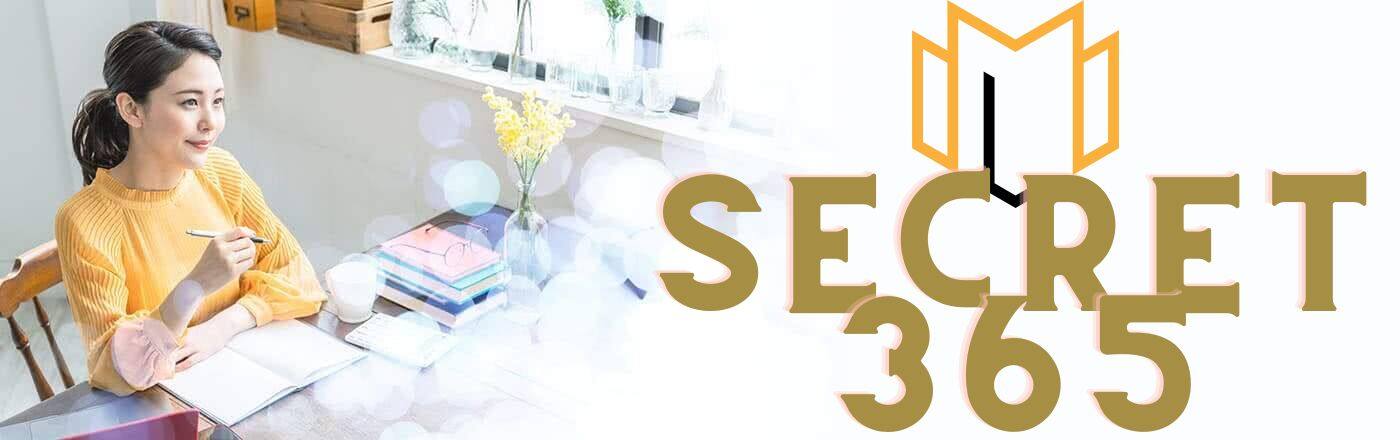
Master Laravel Eloquent: Unlock the Power of Efficient Database Operations.
Mastering Laravel Eloquent: Six Essential Things to Know is a comprehensive guide that aims to provide developers with a deep understanding of Laravel's Eloquent ORM (Object-Relational Mapping). This book covers six essential aspects of Eloquent, including querying the database, defining relationships between models, working with eager loading and lazy loading, utilizing advanced features like scopes and mutators, and optimizing performance. Whether you are a beginner or an experienced Laravel developer, this book will equip you with the knowledge and skills needed to effectively leverage Eloquent in your Laravel projects.
Laravel Eloquent is a powerful and intuitive ORM (Object-Relational Mapping) tool that allows developers to interact with databases using PHP code. It simplifies the process of working with databases by providing a fluent and expressive syntax. In this article, we will explore six essential things to know about Laravel Eloquent, focusing on understanding the basics.
First and foremost, it is important to understand that Eloquent is an integral part of the Laravel framework. It provides a convenient way to interact with databases, making it easier to perform common database operations such as retrieving, inserting, updating, and deleting records. Eloquent follows the active record pattern, which means that each database table has a corresponding "model" class that represents it.
To get started with Eloquent, you need to define a model class for each table in your database. These model classes extend the base Eloquent model class provided by Laravel. By convention, the model class name should be singular and capitalized, while the corresponding table name should be plural and lowercase. This naming convention allows Eloquent to automatically map between the model and the table.
Once you have defined your model classes, you can start using Eloquent to perform database operations. One of the most common operations is retrieving records from the database. Eloquent provides a fluent query builder interface that allows you to build complex queries using a chainable syntax. You can use methods like `where`, `orderBy`, and `limit` to filter, sort, and limit the results.
