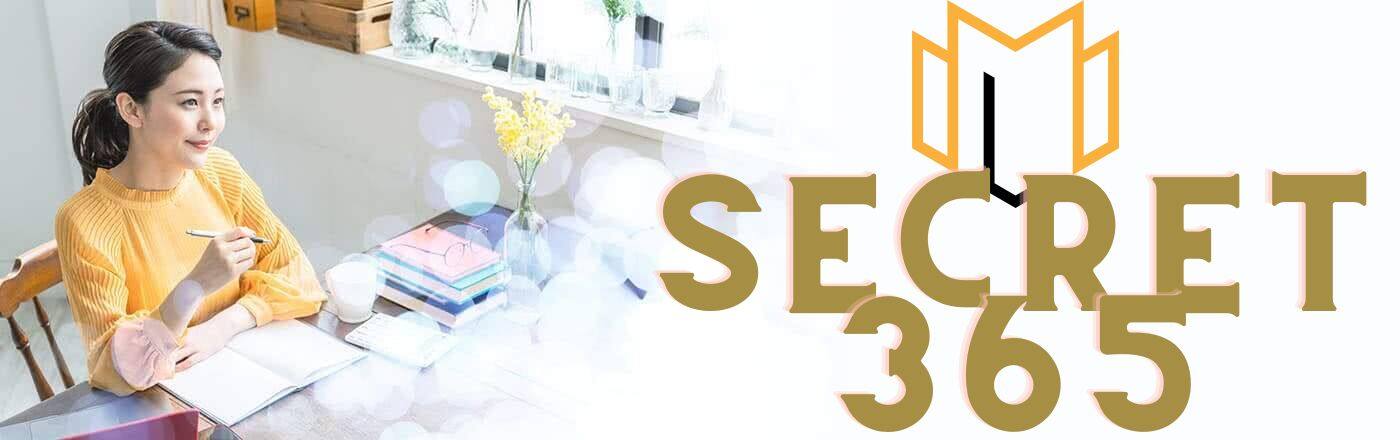
Master data analysis in Python with Pandas Series
A Comprehensive Guide to Data Analysis in Python Using Pandas Series is a comprehensive resource that provides a detailed overview of data analysis techniques using the Pandas Series library in Python. This guide covers various aspects of data analysis, including data manipulation, cleaning, exploration, and visualization. It is designed to help both beginners and experienced Python programmers learn and master the essential skills required for effective data analysis using Pandas Series.
Data analysis is a crucial aspect of any business or research project. It involves examining and interpreting data to uncover patterns, trends, and insights that can inform decision-making. Python is a popular programming language for data analysis due to its simplicity and versatility. One of the most powerful tools for data analysis in Python is the Pandas library, specifically the Pandas Series.
The Pandas Series is a one-dimensional labeled array that can hold any data type. It is similar to a column in a spreadsheet or a SQL table. The Series is built on top of the NumPy array, which makes it fast and efficient for handling large datasets. It also provides a wide range of functions and methods for data manipulation and analysis.
To get started with data analysis using Pandas Series, you first need to install the Pandas library. You can do this by running the command "pip install pandas" in your command prompt or terminal. Once installed, you can import the Pandas library into your Python script using the "import pandas as pd" statement.
Next, you need to create a Pandas Series object. You can do this by passing a list, array, or dictionary to the "pd.Series()" function. For example, if you have a list of numbers, you can create a Series object as follows:
```
import pandas as pd
numbers = [1, 2, 3, 4, 5]
series = pd.Series(numbers)
```
Once you have created a Series object, you can perform various operations on it. For example, you can access individual elements of the Series using indexing. You can also perform mathematical operations on the entire Series, such as addition, subtraction, multiplication, and division. Pandas automatically aligns the elements based on their labels, making it easy to perform element-wise operations.
Another useful feature of Pandas Series is the ability to filter and subset data. You can use conditional statements to select specific elements that meet certain criteria. For example, you can filter a Series to only include values greater than a certain threshold. You can also use logical operators to combine multiple conditions.
In addition to filtering, Pandas Series provides functions for sorting, grouping, and aggregating data. You can sort the Series based on its values or labels using the "sort_values()" and "sort_index()" methods, respectively. You can also group the data based on one or more columns and apply aggregate functions, such as sum, mean, and count, to each group.
Furthermore, Pandas Series supports missing data handling. You can use the "isnull()" and "notnull()" functions to check for missing values in a Series. You can also fill or drop missing values using the "fillna()" and "dropna()" methods, respectively. This is particularly useful when dealing with real-world datasets that often contain missing or incomplete data.
In conclusion, Pandas Series is a powerful tool for data analysis in Python. It provides a convenient and efficient way to manipulate, analyze, and visualize data. Whether you are a beginner or an experienced data analyst, mastering Pandas Series will greatly enhance your data analysis skills. In the next section, we will explore some advanced techniques for data analysis using Pandas Series.
Data analysis is a crucial aspect of any research or business endeavor. It involves examining and interpreting data to uncover patterns, trends, and insights that can inform decision-making. Python, with its powerful libraries and tools, has become a popular choice for data analysis. One such library is Pandas, which provides data structures and functions for efficient data manipulation and analysis. In this article, we will explore various data manipulation techniques using Pandas Series in Python.
