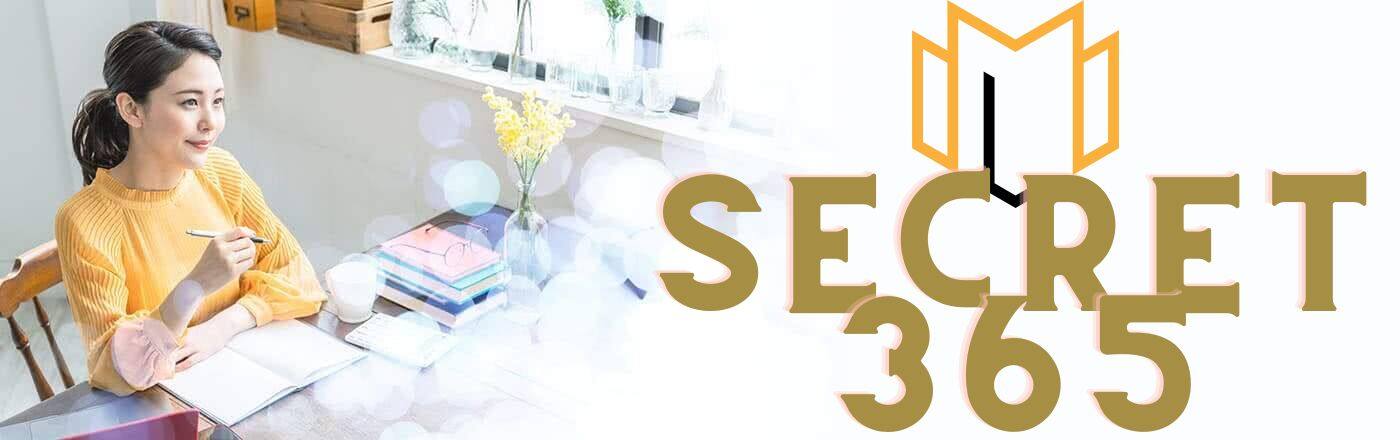
16 Python Data Normalization Methods with Examples - Part 6/6: Master the art of data normalization in Python with these comprehensive methods.
In this final part of the series on Python data normalization methods, we will explore the remaining eight techniques commonly used for data normalization. These methods include feature scaling, robust scaling, quantile transformation, power transformation, Yeo-Johnson transformation, Box-Cox transformation, log transformation, and sigmoid transformation. Each method will be explained in detail, along with code examples to demonstrate their implementation in Python. By understanding these normalization techniques, you will be equipped with a comprehensive toolkit to preprocess and normalize your data effectively for various machine learning tasks.
Data normalization is an essential step in data preprocessing, especially when dealing with machine learning algorithms. It helps to bring all the features of a dataset to a common scale, ensuring that no single feature dominates the others. In this final part of our series on Python data normalization methods, we will explore the Min-Max Scaling technique.
Min-Max Scaling, also known as feature scaling, is a popular method used to normalize data. It transforms the values of a dataset to a fixed range, typically between 0 and 1. This technique is particularly useful when the distribution of the data is unknown or when the data does not follow a Gaussian distribution.
To apply Min-Max Scaling, we need to determine the minimum and maximum values of each feature in the dataset. Once we have these values, we can use the following formula to normalize the data:
[ X_{text{normalized}} = frac{X - X_{text{min}}}{X_{text{max}} - X_{text{min}}} ]
where ( X ) is the original value, ( X_{text{min}} ) is the minimum value of the feature, and ( X_{text{max}} ) is the maximum value of the feature.
Let's consider an example to better understand how Min-Max Scaling works. Suppose we have a dataset with a feature representing the age of individuals. The minimum age in the dataset is 20, and the maximum age is 60. If we want to normalize the age values using Min-Max Scaling, we can apply the formula as follows:
[ X_{text{normalized}} = frac{X - 20}{60 - 20} ]
If an individual's age is 30, the normalized value would be:
[ X_{text{normalized}} = frac{30 - 20}{60 - 20} = frac{10}{40} = 0.25 ]
Similarly, if another individual's age is 50, the normalized value would be:
[ X_{text{normalized}} = frac{50 - 20}{60 - 20} = frac{30}{40} = 0.75 ]
By applying Min-Max Scaling, we have transformed the age values to a common scale between 0 and 1. This normalization technique ensures that the age feature does not dominate other features in the dataset, allowing machine learning algorithms to make fair comparisons.
Python provides several libraries that offer convenient functions to perform Min-Max Scaling. One such library is scikit-learn, a popular machine learning library. The `MinMaxScaler` class in scikit-learn can be used to normalize data using Min-Max Scaling. Here's an example of how to use it:
```python
from sklearn.preprocessing import MinMaxScaler
data = [[30], [50], [40], [45], [55]]
scaler = MinMaxScaler()
normalized_data = scaler.fit_transform(data)
print(normalized_data)
```
In this example, we create a `MinMaxScaler` object and then use its `fit_transform` method to normalize the data. The resulting `normalized_data` will contain the normalized values of the original data.
Min-Max Scaling is a powerful technique for normalizing data, especially when the distribution is unknown or non-Gaussian. It brings all the features of a dataset to a common scale, ensuring that no single feature dominates the others. By using libraries like scikit-learn, implementing Min-Max Scaling in Python becomes a straightforward task.
In conclusion, data normalization is a crucial step in data preprocessing, and Min-Max Scaling is a popular technique for achieving it. By transforming the values of a dataset to a fixed range between 0 and 1, Min-Max Scaling ensures that all features are on an equal footing. With the help of libraries like scikit-learn, implementing Min-Max Scaling in Python is both efficient and effective.
