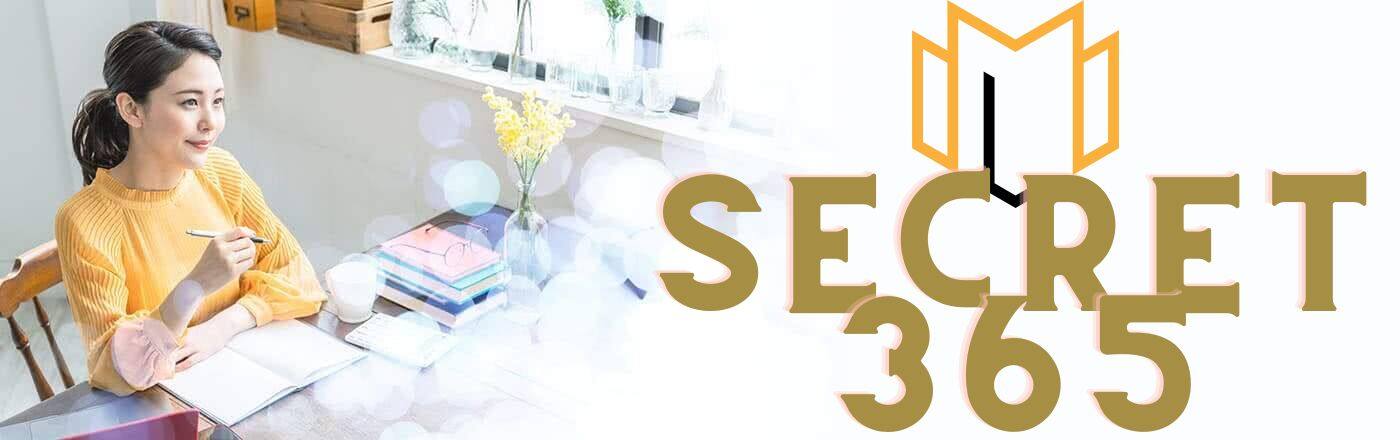
"Master the art of data normalization with Python: Your ultimate guide to achieving clean and consistent data."
Data normalization is a crucial step in data preprocessing that ensures data consistency and improves the accuracy of machine learning models. Python provides various methods for data normalization, allowing users to transform their data into a standardized format suitable for analysis. In this comprehensive guide, we will explore different data normalization methods using Python, accompanied by practical examples. By the end, you will have a solid understanding of how to apply these techniques to your own datasets, enabling you to make informed decisions and derive meaningful insights from your data.
Data Normalization Methods Using Python: A Comprehensive Guide (With Examples)
Introduction to Data Normalization Methods in Python
Data normalization is a crucial step in the data preprocessing phase of any data analysis or machine learning project. It involves transforming the data into a standard format to eliminate redundancy and inconsistencies, making it easier to analyze and interpret. Python, with its extensive libraries and tools, provides several methods for data normalization. In this comprehensive guide, we will explore some of the most commonly used data normalization methods in Python, along with examples to illustrate their implementation.
One of the most widely used data normalization techniques is Min-Max scaling. This method rescales the data to a fixed range, typically between 0 and 1. It works by subtracting the minimum value from each data point and then dividing it by the range of the data. Python's scikit-learn library provides a convenient function called MinMaxScaler that automates this process. Let's consider an example where we have a dataset of house prices ranging from $100,000 to $1,000,000. By applying Min-Max scaling, we can transform these values to a range between 0 and 1, making them more comparable and suitable for analysis.
Another popular data normalization technique is Z-score normalization, also known as standardization. This method transforms the data to have a mean of 0 and a standard deviation of 1. It is particularly useful when dealing with datasets that have outliers or a non-normal distribution. Python's scikit-learn library provides a StandardScaler class that simplifies the implementation of Z-score normalization. Let's consider an example where we have a dataset of students' test scores. By applying Z-score normalization, we can standardize the scores, making them easier to compare and interpret.