Before diving into the specifics, let's understand what a Pandas Series is. A Series is a one-dimensional labeled array that can hold any data type. It is similar to a column in a spreadsheet or a database table. Each element in a Series has a unique label called an index. This index allows for easy and efficient access to the data.
One of the fundamental operations in data analysis is filtering data based on certain conditions. Pandas Series provides a convenient way to filter data using boolean indexing. By applying a condition to a Series, we can create a new Series that contains only the elements that satisfy the condition. This is particularly useful when dealing with large datasets, as it allows us to extract relevant information quickly.
Another common data manipulation technique is sorting. Pandas Series provides a sort_values() function that allows us to sort the elements in a Series in ascending or descending order. We can also specify multiple columns to sort by, which is useful when dealing with complex datasets. Sorting data is essential for tasks such as finding the highest or lowest values, identifying outliers, or arranging data for visualization.
In addition to filtering and sorting, Pandas Series provides various functions for aggregating data. Aggregation involves combining multiple values into a single value based on a specific criterion. For example, we can calculate the sum, mean, median, or standard deviation of a Series using the sum(), mean(), median(), and std() functions, respectively. These functions allow us to gain insights into the overall characteristics of the data.
Data cleaning is another crucial step in data analysis. Pandas Series provides functions for handling missing values, duplicates, and outliers. The dropna() function allows us to remove rows or columns that contain missing values, while the drop_duplicates() function helps us eliminate duplicate values. We can also use the clip() function to limit the values in a Series to a specific range, effectively dealing with outliers.
Pandas Series also supports various mathematical and statistical operations. We can perform arithmetic operations such as addition, subtraction, multiplication, and division on Series, as well as apply mathematical functions like logarithm, exponential, and trigonometric functions. These operations are particularly useful when performing calculations or transformations on the data.
In conclusion, Pandas Series in Python provides a comprehensive set of tools for data manipulation and analysis. From filtering and sorting to aggregating and cleaning, Pandas Series offers a wide range of functions that can help us gain insights from our data. By mastering these techniques, we can effectively analyze and interpret data, enabling us to make informed decisions in various domains, including research, business, and finance. So, if you're looking to enhance your data analysis skills, Pandas Series is definitely worth exploring.
A Comprehensive Guide to Data Analysis in Python Using Pandas Series
Data analysis is a crucial step in any data-driven project. It involves examining, cleaning, transforming, and modeling data to discover useful information and make informed decisions. Python, with its powerful libraries, has become a popular choice for data analysis tasks. One such library is Pandas, which provides high-performance, easy-to-use data structures and data analysis tools.
In this article, we will explore the advanced data analysis and visualization capabilities of Pandas Series in Python. Pandas Series is a one-dimensional labeled array that can hold any data type. It is similar to a column in a spreadsheet or a SQL table. With Pandas Series, you can perform various data analysis tasks efficiently.
To begin with, let's discuss how to create a Pandas Series. You can create a Series from a list, array, or dictionary. For example, you can create a Series from a list of numbers as follows:
```
import pandas as pd
data = [10, 20, 30, 40, 50]
series = pd.Series(data)
```
Once you have created a Series, you can access its elements using indexing and slicing. You can also perform mathematical operations on Series, such as addition, subtraction, multiplication, and division. Pandas Series provides a wide range of built-in functions for statistical analysis, such as mean, median, mode, standard deviation, and correlation.
In addition to basic operations, Pandas Series offers advanced data manipulation techniques. You can filter, sort, and group data based on specific criteria. You can also apply functions to each element of a Series using the `apply()` method. This allows you to perform custom operations on your data.
Another powerful feature of Pandas Series is its ability to handle missing data. You can easily identify and handle missing values using functions like `isnull()` and `fillna()`. Pandas Series also provides methods for removing duplicates and handling outliers.
Data visualization is an essential part of data analysis. Pandas Series integrates well with other Python libraries, such as Matplotlib and Seaborn, to create visually appealing plots and charts. You can create line plots, bar plots, scatter plots, histograms, and more using the `plot()` method of a Series.
Furthermore, Pandas Series supports time series analysis. You can easily manipulate and analyze time-based data using functions like `resample()` and `rolling()`. This makes Pandas Series a valuable tool for analyzing stock prices, weather data, and other time-dependent datasets.
In conclusion, Pandas Series is a powerful tool for data analysis in Python. It provides a wide range of functions and methods for manipulating, analyzing, and visualizing data. Whether you are a beginner or an experienced data analyst, Pandas Series can help you efficiently analyze and interpret your data. So, if you are working on a data-driven project, consider using Pandas Series for your data analysis tasks.
1. What is a Pandas Series?
A Pandas Series is a one-dimensional labeled array that can hold any data type. It is similar to a column in a spreadsheet or a SQL table.
2. What are the key features of Pandas Series?
Some key features of Pandas Series include indexing, alignment, data manipulation, data alignment, and handling missing data.
3. How can Pandas Series be created?
Pandas Series can be created using various methods, such as using a list, NumPy array, dictionary, or scalar value.
In conclusion, "A Comprehensive Guide to Data Analysis in Python Using Pandas Series" provides a detailed and comprehensive overview of using the Pandas Series library for data analysis in Python. The guide covers various aspects of data analysis, including data manipulation, cleaning, exploration, and visualization. It offers step-by-step instructions and examples to help users effectively utilize Pandas Series for their data analysis tasks. Overall, this guide serves as a valuable resource for individuals looking to enhance their data analysis skills using Python and Pandas Series.