Z-Score Normalization is the final method in our series of 16 Python data normalization techniques. It is a widely used method that transforms data into a standard normal distribution. This technique is particularly useful when dealing with data that has a wide range of values and different scales.
To understand Z-Score Normalization, it is important to first grasp the concept of a z-score. A z-score measures the number of standard deviations a data point is from the mean of a distribution. By applying this concept to our data, we can transform it into a distribution with a mean of 0 and a standard deviation of 1.
The formula for calculating the z-score of a data point is relatively straightforward. We subtract the mean of the data set from the data point and then divide the result by the standard deviation. This process is repeated for each data point in the set, resulting in a normalized distribution.
Let's illustrate this with an example. Suppose we have a dataset of students' test scores, ranging from 60 to 100. We want to normalize this data using the z-score method. First, we calculate the mean and standard deviation of the dataset. Let's say the mean is 80 and the standard deviation is 10.
For a student who scored 70, we subtract the mean (80) from the score (70) and divide the result by the standard deviation (10). The z-score for this student is -1. Similarly, for a student who scored 90, the z-score would be 1. We repeat this process for each student in the dataset, resulting in a new set of scores that are standardized.
Z-Score Normalization has several advantages. Firstly, it allows us to compare data points from different distributions. By transforming the data into a standard normal distribution, we can easily compare values and identify outliers. Secondly, it preserves the shape of the original distribution while standardizing the values. This is particularly useful when working with data that follows a specific distribution pattern.
However, it is important to note that Z-Score Normalization assumes that the data is normally distributed. If the data does not follow a normal distribution, this method may not be appropriate. In such cases, alternative normalization techniques should be considered.
In Python, implementing Z-Score Normalization is relatively simple. The SciPy library provides a function called `zscore` that calculates the z-scores for a given dataset. We can use this function to normalize our data. Additionally, the NumPy library offers a similar function called `standardize` that performs the same task.
To conclude, Z-Score Normalization is a powerful technique for standardizing data and comparing values from different distributions. By transforming the data into a standard normal distribution, we can easily identify outliers and make meaningful comparisons. However, it is important to ensure that the data follows a normal distribution before applying this method. With the help of Python libraries such as SciPy and NumPy, implementing Z-Score Normalization is a straightforward process.
Decimal Scaling
Decimal scaling is a data normalization method that involves shifting the decimal point of a number to the left or right. This technique is useful when dealing with data that has a wide range of values. By scaling the data, we can bring all the values within a specific range, making it easier to compare and analyze.
To apply decimal scaling, we need to determine the maximum absolute value in the dataset. Once we have this value, we calculate the number of decimal places needed to shift the decimal point. This is done by finding the smallest power of 10 that is greater than the maximum absolute value.
For example, let's say we have a dataset with values ranging from -500 to 1000. The maximum absolute value in this case is 1000. To shift the decimal point, we need to find the smallest power of 10 greater than 1000, which is 10000. This means we need to shift the decimal point four places to the left.
To apply decimal scaling, we divide each value in the dataset by the scaling factor, which is 10 raised to the power of the number of decimal places we calculated earlier. In our example, we divide each value by 10000.
Let's consider a practical example to illustrate decimal scaling. Suppose we have a dataset of house prices ranging from $100,000 to $1,000,000. To apply decimal scaling, we need to find the maximum absolute value, which is $1,000,000. The smallest power of 10 greater than $1,000,000 is $10,000,000. Therefore, we need to shift the decimal point six places to the left.
To normalize the data, we divide each house price by $10,000,000. For instance, a house priced at $500,000 would be normalized to 0.05. Similarly, a house priced at $800,000 would be normalized to 0.08.
Decimal scaling is a simple and effective method for normalizing data. It preserves the order of magnitude of the values while bringing them within a specific range. However, it is important to note that decimal scaling does not guarantee that the normalized values will fall within a specific range, such as between 0 and 1. The range of the normalized values depends on the scaling factor, which is determined by the maximum absolute value in the dataset.
One advantage of decimal scaling is that it does not require any prior knowledge about the dataset. It can be applied to any dataset with numerical values, regardless of their distribution or characteristics. Additionally, decimal scaling is a linear transformation, which means it does not distort the relationships between the values in the dataset.
However, decimal scaling may not be suitable for datasets with extreme outliers. Since the scaling factor is determined by the maximum absolute value, outliers can significantly affect the scaling process. In such cases, it may be necessary to consider other normalization methods that are more robust to outliers.
In conclusion, decimal scaling is a data normalization method that involves shifting the decimal point of a number to bring all the values within a specific range. It is a simple and effective technique that can be applied to any dataset with numerical values. However, it is important to consider the characteristics of the dataset and the presence of outliers before applying decimal scaling.
1. What is data normalization in Python?
Data normalization in Python refers to the process of transforming data into a standard format, ensuring that it is consistent and comparable across different variables or datasets.
2. Why is data normalization important in Python?
Data normalization is important in Python as it helps to eliminate inconsistencies and biases in the data, making it easier to analyze and interpret. It also improves the performance of machine learning algorithms by reducing the impact of outliers and improving convergence.
3. What are some examples of data normalization methods in Python?
Some examples of data normalization methods in Python include Min-Max scaling, Z-score normalization, Decimal scaling, Log transformation, and Power transformation. Other methods include Robust scaling, Unit vector scaling, and Quantile transformation.
In conclusion, this article has provided an overview of 16 different data normalization methods in Python. Each method has been explained with examples to demonstrate their usage and benefits. By understanding these normalization techniques, Python developers can effectively preprocess and transform their data to improve the performance and accuracy of their machine learning models.