In addition to Min-Max scaling and Z-score normalization, there are other data normalization methods that can be useful in specific scenarios. One such method is Decimal Scaling, which involves shifting the decimal point of the data values to achieve normalization. This method is particularly useful when dealing with datasets that have a wide range of values. Python provides various mathematical functions and libraries, such as NumPy, that can be used to implement Decimal Scaling.
Another data normalization method worth mentioning is Log Transformation. This method involves taking the logarithm of the data values, which can help in handling skewed distributions and reducing the impact of outliers. Python's NumPy library provides a log function that can be used to implement Log Transformation. Let's consider an example where we have a dataset of population sizes. By applying Log Transformation, we can reduce the skewness of the data and make it more suitable for analysis.
It is important to note that the choice of data normalization method depends on the specific characteristics of the dataset and the requirements of the analysis or machine learning task. It is recommended to explore and experiment with different normalization methods to determine the most appropriate one for a given scenario. Python's extensive libraries and tools make it easy to implement and compare various data normalization techniques.
In conclusion, data normalization is a crucial step in the data preprocessing phase of any data analysis or machine learning project. Python provides several methods for data normalization, including Min-Max scaling, Z-score normalization, Decimal Scaling, and Log Transformation. Each method has its own advantages and is suitable for different scenarios. By understanding and implementing these data normalization techniques using Python, analysts and data scientists can ensure that their data is in a standardized format, making it easier to analyze and interpret.
Data Normalization Methods Using Python: A Comprehensive Guide (With Examples)
Exploring Different Data Normalization Techniques in Python
Data normalization is a crucial step in the data preprocessing phase of any machine learning project. It involves transforming the data into a standard format to eliminate any biases or inconsistencies that may affect the performance of the model. Python, with its extensive libraries and tools, provides several methods for data normalization. In this article, we will explore some of the most commonly used techniques and provide examples to illustrate their implementation.
One of the simplest and most widely used normalization techniques is Min-Max scaling. This method rescales the data to a specific range, typically between 0 and 1. It is achieved by subtracting the minimum value from each data point and dividing it by the range of the data. Python's scikit-learn library provides a convenient function called MinMaxScaler that automates this process. Let's consider an example where we have a dataset of house prices. By applying Min-Max scaling, we can ensure that all the prices fall within the range of 0 to 1, making it easier for the model to interpret and compare the data.
Another popular normalization technique is Z-score normalization, also known as standardization. This method transforms the data to have a mean of 0 and a standard deviation of 1. It is particularly useful when dealing with data that follows a Gaussian distribution. The scikit-learn library offers a StandardScaler function that simplifies the implementation of Z-score normalization. For instance, if we have a dataset of student exam scores, applying Z-score normalization will allow us to compare the scores across different exams and identify outliers more effectively.
In addition to Min-Max scaling and Z-score normalization, there are other normalization techniques that can be beneficial in specific scenarios. One such technique is Robust Scaling, which is less sensitive to outliers compared to Min-Max scaling and Z-score normalization. It uses the median and interquartile range to rescale the data, making it more robust to extreme values. Python's scikit-learn library provides a RobustScaler function that simplifies the implementation of this technique. For example, if we have a dataset of income levels, Robust Scaling can help us handle outliers and ensure that the data is normalized appropriately.
Furthermore, we have the Log Transformation technique, which is useful when dealing with skewed data. Skewed data can negatively impact the performance of machine learning models, as they often assume a normal distribution. By applying a logarithmic transformation, we can reduce the skewness and make the data more symmetrical. Python's numpy library offers a log function that can be used to implement this technique. For instance, if we have a dataset of population sizes, applying a log transformation can help us normalize the data and improve the model's accuracy.
In conclusion, data normalization is a crucial step in the data preprocessing phase of any machine learning project. Python provides several methods for data normalization, including Min-Max scaling, Z-score normalization, Robust Scaling, and Log Transformation. Each technique has its own advantages and is suitable for different types of data. By understanding and implementing these techniques using Python's libraries, we can ensure that our data is standardized and ready for further analysis and modeling.