In addition to querying records, Eloquent also provides convenient methods for creating, updating, and deleting records. To create a new record, you can simply create a new instance of the model class and set its attributes. Then, you can call the `save` method to persist the record to the database. To update an existing record, you can retrieve it from the database, modify its attributes, and call the `save` method again. To delete a record, you can call the `delete` method on the model instance.
Eloquent also supports relationships between tables, allowing you to define and work with complex data structures. There are four types of relationships supported by Eloquent: one-to-one, one-to-many, many-to-one, and many-to-many. These relationships are defined using methods like `hasOne`, `hasMany`, `belongsTo`, and `belongsToMany`. Once the relationships are defined, you can use them to retrieve related records and perform operations on them.
Another important feature of Eloquent is its support for eager loading. When retrieving records, Eloquent automatically fetches their related records, reducing the number of database queries and improving performance. You can specify which relationships to eager load using the `with` method. This is especially useful when working with large datasets or when you need to minimize the number of database queries.
In conclusion, mastering Laravel Eloquent is essential for any Laravel developer. Understanding the basics of Eloquent, such as defining model classes, querying records, performing CRUD operations, working with relationships, and using eager loading, will greatly enhance your ability to work with databases in Laravel. Eloquent's expressive syntax and powerful features make it a valuable tool for building robust and efficient applications.
Mastering Laravel Eloquent: Six Essential Things to Know
Laravel Eloquent is a powerful ORM (Object-Relational Mapping) tool that simplifies database operations in Laravel applications. It provides an intuitive and expressive syntax for querying and manipulating database records. While Eloquent is easy to get started with, mastering its advanced techniques can greatly optimize your Laravel Eloquent queries. In this article, we will explore six essential things to know to take your Eloquent skills to the next level.
Firstly, eager loading is a technique that allows you to load relationships along with the main model. By default, Eloquent uses lazy loading, which means it loads relationships only when they are accessed. However, this can result in the N+1 problem, where multiple queries are executed to fetch related records. Eager loading solves this issue by fetching all related records in a single query. To eager load relationships, you can use the `with` method. This can significantly improve the performance of your queries, especially when dealing with large datasets.
Secondly, understanding the difference between `where` and `orWhere` clauses is crucial for constructing complex queries. The `where` clause adds a condition to the query, while the `orWhere` clause adds an alternative condition. By combining these clauses with logical operators such as `and` and `or`, you can create intricate query conditions. This allows you to filter records based on multiple criteria, making your queries more flexible and powerful.
Next, utilizing query scopes can enhance the reusability and readability of your code. A query scope is a method defined on an Eloquent model that encapsulates a specific query condition. By defining query scopes, you can easily apply common conditions to your queries without repeating code. For example, you can create a scope to retrieve only active users or filter records based on a specific date range. Query scopes make your code more maintainable and help you write cleaner and more concise queries.
Fourthly, leveraging the power of subqueries can greatly optimize complex queries. A subquery is a query nested within another query. It allows you to perform calculations or retrieve data from related tables without the need for multiple queries. Eloquent provides a fluent interface for constructing subqueries using the `selectSub` and `fromSub` methods. By using subqueries, you can avoid unnecessary joins and improve the performance of your queries.
Fifthly, taking advantage of the `pluck` method can simplify your code when you only need a single column from a query result. The `pluck` method retrieves a single column's value from the first result of a query. This is particularly useful when you need to retrieve a list of IDs or names from a table. Instead of fetching the entire record and extracting the desired column manually, you can use the `pluck` method to retrieve the column directly. This reduces the amount of data transferred from the database and improves the efficiency of your queries.
Lastly, understanding the difference between `find` and `findOrFail` methods is crucial for error handling. The `find` method retrieves a record by its primary key, returning `null` if the record is not found. On the other hand, the `findOrFail` method throws an exception if the record is not found. By using `findOrFail`, you can handle missing records gracefully and provide appropriate error messages to users. This ensures that your application remains robust and prevents unexpected errors from occurring.