Data normalization is a crucial step in the data preprocessing phase of any data analysis or machine learning project. It involves transforming the data into a standardized format, ensuring that it is consistent and comparable across different variables. Python, with its extensive libraries and packages, provides several methods for implementing data normalization. In this article, we will explore some of the most commonly used data normalization methods in Python, along with step-by-step examples.
One of the simplest and most widely used data normalization methods is the Min-Max scaling method. This method rescales the data to a fixed range, typically between 0 and 1. The formula for Min-Max scaling is straightforward: for each data point, subtract the minimum value of the variable and divide it by the range (maximum value minus minimum value). Python provides a convenient way to implement this method using the scikit-learn library. Let's take a look at an example:
```python
from sklearn.preprocessing import MinMaxScaler
import numpy as np
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
scaler = MinMaxScaler()
normalized_data = scaler.fit_transform(data)
print(normalized_data)
```
In this example, we have a 2D array `data` with three variables and three data points. We create an instance of the `MinMaxScaler` class and use its `fit_transform` method to normalize the data. The resulting `normalized_data` is printed, showing the transformed values.
Another commonly used data normalization method is Z-score normalization, also known as standardization. This method transforms the data so that it has a mean of 0 and a standard deviation of 1. The formula for Z-score normalization is straightforward: for each data point, subtract the mean of the variable and divide it by the standard deviation. Python provides a simple way to implement this method using the `StandardScaler` class from the scikit-learn library. Let's see an example:
```python
from sklearn.preprocessing import StandardScaler
import numpy as np
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
scaler = StandardScaler()
normalized_data = scaler.fit_transform(data)
print(normalized_data)
```
In this example, we have the same 2D array `data` as before. We create an instance of the `StandardScaler` class and use its `fit_transform` method to normalize the data. The resulting `normalized_data` is printed, showing the transformed values.
In addition to Min-Max scaling and Z-score normalization, there are other data normalization methods available in Python. These include decimal scaling, logarithmic transformation, and power transformation. Each method has its own advantages and is suitable for different types of data and analysis tasks.
Implementing data normalization methods in Python is relatively straightforward, thanks to the various libraries and packages available. By using the scikit-learn library, we can easily apply Min-Max scaling, Z-score normalization, and other normalization methods to our data. These methods help ensure that our data is in a consistent and comparable format, making it easier to analyze and interpret.
In conclusion, data normalization is an essential step in the data preprocessing phase of any data analysis or machine learning project. Python provides several methods for implementing data normalization, including Min-Max scaling and Z-score normalization. By using the scikit-learn library, we can easily apply these methods to our data. It is important to choose the appropriate normalization method based on the characteristics of the data and the analysis task at hand.
1. What is data normalization?
Data normalization is the process of organizing and transforming data into a consistent and standardized format, eliminating redundancy and improving data integrity.
2. Why is data normalization important?
Data normalization is important because it helps in reducing data redundancy, improving data integrity, and ensuring accurate analysis and comparisons. It also helps in optimizing database performance and simplifying data management.
3. What are some common data normalization methods in Python?
Some common data normalization methods in Python include Min-Max scaling, Z-score normalization, Decimal scaling, and Log transformation. These methods help in scaling and transforming data to a specific range or distribution, based on the requirements of the analysis or model.
In conclusion, the article "Data Normalization Methods Using Python: A Comprehensive Guide (With Examples)" provides a detailed overview of various data normalization techniques in Python. The guide covers essential concepts such as min-max scaling, z-score normalization, and robust scaling, along with their implementation using Python libraries like NumPy and scikit-learn. The article also includes practical examples to illustrate the application of these methods in real-world scenarios. Overall, this comprehensive guide serves as a valuable resource for individuals looking to understand and implement data normalization techniques in Python.