In conclusion, mastering Laravel Eloquent requires a deep understanding of its advanced techniques. By utilizing eager loading, constructing complex queries with `where` and `orWhere` clauses, defining query scopes, leveraging subqueries, using the `pluck` method, and handling missing records with `findOrFail`, you can optimize your Laravel Eloquent queries and improve the performance and maintainability of your applications. With these essential skills in your toolkit, you'll be well-equipped to tackle complex database operations in your Laravel projects.
Laravel Eloquent is a powerful and intuitive ORM (Object-Relational Mapping) tool that allows developers to interact with databases using PHP syntax. It simplifies the process of working with databases by providing a fluent and expressive API. However, to truly master Laravel Eloquent, there are six essential things that every developer should know.
First and foremost, understanding the relationship types in Laravel Eloquent is crucial. Eloquent provides several types of relationships, including one-to-one, one-to-many, many-to-many, and polymorphic relationships. Each type has its own use case and can greatly simplify database interactions. By understanding these relationship types and how to define them in your models, you can build efficient and scalable applications.
Next, it is important to know how to use eager loading in Laravel Eloquent. Eager loading allows you to load relationships along with the main model, reducing the number of database queries and improving performance. By using the `with` method, you can specify which relationships to load, resulting in a more efficient and optimized application.
Another essential aspect of Laravel Eloquent is the use of accessors and mutators. Accessors allow you to define custom attributes on your models, while mutators allow you to modify attribute values before they are saved to the database. By using accessors and mutators, you can manipulate data in a consistent and controlled manner, ensuring data integrity and flexibility.
Furthermore, taking advantage of query scopes in Laravel Eloquent can greatly enhance your development experience. Query scopes allow you to define reusable query constraints on your models, making it easier to write complex queries. By encapsulating common query logic into scopes, you can improve code readability and maintainability.
In addition, understanding how to use the `firstOrCreate` and `updateOrCreate` methods in Laravel Eloquent is essential for efficient database operations. These methods allow you to find a record based on certain attributes and create or update it if it doesn't exist. By using these methods, you can simplify your code and avoid unnecessary database queries.
Lastly, knowing how to use events and observers in Laravel Eloquent can greatly extend the functionality of your models. Events allow you to hook into various model lifecycle events, such as creating, updating, and deleting, and perform additional actions. Observers, on the other hand, allow you to separate the logic related to model events from the models themselves, resulting in cleaner and more maintainable code.
In conclusion, mastering Laravel Eloquent requires a deep understanding of its essential features and best practices. By understanding relationship types, eager loading, accessors and mutators, query scopes, `firstOrCreate` and `updateOrCreate` methods, as well as events and observers, you can build efficient and scalable Laravel Eloquent models. These six essential things will not only improve your development experience but also help you create robust and maintainable applications. So, take the time to learn and practice these concepts, and you'll be well on your way to becoming a Laravel Eloquent master.
1. What is Laravel Eloquent?
Laravel Eloquent is an ORM (Object-Relational Mapping) that allows developers to interact with databases using object-oriented syntax in the Laravel PHP framework.
2. What are the six essential things to know about mastering Laravel Eloquent?
The six essential things to know about mastering Laravel Eloquent are: understanding models and relationships, using query scopes, utilizing eager loading, leveraging accessors and mutators, implementing event listeners, and optimizing database queries.
3. Why is mastering Laravel Eloquent important?
Mastering Laravel Eloquent is important because it allows developers to efficiently and effectively work with databases in their Laravel applications. It provides a powerful and intuitive way to interact with data, simplifying database operations and improving code readability and maintainability.
In conclusion, mastering Laravel Eloquent requires understanding six essential things: understanding the Eloquent ORM, defining relationships between models, utilizing query scopes, using eager loading to optimize performance, leveraging model events for additional functionality, and utilizing advanced features like polymorphic relationships and query builders. By gaining proficiency in these areas, developers can effectively utilize Laravel Eloquent to build robust and efficient applications.